How to Check if Input Is Integer in Java
-
Check if Input Is Integer Using the
hasNextInt
Method in Java -
Check if the Number Is an Integer Using the
try...catch
Block - Check if the Number Is an Integer Using Regular Expressions
-
Check if the Number Is an Integer Using
Pattern
andMatcher
Classes - Check if the Number Is an Integer Using Apache Commons Lang
- Conclusion
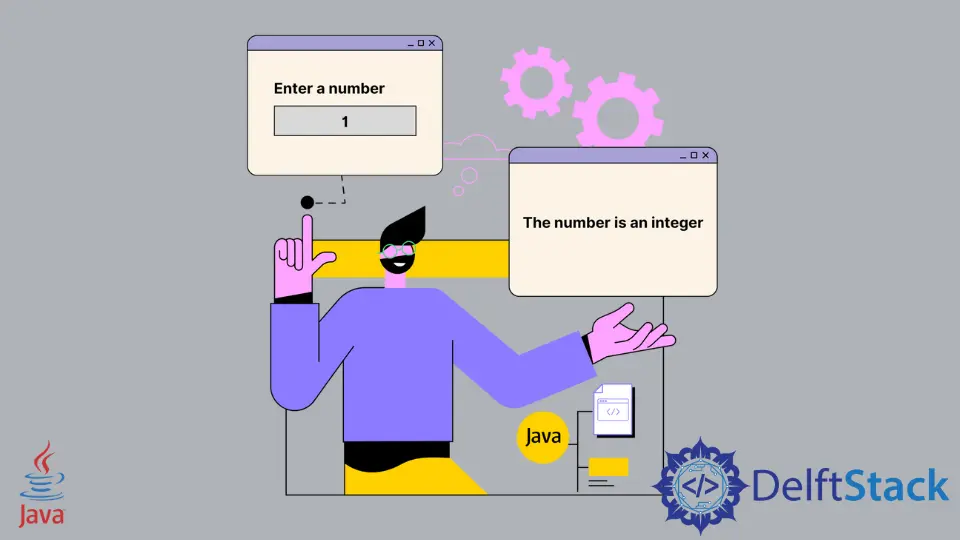
Java offers several methods and techniques to check whether user-provided input is an integer. This is a common requirement in applications that involve user interaction.
We will explore four distinct approaches to achieving this goal. By the end of this article, you will have a comprehensive understanding of how to verify if user input is an integer using different Java techniques.
Check if Input Is Integer Using the hasNextInt
Method in Java
The Scanner
class is an essential utility in Java that allows for tokenization and parsing of input. It can break the input into tokens based on specified delimiters, making it easier to handle various types of input.
The Scanner
class offers a convenient method named hasNextInt()
that allows you to check whether the next token in the input stream is an integer. It returns true
if the next token is an integer and false
otherwise.
You must remember that if the scanner object is closed when attempting to use hasNextInt()
, it throws an IllegalStateException
.
Here’s a simple Java program that utilizes the Scanner
class and the hasNextInt()
method to check if the input provided by the user is an integer:
import java.util.Scanner;
public class CheckIntegerInput {
public static void main(String[] args) {
System.out.print("Enter the number: ");
Scanner scanner = new Scanner(System.in);
if (scanner.hasNextInt()) {
System.out.println("The number is an integer");
} else {
System.out.println("The number is not an integer");
}
}
}
Here, the program begins by prompting the user to enter a number with the message Enter the number:
. This ensures that the user knows the expected input format.
A Scanner
object named scanner
is created to read input from the standard input stream (System.in
). This object will be used to check if the input is an integer.
The heart of the code lies in the conditional statement:
if (scanner.hasNextInt()) {
System.out.println("The number is an integer");
} else {
System.out.println("The number is not an integer");
}
The hasNextInt()
method checks if the next token in the input stream can be evaluated as an integer. If it can, the program prints The number is an integer
; otherwise, it prints The number is not an integer.
To illustrate the code’s behavior, consider the following examples.
For valid integer input:
Enter the number: 1
The number is an integer
In this case, the user entered the integer 1
, and the program correctly identified it as an integer.
For non-integer input:
Enter the number: Hi
The number is not an integer
Here, the user input is Hi
, which is not a valid integer. The program appropriately identifies it as such.
Check if the Number Is an Integer Using the try...catch
Block
Determining whether a given input is an integer can also be efficiently achieved using the try...catch
block and the Integer.parseInt()
method.
The try...catch
block in Java is a control flow mechanism that allows for the handling of exceptions. It works as follows:
- The code that might throw an exception is placed within the
try
block. - If an exception is thrown during the execution of the
try
block, the correspondingcatch
block is executed, providing an opportunity to handle the exception gracefully.
On the other hand, the Integer.parseInt()
method in Java is used to convert a string to its integer representation. It throws a NumberFormatException
if the string cannot be converted to an integer.
This method is fundamental for validating whether a given input is an integer.
Here’s a simple Java program that utilizes the try...catch
block and the Integer.parseInt()
method to check if the input provided by the user is an integer:
import java.util.Scanner;
public class CheckIntegerInput {
public static void main(String[] args) {
System.out.print("Enter the number: ");
Scanner scanner = new Scanner(System.in);
try {
Integer.parseInt(scanner.next());
System.out.println("The number is an integer");
} catch (NumberFormatException ex) {
System.out.println("The number is not an integer");
}
}
}
In this code, we use the Scanner
class to take user input from the console. Then, the Integer.parseInt()
method attempts to parse the input as an integer inside the try
block.
If parsing succeeds, it prints The number is an integer
. However, if an exception (specifically NumberFormatException
) is caught, it prints The number is not an integer
.
Let’s see how the program behaves with different inputs.
For valid integer input:
Enter the number: 42
The number is an integer
For non-integer input:
Enter the number: abc
The number is not an integer
As we can see, the output of the above code is similar to the one in the first example code given above.
Check if the Number Is an Integer Using Regular Expressions
Another effective approach to validate whether a given input is an integer is by using regular expressions.
Regular expressions are patterns that define a set of strings. They are widely used for pattern matching within strings, allowing us to search, match, and extract information based on a specified pattern.
In our case, we’ll define a regular expression pattern that represents an integer. The pattern will match strings that consist of one or more digits.
We can use the matches()
method provided by the String
class in Java, which allows us to check if a string matches a given regular expression.
Let’s have an example that uses regular expressions to check if the input provided by the user is an integer:
import java.util.Scanner;
public class IntegerInputValidator {
public static boolean isInteger(String input) {
String integerPattern = "^-?\\d+$";
return input.matches(integerPattern);
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
String userInput = scanner.nextLine();
boolean isInputInteger = isInteger(userInput);
if (isInputInteger) {
System.out.println("The input is an integer.");
} else {
System.out.println("The input is not an integer.");
}
}
}
As we can see, this program defines an isInteger
method that utilizes a regular expression to validate whether a given string represents an integer.
Here:
^
: Asserts the start of the string.-?
: Allows for an optional minus sign.\\d+
: Matches one or more digits.$
: Asserts the end of the string.
Within the main
method, the program prompts the user to input a number, which is obtained using the Scanner
. The obtained input is then passed to the isInteger
method for validation.
Depending on whether the input matches the integer pattern, the program outputs whether it is an integer or not.
Let’s observe how the program behaves with different inputs:
For valid integer input:
Enter a number: 34
The input is an integer.
For non-integer input:
Enter a number: abc
The input is not an integer.
This is the same output as the one in the previous example codes.
Check if the Number Is an Integer Using Pattern
and Matcher
Classes
Validating whether a given input is an integer can also be accomplished by using the Pattern
and Matcher
classes from the java.util.regex
package.
The Pattern
class in Java is used to represent a compiled regular expression. It provides methods to work with the compiled regular expression pattern, allowing for efficient pattern matching and searching within strings.
public static Pattern compile(String regex)
The compile
method of the Pattern
class compiles the given regular expression String regex
into a Pattern
object. The compiled Pattern
can then be used to create a Matcher
for pattern matching operations.
Now, the Matcher
class in Java is used to perform matching operations on a character sequence (typically a string) against a compiled regular expression Pattern
. It provides methods to match the pattern against the input string and extract matched substrings.
public boolean matches()
The matches
method of the Matcher
class attempts to match the entire input sequence against the specified pattern. This method returns true
if the entire input sequence matches the pattern and false
otherwise.
Take a look at an example that uses the Pattern
and Matcher
classes to check if the input provided by the user is an integer:
import java.util.Scanner;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class IntegerInputValidator {
public static boolean isInteger(String input) {
String integerPattern = "^-?\\d+$";
Pattern pattern = Pattern.compile(integerPattern);
Matcher matcher = pattern.matcher(input);
return matcher.matches();
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
String userInput = scanner.nextLine();
boolean isInputInteger = isInteger(userInput);
if (isInputInteger) {
System.out.println("The input is an integer.");
} else {
System.out.println("The input is not an integer.");
}
}
}
In this example, we define a regular expression pattern, integerPattern
, that matches integers. The pattern ^-?\\d+$
allows for an optional minus sign (-
) followed by one or more digits (\\d+
).
We then compile the pattern using Pattern.compile()
before we create a Matcher
object using the compiled pattern and the input string.
We use matcher.matches()
to check if the input matches the defined pattern, determining if it’s an integer.
When you run the program, it prompts the user to enter a number. After the user provides the input, the program validates whether it’s an integer using the isInteger
method.
Depending on the result, it displays whether the input is an integer or not.
Let’s observe how the program behaves with different inputs:
For valid integer input:
Enter a number: 12345
The input is an integer.
Enter a number: -6789
The input is an integer.
Enter a number: 0
The input is an integer.
For non-integer input:
Enter a number: abc123
The input is not an integer.
Check if the Number Is an Integer Using Apache Commons Lang
One of the functionalities Apache Commons Lang offers is a method to determine if a string is a valid integer. It provides a class called NumberUtils
, which contains various utility methods for working with numbers in Java.
The NumberUtils
class includes methods to check if a string is a valid number or integer, the NumberUtils.isDigits()
method. It allows us to check if the input string consists of digits only, indicating it could be a valid integer.
First, make sure you have Apache Commons Lang library added to your project. You can download it from the Apache Commons Lang website.
Next, include the JAR file in your project.
Take a look at a simple example that uses the NumberUtils.isDigits()
method to check if the input provided by the user is an integer:
import java.util.Scanner;
import org.apache.commons.lang3.math.NumberUtils;
public class IntegerInputValidator {
public static boolean isInteger(String input) {
return NumberUtils.isDigits(input);
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
String userInput = scanner.nextLine();
boolean isInputInteger = isInteger(userInput);
if (isInputInteger) {
System.out.println("The input is an integer.");
} else {
System.out.println("The input is not an integer.");
}
}
}
In this example, we use the NumberUtils.isDigits()
method from Apache Commons Lang to check if the input consists of digits only, indicating a potential integer.
If the input string contains only digits, the method returns true
, suggesting that the input could be a valid integer.
Let’s observe how the program behaves with different inputs:
For valid integer input:
Enter a number: 12345
The input is an integer.
Enter a number: -6789
The input is not an integer.
For non-integer input:
Enter a number: abc123
The input is not an integer.
Conclusion
In this article, we have explored multiple methods and techniques to check whether user-provided input is an integer in Java. The choice of method largely depends on the complexity of your application and specific use cases.
The try...catch
block with Integer.parseInt()
is useful when you need to handle non-integer input gracefully, while regular expressions offer powerful pattern-matching capabilities. On the other hand, the NumberUtils.isDigits()
method from Apache Commons Lang simplifies the process in projects where you already use this library.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn