How to Convert Time to String in Go
- Using the Time.Format Method
- Parsing Time from String
- Formatting Time with Custom Layouts
- Handling Time Zones
- Conclusion
- FAQ
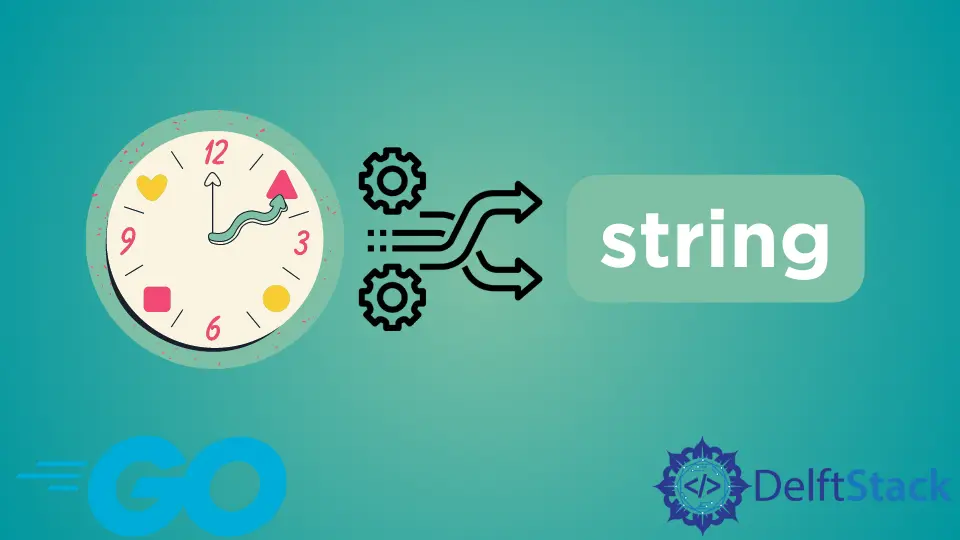
Converting time to a string in Go can be a crucial task for developers, especially when formatting timestamps for logs, user interfaces, or data storage. Understanding how to manipulate time effectively allows you to present data in a human-readable format, making your applications more user-friendly.
In this tutorial, we will explore various methods to convert time to string in Go, complete with code examples and detailed explanations. Whether you are a beginner or an experienced Go developer, this guide will provide you with the insights you need to master time formatting in your applications.
Using the Time.Format Method
One of the most straightforward ways to convert time to a string in Go is by utilizing the Time.Format
method. This method allows you to specify a layout that defines how the time should be represented as a string. The layout uses a reference time, which is the specific date and time of “Mon Jan 2 15:04:05 MST 2006”.
Here’s a simple example:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
formattedTime := currentTime.Format("2006-01-02 15:04:05")
fmt.Println(formattedTime)
}
Output:
2023-10-15 14:30:45
In this example, we first import the necessary packages: fmt
for printing output and time
for handling time-related functions. We then capture the current time using time.Now()
. The Format
method is called on the currentTime
variable, passing the desired layout as a string. The output will display the current date and time in a formatted string. This method is flexible, allowing you to customize the output to suit your needs, whether you prefer a simple date or a detailed timestamp.
Parsing Time from String
In addition to converting time to a string, Go also allows you to parse a string back into a time object. This can be useful when you receive date and time data in string format and need to manipulate it as a time object. The time.Parse
function is used for this purpose.
Here’s how you can do it:
package main
import (
"fmt"
"time"
)
func main() {
dateString := "2023-10-15 14:30:45"
layout := "2006-01-02 15:04:05"
parsedTime, err := time.Parse(layout, dateString)
if err != nil {
fmt.Println("Error parsing date:", err)
return
}
fmt.Println("Parsed time:", parsedTime)
}
Output:
Parsed time: 2023-10-15 14:30:45 +0000 UTC
In this example, we define a string representing a date and time. The time.Parse
function takes two arguments: the layout and the string to be parsed. If the parsing is successful, it returns a Time
object. If there’s an error, we handle it gracefully by printing an error message. The output shows the parsed time, which can now be used for further manipulation or formatting.
Formatting Time with Custom Layouts
One of the powerful features of Go’s time
package is the ability to create custom layouts for formatting time. This flexibility allows you to present time in various ways, depending on your application’s requirements. You can include components like the month, day, year, hour, minute, and second, and arrange them in any order.
Here’s an example of formatting time with a custom layout:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
customLayout := "02-Jan-2006 03:04 PM"
formattedTime := currentTime.Format(customLayout)
fmt.Println(formattedTime)
}
Output:
15-Oct-2023 02:30 PM
In this code snippet, we use a custom layout that formats the date as “day-month-year hour:minute AM/PM”. The Format
method applies this layout to the current time, producing a string that is easy to read and understand. This method is particularly useful for applications that require specific date formats, such as reporting tools or user-facing interfaces.
Handling Time Zones
When working with time in Go, it’s essential to consider time zones, especially if your application will be used across different geographical locations. The time
package in Go provides robust support for time zones, allowing you to convert and format time according to specific zones.
Here’s an example of converting time to a string while considering a specific time zone:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
loc, err := time.LoadLocation("America/New_York")
if err != nil {
fmt.Println("Error loading location:", err)
return
}
newYorkTime := currentTime.In(loc)
formattedTime := newYorkTime.Format("2006-01-02 15:04:05 MST")
fmt.Println(formattedTime)
}
Output:
2023-10-15 10:30:45 EDT
In this example, we load a specific time zone using time.LoadLocation
. We then convert the current time to that time zone using the In
method. Finally, we format the time to include the time zone abbreviation in the output. This is particularly useful for applications that need to display local times to users based on their geographical locations.
Conclusion
Converting time to a string in Go is a fundamental skill that can enhance the usability of your applications. By leveraging the time
package, you can format timestamps in various ways, parse strings into time objects, and handle time zones effectively. Whether you are displaying data in a user interface or logging events for analysis, mastering time formatting will improve your application’s functionality and user experience. With the examples and explanations provided in this tutorial, you should now feel empowered to work with time in Go confidently.
FAQ
-
How do I format time in Go?
You can format time in Go using theTime.Format
method, specifying a layout string that represents the desired format. -
What is the reference time used in Go for formatting?
The reference time in Go is “Mon Jan 2 15:04:05 MST 2006”, and it is used to define the layout for formatting. -
Can I parse a string into a time object in Go?
Yes, you can parse a string into a time object using thetime.Parse
function, which takes a layout and a string as arguments. -
How do I handle time zones in Go?
You can handle time zones in Go using thetime.LoadLocation
function to load a specific time zone and theIn
method to convert the time to that zone. -
What should I do if I encounter an error while parsing time?
If you encounter an error while parsing time, you should handle it gracefully, typically by checking for the error and providing feedback, such as printing an error message.
Related Article - Go Time
Related Article - Go String
- How to Convert String to Integer Type in Go
- How to Convert an Int Value to String in Go
- How to Parse Date String in Go
- How to Efficiently Concatenate Strings in Go
- How to Write Multiline Strings in Go
- How to Read a File Into String in Go