Convertir un booléen en une chaîne en Go
Jay Singh
30 janvier 2023
-
Utilisez
FormatBool
pour convertir un booléen en une chaîne dans Go -
Utilisez
Sprintf
pour convertir un booléen en une chaîne en Go
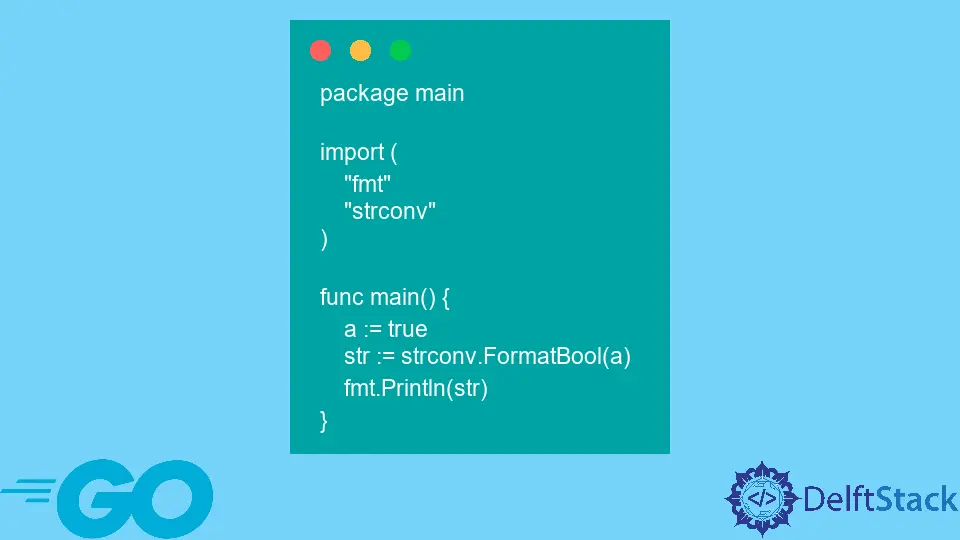
Cet article présentera les méthodes pour convertir un booléen en un type de données chaîne dans Go.
Utilisez FormatBool
pour convertir un booléen en une chaîne dans Go
Dans l’exemple ci-dessous, FormatBool
renvoie vrai ou faux selon la valeur de a
.
package main
import (
"fmt"
"strconv"
)
func main() {
a := true
str := strconv.FormatBool(a)
fmt.Println(str)
}
Production:
true
Dans l’exemple suivant, FormatBool
reçoit une valeur booléenne en argument et la transforme en chaîne.
package main
import (
"fmt"
"strconv"
)
func main() {
boolVal := true
strVal := strconv.FormatBool(boolVal)
fmt.Printf("Type of strVal: %T\n", strVal)
fmt.Printf("Type of boolVal: %T\n", boolVal)
fmt.Println()
fmt.Printf("Value of strVal: %v\n", strVal)
fmt.Printf("Value of boolVal: %v", boolVal)
}
Production:
Type of strVal: string
Type of boolVal: bool
Value of strVal: true
Value of boolVal: true
Utilisez Sprintf
pour convertir un booléen en une chaîne en Go
En utilisant la fonction Sprintf
, nous pouvons également convertir un booléen en une chaîne.
package main
import (
"fmt"
)
func main() {
boolVal := false
strVal := fmt.Sprintf("%v", boolVal)
fmt.Printf("Type of strVal: %T\n", strVal)
fmt.Printf("Type of boolVal: %T\n", boolVal)
fmt.Println()
fmt.Printf("Value of strVal: %v\n", strVal)
fmt.Printf("Value of boolVal: %v", boolVal)
}
Production:
Type of strVal: string
Type of boolVal: bool
Value of strVal: false
Value of boolVal: false
Article connexe - Go String
- Comment analyser la chaîne de dates dans Go
- Comment concaténer efficacement des chaînes dans Go
- Comment convertir une chaîne de caractères en un nombre entier en Go
- Comment convertir une valeur int en chaîne dans Go
- Comment écrire des chaînes de caractères multilignes dans Go
- Convertir une chaîne en tableau d'octets dans Golang