How to Initialize Array of Objects in C#
- Initializing an Array of Objects in C# with a Given Size
- Initializing an Array of Objects in C# by Defining the Array and Adding Values
- Initializing an Array of Objects in C# by Declaring an Array with Elements
- Initializing Arrays in C# Through Extension Methods
- Conclusion
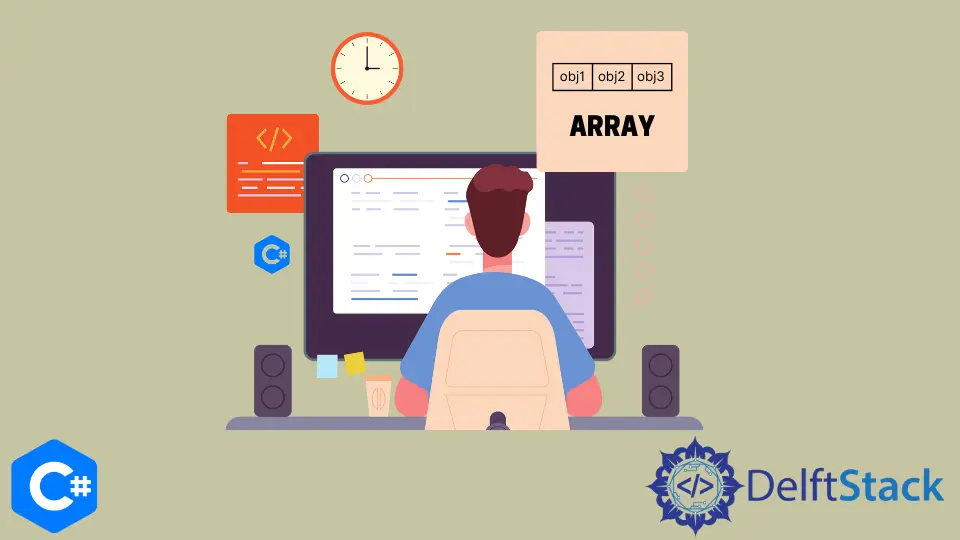
In the realm of software development, particularly in C#, efficiently managing collections of objects is a cornerstone of robust application design. Arrays, one of the fundamental data structures, play a crucial role in organizing and manipulating collections of objects.
This tutorial delves into various methods of initializing arrays of objects in C#.
Initializing an Array of Objects in C# with a Given Size
Initializing an array with a predetermined size is a fundamental concept, especially when dealing with collections of objects. This technique is particularly useful when you know the number of elements your array will hold, but the actual objects are to be assigned or created later in the program.
Let’s consider a practical example to illustrate this concept. Imagine we have a class named Book
that we will use to create an array of book objects.
Initially, we only know how many books we will have, but not their specific details.
Example:
using System;
class Book {
public string Title { get; set; }
public string Author { get; set; }
}
class Program {
static void Main() {
// Initialize an array of Book objects with a size of 5
Book[] library = new Book[5];
// Example of populating the array with Book objects
for (int i = 0; i < library.Length; i++) {
library[i] = new Book(); // Assign a new Book object to each element
}
// Optional: Demonstrate that the array contains Book objects
foreach (Book book in library) {
Console.WriteLine($"Book object: {book}");
}
}
}
In this code, we start by defining a Book
class with properties Title
and Author
. This class will serve as the blueprint for our book objects.
Inside the Main
method of the Program
class, we declare an array named library
that will hold our book objects. The line Book[] library = new Book[5];
initializes the library
array with a size of five.
This means it can hold five Book
objects. At this point, the array elements are null because we have only reserved the space for the Book
objects but haven’t created them yet.
The for
loop that follows is where we create and assign Book
objects to each element of the array. library[i] = new Book();
creates a new Book
object and assigns it to the current element in the array, as determined by the loop index i
.
Finally, we have an optional foreach
loop to demonstrate that the array indeed contains Book
objects. This loop iterates through each element in the library
array and prints a reference to the Book
object.
When this code is executed, it will output references to the Book
objects contained in the array. Since we haven’t assigned specific values to the Title
and Author
properties, these details are not shown.
Output:
Book object: Book
Book object: Book
Book object: Book
Book object: Book
Book object: Book
Initializing an Array of Objects in C# by Defining the Array and Adding Values
Initializing an array of objects in C# involves creating an array that can hold instances of a specific class or type and then populating that array with instances of the class. This process allows you to manage a collection of related objects in a structured manner, which is ideal when the size of the array and the objects to be added are known beforehand.
To explore this concept, let’s dive into an example where we have a Student
class, and we want to create an array of Student
objects. Each student has properties like Name
and Grade
.
Example:
using System;
class Student {
public string Name { get; set; }
public int Grade { get; set; }
}
class Program {
static void Main() {
Student[] students = new Student[3];
students[0] = new Student { Name = "John", Grade = 90 };
students[1] = new Student { Name = "Emma", Grade = 85 };
students[2] = new Student { Name = "Alex", Grade = 92 };
foreach (Student student in students) {
Console.WriteLine($"Student: {student.Name}, Grade: {student.Grade}");
}
}
}
In this code, we begin by defining a Student
class, which includes Name
and Grade
as its properties. This class provides the structure for each student object we intend to create.
We then proceed to the Main
method in the Program
class, which serves as the entry point of our application. Within this method, we declare an array of Student
objects named students
and initialize it to hold three Student
objects.
This is achieved through the expression new Student[3];
which sets the array size but doesn’t yet populate it with Student
objects.
Next, we individually create and assign Student
objects to each element of the array. For instance, students[0] = new Student { Name = "John", Grade = 90 };
creates a new Student
object with the name John
and a grade of 90
, and then assigns this object to the first element of the array.
We repeat this for the next two elements, each time creating a new Student
object with different property values.
The foreach
loop that follows is an elegant way to iterate through the students
array. Within this loop, we print out the name and grade of each student.
The Console.WriteLine
method formats and displays this information on the console.
Output:
Student: John, Grade: 90
Student: Emma, Grade: 85
Student: Alex, Grade: 92
Initializing an Array of Objects in C# by Declaring an Array with Elements
Initializing an array of objects directly with elements is a streamlined and efficient approach, especially when the elements to be stored are already known at the time of array creation. This method is particularly useful for setting up static or predefined data, allowing developers to write more concise and readable code.
To illustrate this concept, let’s consider an example where we have a Color
class and want to create an array of Color
objects. Each color object will represent a color with properties like Name
and HexValue
.
Example:
using System;
class Color {
public string Name { get; set; }
public string HexValue { get; set; }
}
class Program {
static void Main() {
Color[] colors = new Color[] { new Color { Name = "Red", HexValue = "#FF0000" },
new Color { Name = "Green", HexValue = "#00FF00" },
new Color { Name = "Blue", HexValue = "#0000FF" } };
foreach (Color color in colors) {
Console.WriteLine($"Color: {color.Name}, Hex: {color.HexValue}");
}
}
}
In this example, we start by defining a Color
class with two properties, Name
and HexValue
. This class serves as a blueprint for creating color objects, each representing a specific color.
Moving to the Main
method in the Program
class, which is the entry point of our application, we declare and initialize an array of Color
objects in a single line. The expression new Color[] { ... }
is used to create the array and populate it with Color
objects simultaneously.
Within the curly braces, we instantiate each Color
object with its respective properties. For instance, new Color { Name = "Red", HexValue = "#FF0000" }
creates a color object representing red.
After initializing the array, we use a foreach
loop to iterate over each Color
object in the colors
array. Inside the loop, we use Console.WriteLine
to output the name and hexadecimal value of each color.
This loop demonstrates how to access and use the properties of the objects stored in the array.
Output:
Color: Red, Hex: #FF0000
Color: Green, Hex: #00FF00
Color: Blue, Hex: #0000FF
Initializing Arrays in C# Through Extension Methods
Initializing arrays using the ToArray()
extension method represents a blend of simplicity and power, especially when dealing with collections or sequences. The ToArray()
method, part of the System.Linq
namespace, allows developers to seamlessly convert enumerable collections into arrays.
This approach is particularly beneficial when working with LINQ queries or when data needs to be transformed into a more rigid array structure for further processing.
To illustrate the usage of the ToArray()
extension method, let’s consider an example where we use a LINQ
query to filter and process a collection of objects before converting it into an array.
Example:
using System;
class Book {
public string Title { get; set; }
public string Author { get; set; }
}
class Program {
static void Main() {
// Initialize an array of Book objects with a size of 5
Book[] library = new Book[5];
// Example of populating the array with Book objects
for (int i = 0; i < library.Length; i++) {
library[i] = new Book(); // Assign a new Book object to each element
}
// Optional: Demonstrate that the array contains Book objects
foreach (Book book in library) {
Console.WriteLine($"Book object: {book}");
}
}
}
In this code, we begin by defining a Person
class with properties Name
and Age
. This class serves as the model for our data.
Next, in the Main
method of our Program
class, we create an array named people
and initialize it with three Person
objects. Each object represents a person with a name and an age.
We then use a LINQ query to filter this array. The Where
clause p => p.Age >= 30
selects only those Person
objects whose Age
is 30 or greater.
The ToArray()
method is then called on the result of this query. This method converts the filtered enumerable collection into an array, which we store in the adults
variable.
Following this, we use a foreach
loop to iterate over the adults
array, printing out the name and age of each person in the array. This loop demonstrates how we can access and use the properties of the objects stored in our newly created array.
Output:
Alice, 30
Charlie, 35
Conclusion
Through this comprehensive guide, we explored diverse strategies for initializing arrays of objects in C#. From defining arrays with a specific size to initializing them with predefined values and using extension methods for dynamic array creation, each method serves unique scenarios and requirements.
These techniques, foundational in C# programming, offer developers the flexibility and power to manipulate collections effectively. By understanding these various approaches, developers can choose the most suitable one for their specific context, leading to more efficient, readable, and maintainable code.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn