How to Initialize a List of Tuples in C#
-
Initialize a List of Tuples in C# Using the
Tuple.Create()
Method -
Initialize a List of Tuples in C# Using the
()
Notation -
Initialize a List of Tuples in C# Using
List<Tuple<T1, T2>>
andAdd()
- Initialize a List of Tuples in C# Using LINQ
- Conclusion
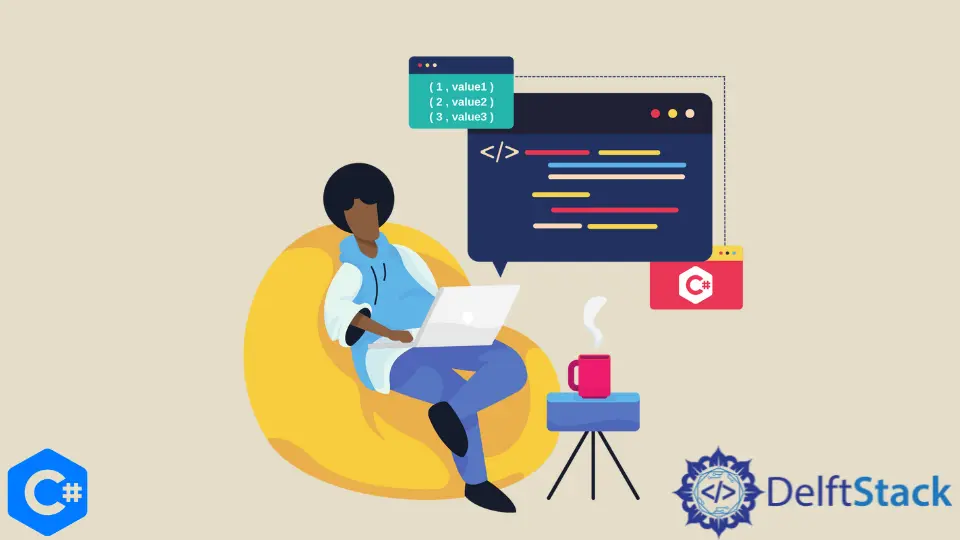
C# is a versatile and powerful programming language that provides various ways to work with data structures. When dealing with collections of related data, tuples offer a convenient solution.
A tuple is a fixed-size, ordered set of elements, and initializing a list of tuples in C# can be achieved through different methods. In this article, we will explore several approaches to efficiently initialize a list of tuples.
Initialize a List of Tuples in C# Using the Tuple.Create()
Method
Initializing a list of tuples in C# can be achieved using the Tuple.Create()
method, which simplifies the process of creating tuples with specified values.
The Tuple.Create()
method is a factory method provided by the Tuple
class in C#. It takes parameters representing the values of the tuple elements and returns a new instance of the Tuple
class initialized with those values.
The basic syntax is as follows:
Tuple<T1, T2, ..., TN> tupleInstance = Tuple.Create(value1, value2, ..., valueN);
Here, T1, T2, ..., TN
represent the types of the tuple elements, and value1, value2, ..., valueN
are the actual values.
Let’s now explore an example of initializing a list of tuples using the Tuple.Create()
method.
using System;
using System.Collections.Generic;
namespace TupleInitializationExample {
class Program {
static void Main(string[] args) {
// Initializing a list of tuples using Tuple.Create()
var tupleList =
new List<Tuple<int, string>> { Tuple.Create(1, "Apple"), Tuple.Create(2, "Banana"),
Tuple.Create(3, "Cherry") };
// Displaying the tuples in the list
foreach (var tuple in tupleList) {
Console.WriteLine(tuple);
}
}
}
}
In the provided code example, we begin by creating a namespace, TupleInitializationExample
. Inside this namespace, we define a class Program
containing the Main
method, which serves as the entry point of the program.
Within the Main
method, we declare a list named tupleList
to store tuples with elements of type int
and string
. The list is initialized using the new List<Tuple<int, string>>
syntax, and the Tuple.Create()
method is employed to create tuples with specific values.
In this case, three tuples are added to the list, each containing an integer and a string.
The foreach
loop is utilized to iterate through the tupleList
, and each tuple is printed to the console using Console.WriteLine(tuple)
.
Code Output:
(1, Apple)
(2, Banana)
(3, Cherry)
This output confirms that the list of tuples has been successfully initialized using the Tuple.Create()
method, with each tuple containing the specified values of integers and strings.
Initialize a List of Tuples in C# Using the ()
Notation
In addition to the Tuple.Create()
method, C# provides a concise and expressive syntax for initializing a list of tuples using the ()
notation. This notation leverages the value tuple feature introduced in C# 7.0, allowing for a more streamlined and readable code.
The ()
notation is a shorthand for creating value tuples in C#. It allows for the direct initialization of tuples without explicitly invoking the Tuple.Create()
method.
The syntax is straightforward:
List<(T1, T2, ..., TN)> tupleList = new List<(T1, T2, ..., TN)> {
(value1, value2, ..., valueN),
// Additional tuples can be added as needed
};
Here, (T1, T2, ..., TN)
represents the types of the tuple elements, and (value1, value2, ..., valueN)
are the actual values.
Let’s dive into a working example that demonstrates how to initialize a list of tuples using the ()
notation.
using System;
using System.Collections.Generic;
namespace TupleInitializationExample {
class Program {
static void Main(string[] args) {
// Initializing a list of tuples using () notation
var tupleList = new List<(int, string)> { (1, "Apple"), (2, "Banana"), (3, "Cherry") };
// Displaying the tuples in the list
foreach (var tuple in tupleList) {
Console.WriteLine(tuple);
}
}
}
}
In this example, we are still within the TupleInitializationExample
namespace, and the Program
class contains the Main
method. Similar to the previous example, we declare a list named tupleList
to store tuples with elements of type int
and string
.
However, instead of using Tuple.Create()
, we directly use the ()
notation to initialize the list. Three tuples are added to the list, each containing an integer and a string.
The foreach
loop iterates through the tupleList
, and each tuple is printed to the console using Console.WriteLine(tuple)
.
Code Output:
(1, Apple)
(2, Banana)
(3, Cherry)
This output demonstrates that the list of tuples has been successfully initialized using the ()
notation, providing a more concise and modern alternative to the Tuple.Create()
method.
Initialize a List of Tuples in C# Using List<Tuple<T1, T2>>
and Add()
You can initialize a list of tuples using the List<Tuple<T1, T2>>
type and the Add()
method. This approach provides a straightforward and explicit way to build a collection of tuples dynamically.
The syntax involves creating an instance of List<Tuple<T1, T2>>
and using the Add()
method to append tuples to the list. The basic structure is as follows:
// Create a list of tuples
List<Tuple<T1, T2>> tupleList = new List<Tuple<T1, T2>>();
// Add tuples to the list using the Add() method
tupleList.Add(new Tuple<T1, T2>(value1, value2));
tupleList.Add(new Tuple<T1, T2>(value3, value4));
// Additional tuples can be added as needed
Here, T1
and T2
represent the types of the tuple elements, and value1
, value2
, etc., are the actual values.
Let’s explore an example that demonstrates how to initialize a list of tuples using List<Tuple<T1, T2>>
with the Add()
method.
using System;
using System.Collections.Generic;
namespace TupleInitializationExample {
class Program {
static void Main(string[] args) {
var tupleList = new List<Tuple<int, string>>();
tupleList.Add(new Tuple<int, string>(1, "Apple"));
tupleList.Add(new Tuple<int, string>(2, "Banana"));
tupleList.Add(new Tuple<int, string>(3, "Cherry"));
foreach (var tuple in tupleList) {
Console.WriteLine(tuple);
}
}
}
}
In this example, within the TupleInitializationExample
namespace, the Program
class and Main
method are defined. We start by creating an empty list of tuples using List<Tuple<int, string>> tupleList = new List<Tuple<int, string>>();
.
Subsequently, individual tuples are added to the list using the Add()
method. Each Add()
call creates a new tuple with specified values and appends it to the tupleList
.
Finally, a foreach
loop is used to iterate through the list, and each tuple is printed to the console using Console.WriteLine(tuple)
.
Code Output:
(1, Apple)
(2, Banana)
(3, Cherry)
This output confirms that the list of tuples has been successfully initialized using List<Tuple<T1, T2>>
with the Add()
method, providing a practical way to build a collection of tuples.
Initialize a List of Tuples in C# Using LINQ
LINQ (Language-Integrated Query) provides another expressive way to initialize a list of tuples. LINQ allows for concise and readable code when working with collections, making it a versatile option for tuple initialization.
Using LINQ, we can leverage the Select
method to project each element of a collection into a new form, creating tuples in our case. The syntax is as follows:
List<Tuple<T1, T2>> tupleList =
Enumerable.Range(start, count).Select(i => new Tuple<T1, T2>(value1, value2)).ToList();
Here, start
and count
represent the range of values to generate, and value1
, value2
, etc., are the values used to initialize the tuples.
Here’s an example demonstrating how to initialize a list of tuples using LINQ.
using System;
using System.Collections.Generic;
using System.Linq;
namespace TupleInitializationExample {
class Program {
static void Main(string[] args) {
var tupleList =
Enumerable.Range(1, 3).Select(i => new Tuple<int, string>(i, $"Fruit{i}")).ToList();
foreach (var tuple in tupleList) {
Console.WriteLine(tuple);
}
}
}
}
Within the TupleInitializationExample
namespace, the Program
class and Main
method are defined. Here, we utilize LINQ to initialize a list of tuples.
The Enumerable.Range(1, 3)
generates a sequence of integers from 1 to 3, inclusive. The Select
method then transforms each integer into a new tuple using the lambda expression i => new Tuple<int, string>(i, $"Fruit{i}")
.
This lambda expression creates a tuple with an integer and a formatted string indicating a fruit.
The result is a list of tuples stored in the tupleList
. The foreach
loop iterates through this list; each tuple is printed to the console using Console.WriteLine(tuple)
.
Code Output:
(1, Fruit1)
(2, Fruit2)
(3, Fruit3)
This output confirms that the list of tuples has been successfully initialized using LINQ.
Conclusion
Initializing a list of tuples in C# offers various methods, each catering to different preferences and scenarios. Whether using the traditional Tuple.Create()
method, the ()
notation, LINQ, or List<Tuple<T1, T2>>
with the Add()
method, you have multiple options to suit your coding style and requirements.
Choosing the appropriate method depends on factors such as code readability, conciseness, and specific use cases.
The Tuple.Create()
method and ()
notation provide simplicity and brevity, while LINQ offers an expressive approach. The explicit List<Tuple<T1, T2>>
with the Add()
method allows for dynamic construction of tuple lists.
Ultimately, the choice between these methods hinges on your preferences and the demands of the particular application.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn