The %= Operator in Java
- Understanding the %= Operator
- Example Usage of the %= Operator
- Benefits of Using the %= Operator
- Common Mistakes to Avoid
- Conclusion
- FAQ
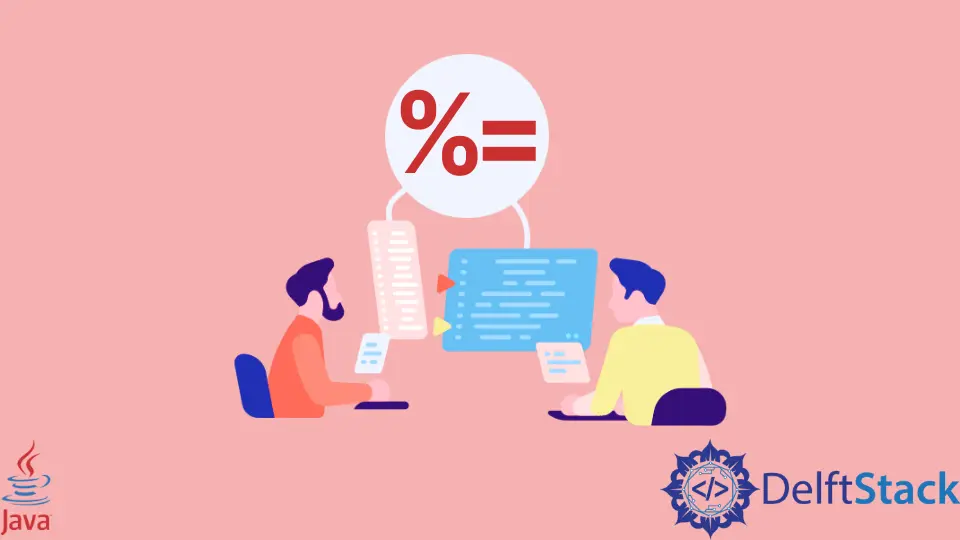
Java is a versatile programming language that offers various operators to perform arithmetic and logical operations. One such operator is the %= operator, which is often overlooked by beginners.
This article introduces the use of the %= operator in Java, explaining how it works and providing practical examples. By the end of this article, you will have a solid understanding of how to use this operator effectively in your Java programs. Whether you’re a novice or an experienced developer, grasping the nuances of the %= operator can enhance your coding efficiency and performance.
Understanding the %= Operator
The %= operator in Java is a compound assignment operator that combines the modulus operation with assignment. It calculates the remainder when one number is divided by another and assigns the result to the variable. The syntax for using the %= operator is straightforward:
variable %= value;
This is equivalent to writing:
variable = variable % value;
For instance, if you have a variable a
with a value of 10 and you perform the operation a %= 3
, the new value of a
will be the remainder of 10 divided by 3, which is 1.
Using the %= operator can make your code cleaner and more concise. It reduces redundancy by allowing you to perform the modulus operation and assignment in one step. This can be particularly useful in loops or when working with arrays, where you might frequently need to calculate remainders.
Example Usage of the %= Operator
Let’s take a practical example to illustrate how the %= operator works in Java. Suppose you want to determine how many items can fit into boxes of a certain size. Here’s a simple Java program that demonstrates this concept.
public class ModulusExample {
public static void main(String[] args) {
int totalItems = 25;
int boxSize = 6;
totalItems %= boxSize;
System.out.println("Remaining items after filling boxes: " + totalItems);
}
}
Output:
Remaining items after filling boxes: 1
In this example, we start with 25 items and a box size of 6. The %= operator calculates how many items remain after completely filling boxes. After executing the operation, the output shows that there is 1 item left. This simple example highlights how the %= operator can be applied in real-world scenarios.
Benefits of Using the %= Operator
Using the %= operator in Java offers several benefits. First and foremost, it enhances code readability. Instead of writing longer expressions, you can condense your code, making it easier to understand at a glance. This is especially beneficial in larger programs where clarity is key.
Moreover, the %= operator can help reduce the likelihood of errors. When you use compound assignment operators, you minimize the chances of mistakenly altering the original variable before performing the operation. This can lead to fewer bugs and more reliable code.
Additionally, using the %= operator can improve performance. Although the difference may be negligible in small programs, in larger applications or loops, reducing the number of operations can lead to better efficiency. This can be crucial in performance-sensitive applications where every millisecond counts.
Common Mistakes to Avoid
While using the %= operator is straightforward, there are a few common pitfalls that developers should watch out for. One mistake is assuming that the operator works with non-integer types. The %= operator is primarily designed for integer-based calculations. If you try to use it with floating-point numbers without proper casting, you might encounter unexpected results.
Another mistake is neglecting to initialize the variable before using the %= operator. If the variable has not been assigned a value, you will run into errors. Always ensure that the variable you are working with has a valid initial value.
Lastly, be cautious with the order of operations in expressions. The %= operator has a specific precedence, and mixing it with other operators can lead to confusion. Always use parentheses to clarify your intentions when combining multiple operations.
Conclusion
The %= operator in Java is a powerful tool that simplifies the process of calculating remainders and assigning values. By understanding how to use this operator effectively, you can write cleaner, more efficient code. Whether you’re working on simple calculations or complex algorithms, the %= operator can save you time and reduce errors. Embrace this operator in your Java programming journey, and watch your coding skills grow.
FAQ
-
What does the %= operator do in Java?
The %= operator calculates the remainder of a division operation and assigns it to a variable. -
Can I use the %= operator with floating-point numbers?
The %= operator is primarily designed for integer calculations. For floating-point numbers, ensure proper casting. -
What are some common mistakes when using the %= operator?
Common mistakes include not initializing the variable and misunderstanding the order of operations. -
How does the %= operator improve code readability?
It condenses longer expressions into a single line, making the code easier to understand. -
Is the %= operator efficient in terms of performance?
Yes, using compound assignment operators like %= can improve performance by reducing the number of operations.