How to Calculate Average in Java
- Method 1: Using a Simple Loop
- Method 2: Using Streams
- Method 3: Using a Method to Calculate Average
- Conclusion
- FAQ
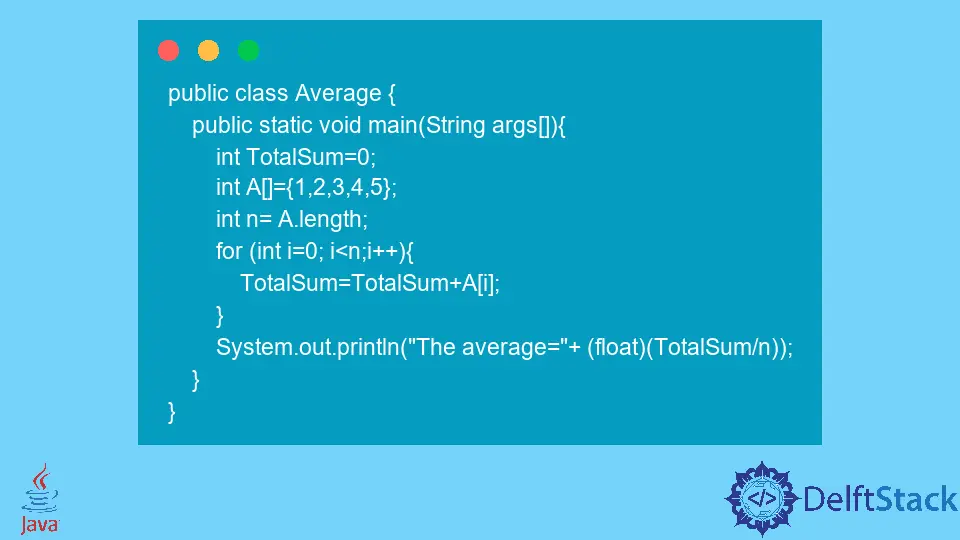
Calculating the average in Java is a fundamental skill that every programmer should master. Whether you’re working with a small set of numbers or handling large datasets, knowing how to compute the average can help you analyze data effectively.
In this tutorial, we will explore various methods to calculate the average in Java, providing you with clear examples and explanations. By the end of this article, you’ll not only understand how to calculate averages but also gain insights into best practices and potential pitfalls. So, let’s dive in and learn how to calculate averages in Java seamlessly!
Method 1: Using a Simple Loop
One of the simplest ways to calculate the average in Java is by using a for
loop. This method involves summing all the numbers in an array and then dividing the total by the number of elements. Here’s how you can do it:
public class AverageCalculator {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
int sum = 0;
for (int number : numbers) {
sum += number;
}
double average = (double) sum / numbers.length;
System.out.println("The average is: " + average);
}
}
Output:
The average is: 30.0
In this code, we first declare an array of integers named numbers
. We then create a variable sum
to hold the total of all numbers. The for-each loop iterates through each number in the array, adding it to sum
. After the loop, we calculate the average by dividing sum
by the length of the array. Finally, we print the average to the console. This method is straightforward and efficient for calculating averages in smaller datasets.
Method 2: Using Streams
Java 8 introduced the Stream API, which provides a more functional approach to handling collections of data. Using streams, you can calculate the average in a more concise and readable manner. Here’s an example of how to do it:
import java.util.Arrays;
public class AverageCalculator {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
double average = Arrays.stream(numbers)
.average()
.orElse(0.0);
System.out.println("The average is: " + average);
}
}
Output:
The average is: 30.0
In this example, we utilize the Arrays.stream()
method to create a stream from the numbers
array. The average()
method computes the average of the elements in the stream. We also use orElse(0.0)
to handle the case where the array might be empty, ensuring that the average defaults to 0.0
in such instances. This method is elegant and leverages the power of Java’s functional programming capabilities, making it a great choice for modern Java applications.
Method 3: Using a Method to Calculate Average
Another effective way to calculate the average in Java is by encapsulating the logic within a separate method. This approach enhances code reusability and organization. Here’s how you can implement it:
public class AverageCalculator {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
double average = calculateAverage(numbers);
System.out.println("The average is: " + average);
}
public static double calculateAverage(int[] numbers) {
int sum = 0;
for (int number : numbers) {
sum += number;
}
return (double) sum / numbers.length;
}
}
Output:
The average is: 30.0
In this code, we define a method called calculateAverage
that takes an array of integers as a parameter. Inside the method, we sum the numbers using a for-each loop, similar to our first example. After calculating the total, we return the average. In the main
method, we call calculateAverage
and print the result. This method promotes better organization and allows you to reuse the average calculation logic across different parts of your application.
Conclusion
Calculating the average in Java is a straightforward process that can be accomplished through various methods, including using loops, streams, and encapsulating logic in separate methods. Each approach has its own advantages, and the choice depends on your specific requirements and coding style. By mastering these techniques, you can effectively analyze data and enhance your programming skills. Whether you’re a beginner or an experienced developer, understanding how to calculate averages will serve you well in your Java programming journey.
FAQ
-
How do I handle an empty array when calculating the average?
You can use theorElse()
method when using streams, or check if the array length is zero before performing the calculation to avoid division by zero errors. -
Can I calculate the average of floating-point numbers in Java?
Yes, you can use adouble
array instead of anint
array to calculate the average of floating-point numbers. -
What is the difference between using a loop and streams for calculating averages?
Using a loop is more traditional and straightforward, while streams offer a more concise and functional approach, which can improve code readability. -
Is there a built-in method in Java to calculate the average?
Java does not have a built-in method specifically for calculating averages, but you can use theStream
API for a streamlined approach. -
How can I calculate the average of a list instead of an array?
You can convert the list to a stream usinglist.stream()
and then apply theaverage()
method just like with an array.