How to Print Array in C#
-
Print an Array With the
String.Join()
Method inC#
-
Print an Array With the
List.ForEach()
Method inC#
-
Print an Array With the
foreach
Loop inC#
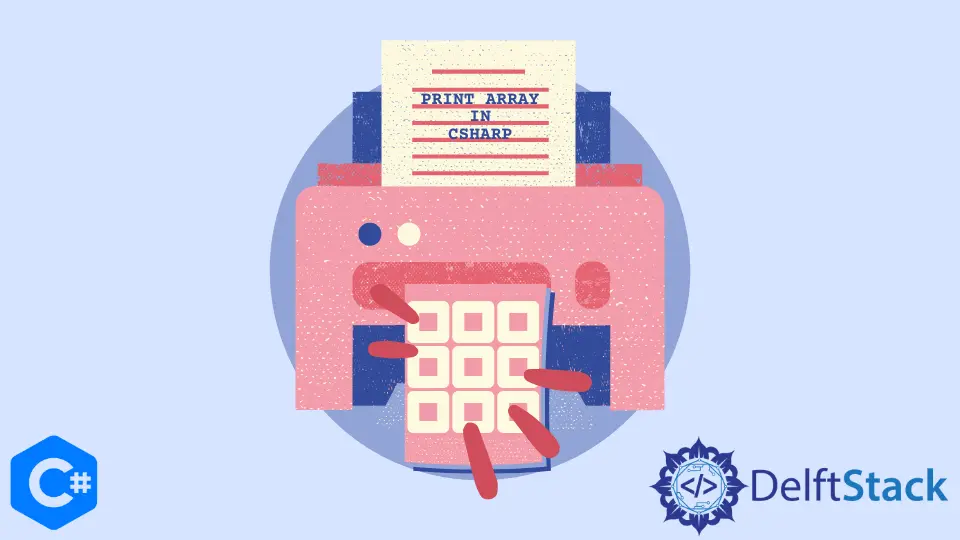
This tutorial will discuss the methods to print an array of strings in C#.
Print an Array With the String.Join()
Method in C#
The String.Join()
method concatenates the elements of a specified array with a specified separator between them in C#. We can use the \n
escape sequence as a separator to place each element of the array in a separate line. The following code example shows us how to print an array of string variables with the String.Join()
method in C#.
using System;
namespace print_string_array {
class Program {
static void Main(string[] args) {
string[] arr = new string[] { "one", "two", "three", "four" };
Console.WriteLine(String.Join("\n", arr));
}
}
}
Output:
one
two
three
four
We initialized an array of string variables arr
and printed each element in a new line with the String.Join("\n", arr)
function in C#. The String.Join()
function returns a string variable. So, we can either store the returned value inside a string variable and then display it or directly use the String.Join()
function inside the Console.WriteLine()
function.
Print an Array With the List.ForEach()
Method in C#
The ForEach()
method performs a specified action on each element of a list in C#. We can print each element of our array with the List.ForEach()
method by first converting the array to a list. We can use the ToList()
function in Linq to convert our array into a list. See the following example.
using System;
using System.Linq;
namespace print_string_array {
class Program {
static void Main(string[] args) {
string[] strArray = new string[] { "abc", "def", "asd" };
strArray.ToList().ForEach(Console.WriteLine);
}
}
}
Output:
abc
def
asd
We initialized an array of strings strArray
and printed all the elements of the strArray
array by first converting it to a list using the ToList()
function in Linq and then using ForEach()
on the resultant list.
Print an Array With the foreach
Loop in C#
The foreach
loop is used to iterate through a data structure in C#. We can also use the foreach
loop to iterate through each element of an array and print it. The following code example shows us how to print an array with the foreach
loop in C#.
using System;
namespace print_string_array {
class Program {
static void Main(string[] args) {
string[] arr = new string[] { "one", "two", "three", "four" };
foreach (var s in arr) {
Console.WriteLine(s);
}
}
}
}
Output:
one
two
three
four
We initialized an array of strings arr
and displayed each element of the arr
array with the foreach
loop in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn