How to Get the Size of an Array in C#
-
Get the Size of Array With the
Array.Length
Property inC#
-
Get Size of Each Dimension of a Multi-Dimensional Array With the
Array.Rank
Property and theArray.GetLength()
Function inC#
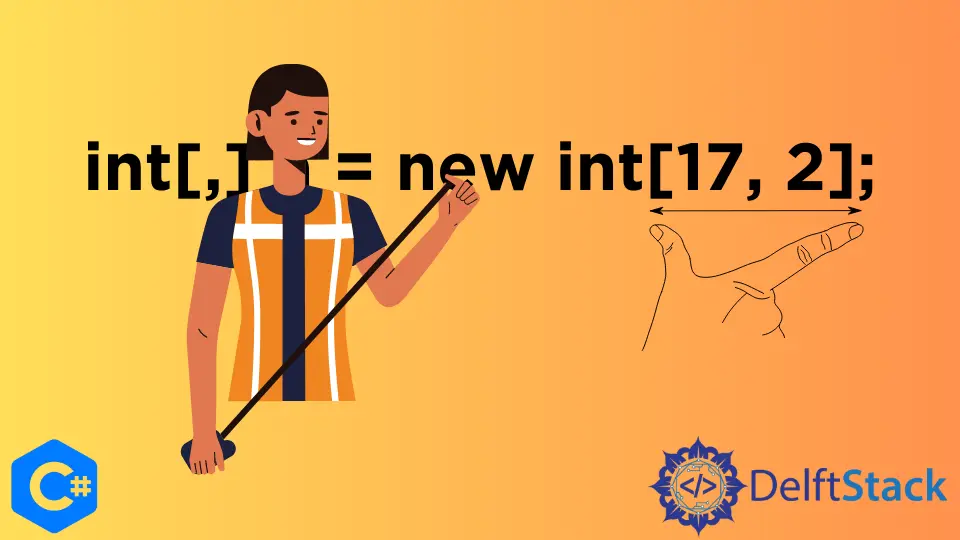
This tutorial will discuss methods to get the size of an array in C#.
Get the Size of Array With the Array.Length
Property in C#
The size of an array means the total number of elements an array can store in it. The Array.Length
property gives us the total size of an array in C#. The following code example shows us how to get the length of an array with the Array.Length
property in C#.
using System;
namespace size_of_array {
class Program {
static void method1() {
int[] a = new int[17];
Console.WriteLine(a.Length);
}
static void Main(string[] args) {
method1();
}
}
}
Output:
17
In the above code, we get the length of the a
array with the a.Length
property in C#. This method can also be used to get the total size of a multi-dimensional array. The code to determine the total size of a 2-D array is given below.
using System;
namespace size_of_array {
class Program {
static void method1() {
int[,] a = new int[17, 2];
Console.WriteLine(a.Length);
}
static void Main(string[] args) {
method1();
}
}
}
Output:
34
Get Size of Each Dimension of a Multi-Dimensional Array With the Array.Rank
Property and the Array.GetLength()
Function in C#
Suppose we have a multi-dimensional array, and we want to get each dimension’s size inside the multi-dimensional array. In that case, we have to use the Array.Rank
property and the [Array.GetLength()
function](https://learn.microsoft.com/en-us/dotnet/api/system.array.getlength?view=net-8.0&viewFallbackFrom=net-5.0%2F) in C#. The Array.Rank
property gives us the number of dimensions inside the array. The Array.GetLength(i)
function gives us the size of the i
dimension of the array. The following code example shows us how we can get the total size of each dimension of a multi-dimensional array with the Array.Rank
property and the Array.GetLength()
function in C#.
using System;
namespace size_of_array {
class Program {
static void method2() {
int[,] a = new int[17, 2];
int i = a.Rank;
for (int x = 0; x < i; x++) {
Console.WriteLine(a.GetLength(x));
}
}
static void Main(string[] args) {
method2();
}
}
}
Output:
17
2
In the above code, we print the size of each dimension of the multi-dimensional array a
with the a.Rank
property and the a.GetLength(x)
function. We get the number of dimensions inside the a
array with the a.Rank
property and iterate through each dimension using a for
loop. Then we print each dimension’s size with the a.GetLength(x)
function, where x
is the index of the dimension.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn