在 C# 中初始化元组列表
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp List
Csharp Tuple
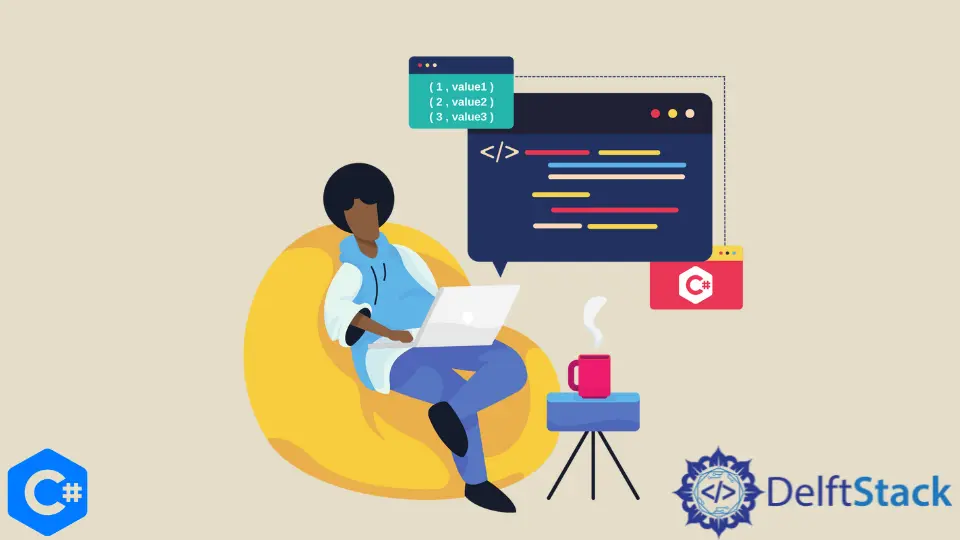
本教程将讨论在 C# 中初始化元组列表的方法。
在 C# 中使用 Tuple.Create()
方法初始化元组列表
C# 中的 Tuple.Create(x, y)
方法创建一个具有值 x
和 y
的新元组。我们可以创建一个元组列表,并在初始化列表时使用 Tuple.Create()
方法。请参见以下示例。
using System;
using System.Collections.Generic;
namespace list_of_tuples {
class Program {
static void Main(string[] args) {
var tupleList =
new List<Tuple<int, string>> { Tuple.Create(1, "value1"), Tuple.Create(2, "value2"),
Tuple.Create(3, "value3") };
foreach (var pair in tupleList) {
Console.WriteLine(pair);
}
}
}
}
输出:
(1, value1)
(2, value2)
(3, value3)
在上面的代码中,我们使用列表构造函数中的 Tuple.Create()
方法初始化了 (int, string)
的 tupleList
列表。这种方法很好用,但是有点多余,因为我们必须对 tupleList
列表中的每个元组使用 Tuple.Create()
方法。
在 C# 中使用 ()
表示法初始化元组列表
C# 中的 (x, y)
表示法指定具有 x
和 y
值的元组。除了 Tuple.Create()
函数外,我们还可以使用列表构造函数中的 ()
表示法来初始化元组列表。下面的代码示例向我们展示了如何使用 C# 中的 ()
表示法初始化元组列表。
using System;
using System.Collections.Generic;
namespace list_of_tuples {
class Program {
static void Main(string[] args) {
var tupleList = new List<(int, string)> { (1, "value1"), (2, "value2"), (3, "value3") };
foreach (var pair in tupleList) {
Console.WriteLine(pair);
}
}
}
}
输出:
(1, value1)
(2, value2)
(3, value3)
在上面的代码中,我们使用列表构造函数中的 (int, string)
标记初始化了元组 (int, string)
的 tupleList
列表。此方法比前面的示例更好,因为它不像前面的方法那样多余,并且执行相同的操作。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn