How to Shuffle an Array in C#
-
Use the
Random
Class to Shuffle Array in C# -
Use the
RandomNumberGenerator
Class to Shuffle an Array in C# - Use the Fisher-Yates Shuffle (Knuth Shuffle) Algorithm to Shuffle an Array in C#
- Conclusion
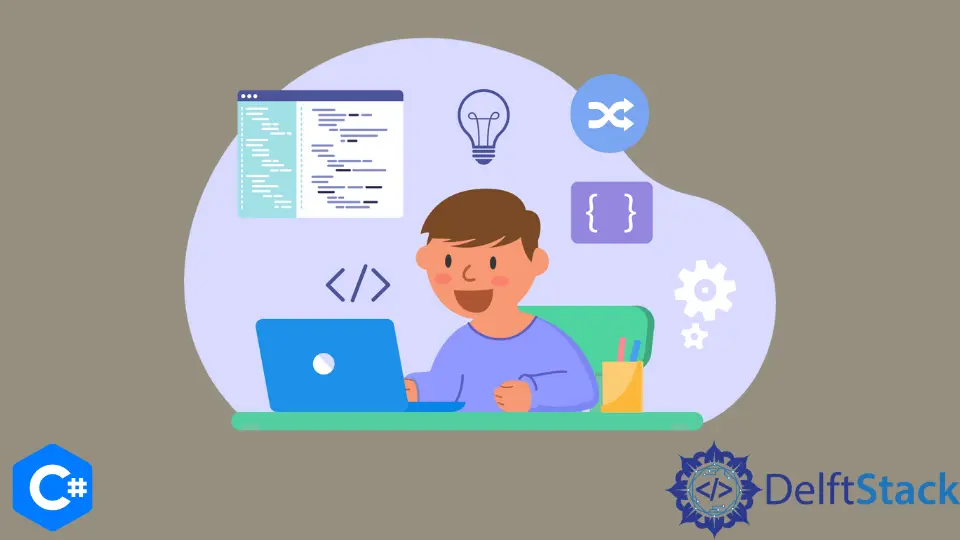
Shuffling is a common operation in programming, often used for randomizing data or creating varied outputs. There are three approaches available to do this in C#, and each of them utilizes different techniques and classes for achieving array shuffling.
In this article, we will explore the three ways to shuffle an array in C# and show examples of how to use it effectively.
Use the Random
Class to Shuffle Array in C#
Using the Random
class to shuffle an array in C# involves leveraging the capabilities of the Random
class to generate random indices within the array and swapping elements at those indices.
The Random.Next()
method generates a random integer value. We can use the Random.Next()
method with LINQ to shuffle an array in C#.
using System;
using System.Linq;
using System.Security.Cryptography;
class Program {
static void Main(string[] args) {
int[] arr = { 1, 2, 3, 4, 5 };
Random random = new Random();
arr = arr.OrderBy(x => random.Next()).ToArray();
foreach (var i in arr) {
Console.WriteLine(i);
}
}
}
Output:
1
5
2
4
3
In this code, we are utilizing the Random
class to shuffle an array of integers. The array arr
initially contains the values 1
through 5
in ascending order.
The Random
class is employed to generate random indices for each element in the array during the ordering process. The OrderBy
method, in combination with the random.Next()
function, rearranges the array elements based on their randomly generated indices.
This results in a randomized order of the elements within the array. The foreach
loop then iterates through the shuffled array, and we use Console.WriteLine(i)
to print each element on a new line.
The output of this program will display the integers 1
through 5
in a random order, with the sequence changing each time the program is executed.
Use the RandomNumberGenerator
Class to Shuffle an Array in C#
Using the RandomNumberGenerator
class to shuffle an array in C# involves leveraging a cryptographic-strength random number generator to produce random indices for shuffling. Unlike the Random
class, RandomNumberGenerator
provides a more secure source of randomness, making it suitable for cryptographic applications.
using System;
using System.Linq;
using System.Security.Cryptography;
class Program {
static int Next(RandomNumberGenerator random) {
byte[] randomInt = new byte[4];
random.GetBytes(randomInt);
return BitConverter.ToInt32(randomInt, 0);
}
static void Main(string[] args) {
int[] arr = { 1, 2, 3, 4, 5 };
RandomNumberGenerator random = RandomNumberGenerator.Create();
arr = arr.OrderBy(x => Next(random)).ToArray();
foreach (var i in arr) {
Console.WriteLine(i);
}
}
}
Output:
5
1
4
2
3
In this code, we are employing the more secure RandomNumberGenerator
class to shuffle an array of integers. We have defined a method named Next
, which takes a RandomNumberGenerator
instance and generates a random integer by obtaining a byte array through GetBytes
.
The array arr
initially contains the values 1
through 5
in ascending order. We create an instance of RandomNumberGenerator
using RandomNumberGenerator.Create()
to ensure cryptographic-strength randomness.
The OrderBy
method, utilizing our custom Next
method, rearranges the array elements based on the generated random integers. This ensures a more secure and cryptographically robust shuffling process.
Finally, we use a foreach
loop to iterate through the shuffled array, and with Console.WriteLine(i)
, we print each element on a new line. The output of this program will display the integers 1
through 5
in a randomized order, with the sequence changing each time the program is executed due to the cryptographic randomness introduced by RandomNumberGenerator
.
Use the Fisher-Yates Shuffle (Knuth Shuffle) Algorithm to Shuffle an Array in C#
The Knuth Shuffle, also known as the Fisher-Yates Shuffle, is a specific algorithm designed to shuffle an array uniformly at random. The algorithm starts with the last element of the array, selects a random element before it (or including itself), and swaps them, and it continues this process for each element in the array.
The Random
class and the Knuth Shuffle algorithm are related but serve different purposes when it comes to shuffling arrays. The key difference lies in purpose and specificity.
The Random
class is a general-purpose random number generator, while the Knuth Shuffle is a specific algorithm for shuffling arrays uniformly at random.
using System;
class Program {
static void FisherYatesShuffle<T>(T[] array) {
Random random = new Random();
int n = array.Length;
for (int i = n - 1; i > 0; i--) {
int j = random.Next(0, i + 1);
T temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
static void Main(string[] args) {
int[] arr = { 1, 2, 3, 4, 5 };
FisherYatesShuffle(arr);
foreach (var i in arr) {
Console.WriteLine(i);
}
}
}
Output:
1
4
3
2
5
In this code, we are implementing the Fisher-Yates Shuffle, also known as the Knuth Shuffle algorithm, to shuffle an array of integers. The array arr
initially contains the values 1
through 5
in ascending order.
Then, we have defined a generic method called FisherYatesShuffle
, which takes an array as input and shuffles its elements using the Random
class to generate random indices. The algorithm starts with the last element and iteratively swaps it with a randomly selected element before it (or itself).
This process continues until the entire array is shuffled. In the Main
method, we invoke the FisherYatesShuffle
method to shuffle the array, and then we use a foreach
loop to iterate through the shuffled array.
With Console.WriteLine(i)
, we print each element on a new line. Consequently, the output of this program will display the integers 1
through 5
in a randomly shuffled order, and the sequence will vary each time the program is executed due to the inherent randomness of the Fisher-Yates Shuffle algorithm.
Conclusion
In conclusion, this tutorial provides an overview of various methods to shuffle arrays in C#. Whether using the Random
class for general scenarios, the RandomNumberGenerator
class for cryptographic applications, or the Fisher-Yates Shuffle algorithm for uniform randomness, we can choose the method that best fits our specific needs.
Understanding these approaches enhances the flexibility and applicability of array shuffling in C# programs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn