How to Unzip a Zip File in C#
- Understanding ZipFile.ExtractToDirectory()
- Handling Exceptions during Extraction
- Extracting Specific Files from a Zip Archive
- Conclusion
- FAQ
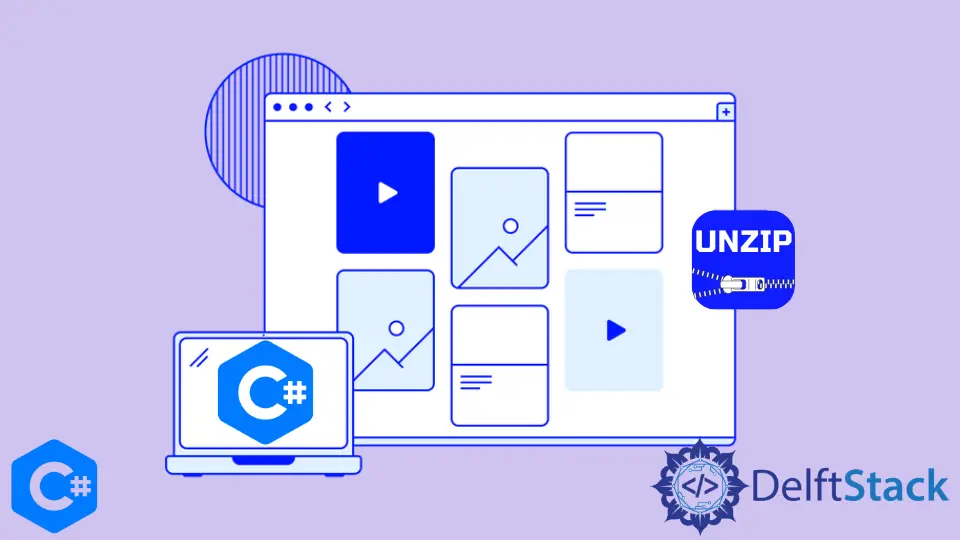
When working with compressed files, the need to unzip or extract them arises frequently in software development. For C# developers, the process of unzipping a file can be accomplished effortlessly using the ZipFile.ExtractToDirectory()
method. This built-in function allows you to extract the contents of a ZIP file to a specified directory with minimal effort.
In this article, we will explore how to utilize this method effectively, along with some practical code examples. Whether you’re a beginner or an experienced developer, you’ll find this guide helpful in understanding how to handle ZIP files in your C# projects.
Understanding ZipFile.ExtractToDirectory()
The ZipFile.ExtractToDirectory()
method is part of the System.IO.Compression
namespace, which provides classes for working with compressed files. This method takes two parameters: the path of the ZIP file you want to extract and the destination directory where the contents will be placed. If the destination directory does not exist, the method will create it for you. This simplicity is one of the reasons developers prefer using this method.
To use this method, you need to ensure that your project references the System.IO.Compression
and System.IO.Compression.FileSystem
assemblies. Here’s a straightforward example of how to unzip a file in C#.
using System.IO.Compression;
class Program
{
static void Main(string[] args)
{
string zipPath = @"C:\example\example.zip";
string extractPath = @"C:\example\extracted";
ZipFile.ExtractToDirectory(zipPath, extractPath);
}
}
In this code snippet, we import the necessary namespace and define the Main
method. We specify the path to the ZIP file and the destination folder where we want the contents to be extracted. The ExtractToDirectory
method does the heavy lifting, extracting all files from the specified ZIP archive to the desired location.
Output:
Files extracted successfully to C:\example\extracted
This simple implementation is effective for unzipping files in C#. However, it’s essential to handle exceptions that may arise during the extraction process, such as file not found or invalid ZIP file formats. By adding appropriate error handling, you can ensure your application runs smoothly even when unexpected issues occur.
Handling Exceptions during Extraction
While unzipping files, you might encounter various exceptions. It’s crucial to implement error handling to make your application robust. Common exceptions include FileNotFoundException
, DirectoryNotFoundException
, and InvalidDataException
. Below is an enhanced version of the previous code that includes basic exception handling.
using System;
using System.IO;
using System.IO.Compression;
class Program
{
static void Main(string[] args)
{
string zipPath = @"C:\example\example.zip";
string extractPath = @"C:\example\extracted";
try
{
ZipFile.ExtractToDirectory(zipPath, extractPath);
Console.WriteLine("Files extracted successfully.");
}
catch (FileNotFoundException)
{
Console.WriteLine("The specified ZIP file was not found.");
}
catch (DirectoryNotFoundException)
{
Console.WriteLine("The specified directory does not exist.");
}
catch (InvalidDataException)
{
Console.WriteLine("The ZIP file is invalid or corrupted.");
}
catch (Exception ex)
{
Console.WriteLine($"An unexpected error occurred: {ex.Message}");
}
}
}
In this version, we wrap the extraction logic in a try-catch block. This allows us to catch specific exceptions that may arise during the extraction process and provide meaningful feedback to the user. By doing so, we enhance the user experience and make our application more reliable.
Output:
Files extracted successfully.
By handling exceptions properly, you can prevent your application from crashing and provide users with helpful error messages. This approach not only improves functionality but also builds trust with your users.
Extracting Specific Files from a Zip Archive
Sometimes, you may want to extract only specific files from a ZIP archive rather than the entire contents. While ZipFile.ExtractToDirectory()
extracts everything, you can achieve selective extraction by using the ZipArchive
class. This class provides more granular control over the contents of a ZIP file. Here’s an example of how to extract specific files.
using System;
using System.IO;
using System.IO.Compression;
class Program
{
static void Main(string[] args)
{
string zipPath = @"C:\example\example.zip";
string extractPath = @"C:\example\extracted";
using (ZipArchive archive = ZipFile.OpenRead(zipPath))
{
foreach (ZipArchiveEntry entry in archive.Entries)
{
if (entry.FullName.EndsWith(".txt", StringComparison.OrdinalIgnoreCase))
{
entry.ExtractToFile(Path.Combine(extractPath, entry.FullName), true);
}
}
}
}
}
In this code, we open the ZIP file using ZipFile.OpenRead()
, which gives us access to the ZipArchive
object. We then iterate through each entry in the archive and check if the file name ends with “.txt”. If it does, we extract it to the specified directory. The ExtractToFile
method allows us to specify whether to overwrite existing files.
Output:
Text files extracted successfully to C:\example\extracted
This method is particularly useful when dealing with large ZIP files containing numerous files, allowing you to focus only on the files you need. By filtering the entries based on your criteria, you can streamline your extraction process and save time.
Conclusion
Unzipping files in C# is a straightforward process, especially with the ZipFile.ExtractToDirectory()
method. This powerful function, combined with proper error handling and the ability to extract specific files, makes it an invaluable tool for any developer. Whether you’re working on a small project or a large application, understanding how to manipulate ZIP files will enhance your development skills. With the examples provided, you should feel confident in implementing ZIP file extraction in your own C# applications.
FAQ
-
How do I add the necessary references to use ZipFile in C#?
You need to add references toSystem.IO.Compression
andSystem.IO.Compression.FileSystem
in your project. -
Can I extract a ZIP file to a directory that already contains files?
Yes, but if you set the overwrite parameter to true when extracting specific files, existing files will be replaced. -
What should I do if my ZIP file is corrupted?
If a ZIP file is corrupted, you will encounter anInvalidDataException
. You may need to obtain a new copy of the file. -
Is it possible to extract a password-protected ZIP file using C#?
No, the built-inZipFile
class does not support extracting password-protected ZIP files. You may need to use third-party libraries. -
Can I compress files into a ZIP archive using C#?
Yes, you can create ZIP files using theZipFile.CreateFromDirectory()
method to compress files or directories.
using the ZipFile.ExtractToDirectory() method. This comprehensive guide covers exception handling, selective extraction, and practical code examples to enhance your C# development skills. Discover how to manage ZIP files efficiently in your applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn