How to Convert Boolean Into a String in Go
-
Use the
strconv.FormatBool
Function to Convert Boolean Into a String in Go -
Use the
Sprintf
Function to Convert Boolean Into a String in Go - Using Map to Convert Boolean Into a String in Go
- Conclusion
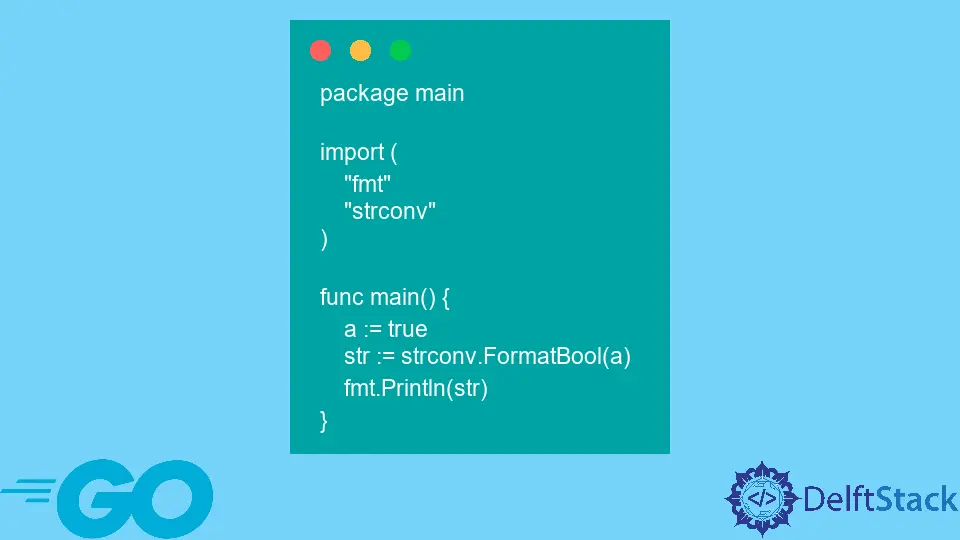
In Go, converting a Boolean value into a string is a common operation with multiple approaches. This process is crucial when dealing with scenarios where Boolean values need to be represented as strings, such as in data formatting or output generation.
This article will introduce the methods to convert a Boolean to a string data type in Go.
Use the strconv.FormatBool
Function to Convert Boolean Into a String in Go
In Go, strconv.FormatBool
is a function provided by the strconv
package. This function is specifically designed to convert a Boolean value into its string representation.
Basic Syntax:
str := strconv.FormatBool(true)
In the syntax, we convert the Boolean value true
to its string representation using the strconv.FormatBool
function and assigns the result to the variable str
.
Code:
package main
import (
"fmt"
"strconv"
)
func main() {
a := true
str := strconv.FormatBool(a)
fmt.Println(str)
}
In this code, we initialize a Boolean variable a
with the value true
. Using the strconv.FormatBool
function from the strconv
package, we convert this Boolean value into its corresponding string representation.
The result is printed to the console using fmt.Println
.
Output:
true
The output will be true
, reflecting the successful conversion of the Boolean value to its string equivalent.
In the next example, strconv.FormatBool
receives a Boolean value as an argument and transforms it into a string.
Code:
package main
import (
"fmt"
"strconv"
)
func main() {
boolVal := true
strVal := strconv.FormatBool(boolVal)
fmt.Printf("Type of strVal: %T\n", strVal)
fmt.Printf("Type of boolVal: %T\n", boolVal)
fmt.Println()
fmt.Printf("Value of strVal: %v\n", strVal)
fmt.Printf("Value of boolVal: %v", boolVal)
}
In this code, we declare a Boolean variable boolVal
with the value true
. Utilizing the strconv.FormatBool
function, we convert this Boolean value into a corresponding string stored in the variable strVal
.
We then use fmt.Printf
statements to display the types and values of both strVal
and boolVal
.
Output:
Type of strVal: string
Type of boolVal: bool
Value of strVal: true
Value of boolVal: true
The output reveals that the type of strVal
is a string, as expected, while the type of boolVal
is a Boolean. The subsequent lines print the actual values, confirming the successful conversion of the Boolean to its string representation.
Use the Sprintf
Function to Convert Boolean Into a String in Go
Using Sprintf
to convert a Boolean into a string in Go involves utilizing the fmt.Sprintf
function from the fmt
package. This function allows us to format a string based on a format specifier, and in this case, it can be used to convert a Boolean value to its string representation.
Basic Syntax:
strVal := fmt.Sprintf("%v", true)
In this syntax, fmt.Sprintf
is used with the format specifier %v
to convert the Boolean value true
to its string representation. The resulting string is stored in the variable strVal
.
You can replace true
with any Boolean variable or expression that you want to convert to a string using this approach.
Code:
package main
import (
"fmt"
)
func main() {
boolVal := false
strVal := fmt.Sprintf("%v", boolVal)
fmt.Printf("Type of strVal: %T\n", strVal)
fmt.Printf("Type of boolVal: %T\n", boolVal)
fmt.Println()
fmt.Printf("Value of strVal: %v\n", strVal)
fmt.Printf("Value of boolVal: %v", boolVal)
}