How to Append to Array in C#
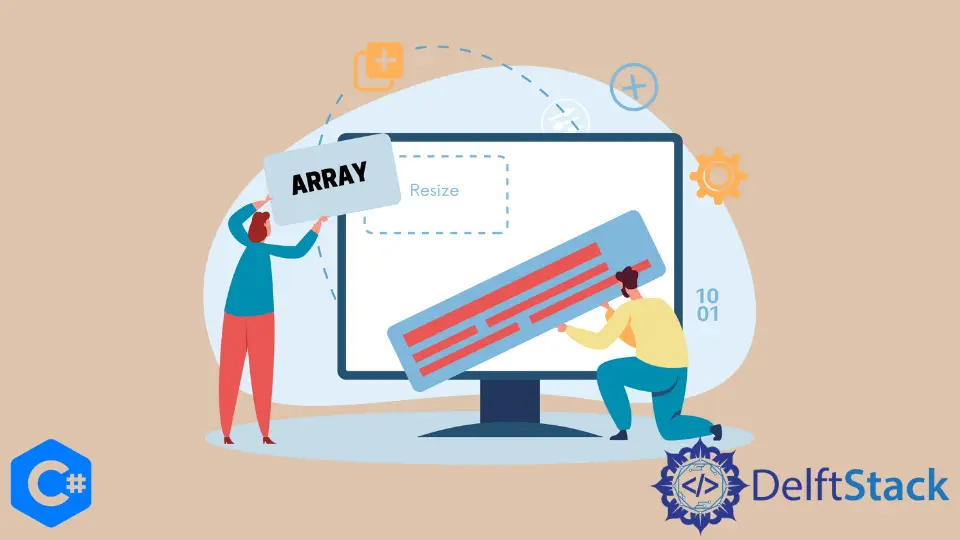
This tutorial will introduce methods to append elements to an array in C#.
Resize an Array With Lists in C#
Unfortunately, by default, we cannot dynamically change the size of an array. If we have an array and want to append more values to the same array, we have to rely on some user-defined approaches for resizing the array. We can resize an array by first converting the array to a list with the ToList()
function in C#. We can then add more values to the list with the List.Add()
function and convert the list back to an array using the ToArray()
function in C#. The following code example shows us how we can resize an array by using lists in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace Resize_Array {
class Program {
static void method1() {
string[] arr = new string[] { "Hi" };
List<string> ls = arr.ToList();
ls.Add("Hello");
ls.Add("World");
arr = ls.ToArray();
foreach (var e in arr) {
Console.WriteLine(e);
}
}
static void Main(string[] args) {
method1();
}
}
}
Output:
Hi
Hello
World
We converted the array arr
to the list ls
with the arr.ToList()
function. We added new elements to the ls
and converted it back to an array with the ls.ToArray()
function in C#.
Resize an Array With the Array.Resize()
Method in C#
We can also use the Array.Resize()
method to achieve the same objective in C#. The Array.Resize()
method takes the pointer to an array as a parameter and changes that array’s size to a specified number. The following code example shows us how we can resize an array with the Array.Resize()
function in C#.
using System;
using System.Collections.Generic;
namespace Resize_Array {
class Program {
static void method2() {
string[] arr = new string[] { "Hi" };
Array.Resize(ref arr, 3);
arr[1] = "Hello";
arr[2] = "World";
foreach (var e in arr) {
Console.WriteLine(e);
}
}
static void Main(string[] args) {
method2();
}
}
}
Output:
Hi
Hello
World
We resized the array arr
with the Array.Resize()
method in C#. We passed the reference to the arr
and the desired size of the arr
in the parameters of the Array.Resize()
method. We appended new elements into the arr
array and displayed the array to the user.
Both the methods discussed above can be used to append elements to an array. But for dynamic memory allocation, the list data structure should be used instead of the array data structure.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn