Time Duration in Go
- Duration Instances Conversion
-
Use the
time.duration
Method -
Calculate the Elapsed Time Using
time
Library - Convert Time Durations Into a Number in Go
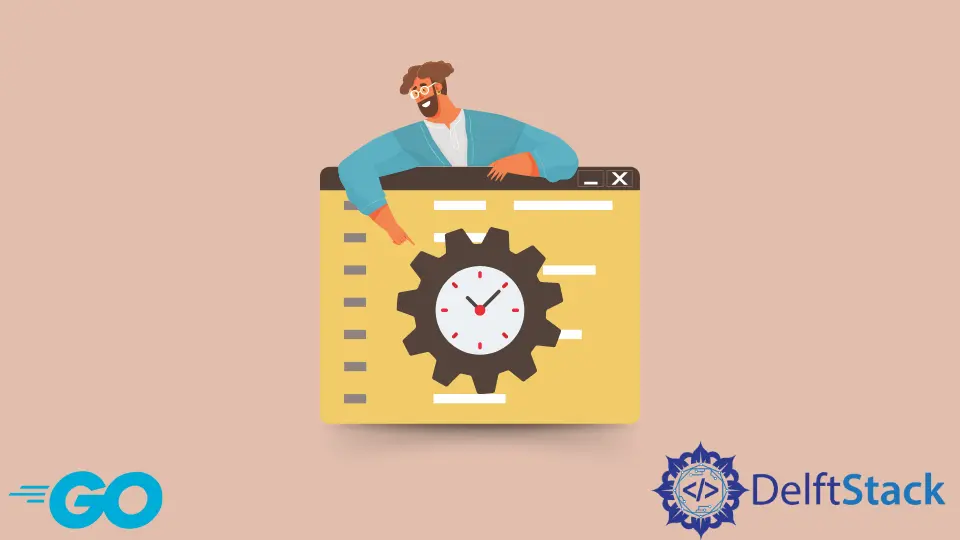
Go time duration conversions can be done in multiple ways. The time
library and time.duration
methods are used frequently for calculating and displaying time.
Note that Duration
refers to the time elapsed between two defined time objects as an int64
nanosecond
count.
Duration Instances Conversion
Nanosecond | 1 |
Microsecond | 1000 * Nanosecond |
Millisecond | 1000 * Microsecond |
Second | 1000 * Millisecond |
Minute | 60 * Second |
Hour | 60 * Minute |
Use the time.duration
Method
package main
import (
"fmt"
"time"
)
func main() {
d, err := time.ParseDuration("1h25m10.9183256645s")
if err != nil {
panic(err)
}
count := []time.Duration{
time.Nanosecond,
time.Microsecond,
time.Millisecond,
time.Second,
2 * time.Second,
time.Minute,
10 * time.Minute,
time.Hour,
}
for _, r := range count {
fmt.Printf("(%6s) = %s\n", r, d.Round(r).String())
}
}
Output:
( 1ns) = 1h25m10.918325664s
( 1µs) = 1h25m10.918326s
( 1ms) = 1h25m10.918s
( 1s) = 1h25m11s
( 2s) = 1h25m10s
( 1m0s) = 1h25m0s
( 10m0s) = 1h30m0s
(1h0m0s) = 1h0m0s
The above code allows us to calculate all the respective durations as a floating-point integer.
Calculate the Elapsed Time Using time
Library
package main
import (
"fmt"
"time"
)
func main() {
var t time.Duration = 100000000000000
fmt.Println(t.Hours())
fmt.Println(t.Minutes())
fmt.Println(t.Seconds())
now := time.Now()
time.Sleep(100000)
diff := now.Sub(time.Now())
fmt.Println("Elapsed time in seconds: ", diff.Seconds())
}
Output:
27.77777777777778
1666.6666666666667
100000
Elapsed time in seconds: -0.0001
The elapsed time tells us how long it took for the processor to calculate the time durations.
Convert Time Durations Into a Number in Go
package main
import (
"fmt"
"time"
)
func main() {
timeInput := 3600
data := time.Duration(timeInput) * time.Millisecond
fmt.Println("The time in Nanoseconds:", int64(data/time.Nanosecond))
fmt.Println("The time in Microseconds:", int64(data/time.Microsecond))
fmt.Println("The time in Milliseconds:", int64(data/time.Millisecond))
}
Output:
The time in Nanoseconds: 3600000000
The time in Microseconds: 3600000
The time in Milliseconds: 3600
The above code allows us to convert a number into a time instance, and then the time.Duration
performs time conversions. In the end, the time instance is converted back into a number.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn