How to Merge Two Arrays in C#
-
Merge Two Arrays With the
Array.Copy()
Method in C# -
Merge Two Arrays With the
Array.Resize()
Method in C# - Merge Two Arrays With the LINQ Method in C#
- Conclusion
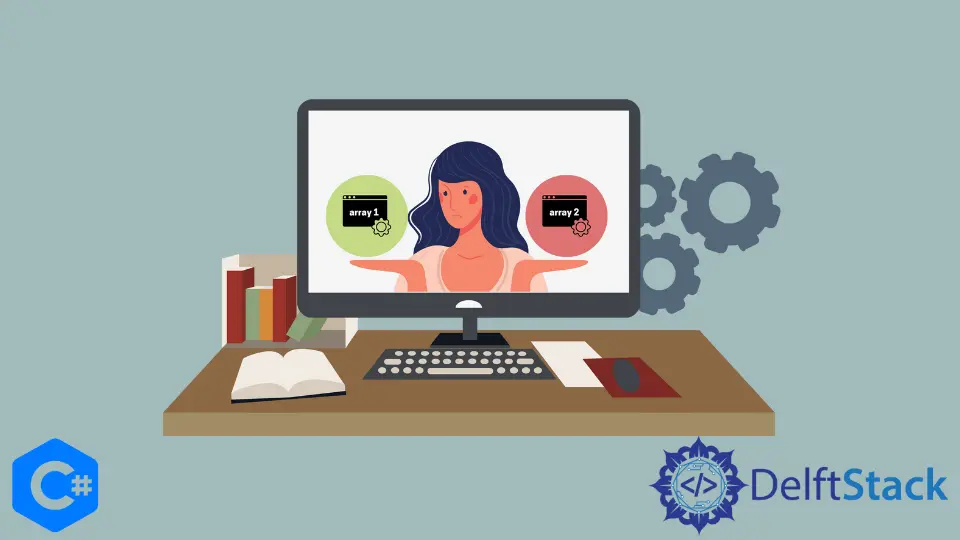
In this tutorial, we’ll delve into various methods for merging two arrays in C#. The article explores three distinct approaches to achieving array merging, each offering its advantages and use cases.
The methods covered include leveraging the Array.Copy()
method, utilizing the Array.Resize()
method in conjunction with copying, and employing the LINQ (Language Integrated Query) method for array concatenation.
Merge Two Arrays With the Array.Copy()
Method in C#
The Array.Copy()
method copies a range of elements from one array to another. We can use the Array.Copy()
method to copy both arrays’ elements to a third merged array.
Code Example:
using System;
class Program {
static void Main(string[] args) {
int[] arr1 = { 1, 2, 3 };
int[] arr2 = { 4, 5, 6 };
int[] arr3 = new int[arr1.Length + arr2.Length];
Array.Copy(arr1, arr3, arr1.Length);
Array.Copy(arr2, 0, arr3, arr1.Length, arr2.Length);
foreach (var e in arr3) {
Console.WriteLine(e);
}
}
}
Output:
1
2
3
4
5
6
In this code, we begin by declaring and initializing two arrays, arr1
and arr2
, with values [1, 2, 3]
and [4, 5, 6]
, respectively.
Subsequently, we create a third array, arr3
, with a length equal to the combined lengths of arr1
and arr2
. The purpose of arr3
is to serve as a container for the merged elements of the two original arrays.
To accomplish the array merging, we employ the Array.Copy()
method twice. In the first instance, we copy the elements from arr1
to arr3
.
Following that, in the second usage, we copy the elements from arr2
starting at index 0
of arr3
, effectively appending them to the end of the previously copied elements from arr1
. Finally, we utilize a foreach
loop to iterate through each element of the merged array, arr3
, and print them to the console using Console.WriteLine(e)
.
The output confirms the successful merging of the two arrays, arr1
and arr2
, into the new array arr3
, with the elements displayed in sequential order on the console.
Merge Two Arrays With the Array.Resize()
Method in C#
In the previous example, we had to create a separate third array to store both arrays’ merged values. If we want to achieve this goal without creating another array, we have to use the Array.Resize()
method on one of the two arrays.
The Array.Resize()
method is used to resize a one-dimensional array in C#. The Array.Resize()
method takes the reference to the array and the desired size as its arguments and resizes the array.
Code Example:
using System;
class Program {
static void Main(string[] args) {
int[] arr1 = { 1, 2, 3 };
int[] arr2 = { 4, 5, 6 };
int array1OriginalLength = arr1.Length;
Array.Resize<int>(ref arr1, array1OriginalLength + arr2.Length);
Array.Copy(arr2, 0, arr1, array1OriginalLength, arr2.Length);
foreach (var e in arr1) {
Console.WriteLine(e);
}
}
}
Output:
1
2
3
4
5
6
In this code, we initialize two arrays, arr1
and arr2
, with values [1, 2, 3]
and [4, 5, 6]
, respectively. We introduce a variable, array1OriginalLength
, to store the original length of arr1
.
The objective is to merge the arrays without creating a separate third array and to achieve this, we use the Array.Resize()
method. By resizing arr1
to accommodate the elements of arr2
, we ensure that it can hold the combined elements of both arrays.
Subsequently, the Array.Copy()
method is employed to copy the elements from arr2
into the resized arr1
, starting at the index equivalent to the original length of arr1
. Finally, a foreach
loop iterates through each element of the modified arr1
, printing them to the console using Console.WriteLine(e)
.
The output confirms the successful merging of the two arrays, arr1
and arr2
, with the elements displayed in sequential order on the console. The Array.Resize()
method efficiently expands the first array in place to accommodate the elements from the second array, resulting in a concise and effective merging operation.
Merge Two Arrays With the LINQ Method in C#
The LINQ or Language Integrated Query integrates the query functionality in data structures in C#.
We can use the Concat()
function to merge the elements of two arrays. The Concat(x)
function concatenates the elements of the parameter x
at the end of the calling object in C#.
We can then use the ToArray()
function to convert the result to an array.
Code Example:
using System;
using System.Linq;
class Program {
static void Main(string[] args) {
int[] arr1 = { 1, 2, 3 };
int[] arr2 = { 4, 5, 6 };
arr1 = arr1.Concat(arr2).ToArray();
foreach (var e in arr1) {
Console.WriteLine(e);
}
}
}
Output:
1
2
3
4
5
6
In this code, we initialize two arrays, arr1
and arr2
, with values [1, 2, 3]
and [4, 5, 6]
, respectively. To merge these arrays, we utilize LINQ’s Concat()
function, which seamlessly concatenates the elements of arr2
to the end of arr1
.
The result is assigned back to arr1
, effectively creating a new array containing the merged elements. Finally, a foreach
loop iterates through each element of the merged array, arr1
, printing them to the console using Console.WriteLine(e)
.
This output confirms the successful merging of the two arrays, arr1
and arr2
, with the elements displayed in sequential order on the console. The concise use of LINQ simplifies the merging process, providing an elegant and efficient solution for array concatenation in C#.
Conclusion
In conclusion, we explored three different methods for merging two arrays in C#.
The first method involved using the Array.Copy()
method to copy elements from both arrays into a third array. The second method utilized the Array.Resize()
method to dynamically resize one array and then copy the elements of the second array into the resized first array.
The third method demonstrated the use of LINQ’s Concat()
function to merge two arrays, followed by converting the result into a new array using ToArray()
. These methods provide flexibility in handling array merging based on specific requirements or preferences.
Whether creating a new array, resizing an existing one, or leveraging LINQ for concise code, C# offers multiple approaches for efficiently merging arrays.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn