How to Get File Size in C#
-
Get File Size With the
FileInfo.Length
Property in C# -
Get File Size With the
FileStream
Class in C# -
Get File Size With the
File.ReadAllBytes
Method in C# - Conclusion
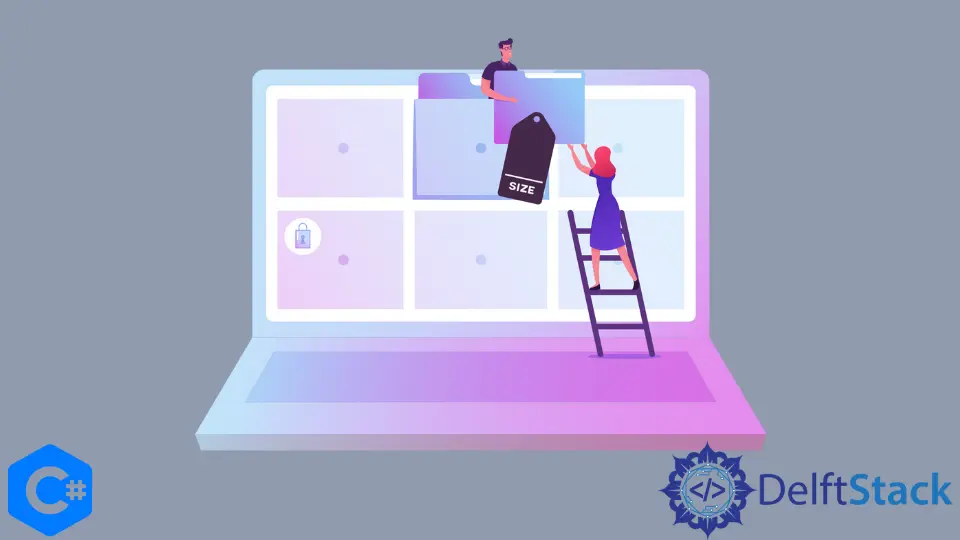
Determining the size of a file is a fundamental task in many software applications, ranging from simple utilities to complex data management systems. In C#, a language renowned for its versatility and efficiency, there are several methods to achieve this, each catering to different scenarios and requirements.
This article delves into three distinct approaches: using the FileInfo.Length
property, the FileStream
class, and the File.ReadAllBytes
method. Each of these techniques offers unique advantages, whether it’s the streamlined efficiency of FileInfo.Length
, the comprehensive functionality of FileStream
, or the straightforward simplicity of File.ReadAllBytes
.
By exploring these methods in detail, including their implementation, advantages, and potential drawbacks, this article aims to equip developers with the knowledge to choose the most suitable approach for their specific needs, enhancing their ability to manage and manipulate file data effectively in C#.
Get File Size With the FileInfo.Length
Property in C#
One common requirement is determining the size of a file, which can be crucial for applications like file upload validations, system monitoring, or data processing. In C#, one of the simplest and most efficient ways to obtain the file size is by using the FileInfo.Length
property.
The FileInfo
class provides methods for creating, opening, copying, deleting, and moving files in C#. The FileInfo.Length
property gets the size of a file in bytes.
using System;
using System.IO;
class Program {
static void Main() {
// Specify the file path
string filePath = "example.txt";
// Create a FileInfo object
FileInfo fileInfo = new FileInfo(filePath);
// Get the file size in bytes
long fileSizeInBytes = fileInfo.Length;
// Print the result
Console.WriteLine($"The size of the file '{filePath}' is: {fileSizeInBytes} bytes");
}
}
In our code analysis, we focus on the efficiency and simplicity of using FileInfo.Length
. Initially, we ensure the file path is accurately specified to avoid exceptions.
Upon creating a FileInfo
object for this file, we gain access to various properties and methods, including the Length
property, without needing to open the file itself. This approach is particularly efficient as it avoids file locking and the overhead of reading the file’s contents.
We retrieve the file size as a long
data type, catering to potentially large files. Lastly, we utilize Console.WriteLine
to display the file size, demonstrating a straightforward and resource-efficient method to acquire file size information suitable for quick checks and validations in various applications.
Output:
Get File Size With the FileStream
Class in C#
The FileStream
class in C#, part of the System.IO
namespace, provides a stream for a file, supporting both synchronous and asynchronous read and write operations. Using FileStream
, we can not only read and write to files but also retrieve file-related information, such as file size.
This method is especially beneficial when dealing with large files or when you need to perform operations on the file while also checking its size.
using System;
using System.IO;
class Program {
static void Main() {
// Specify the file path
string filePath = "example.txt";
// Open the file with FileStream
using (FileStream fileStream = new FileStream(filePath, FileMode.Open, FileAccess.Read)) {
// Get the file size in bytes
long fileSizeInBytes = fileStream.Length;
// Print the result
Console.WriteLine($"The size of the file '{filePath}' is: {fileSizeInBytes} bytes");
}
}
}
In our approach, we start by defining the file path. It’s essential to ensure this path is correct, as attempting to open a non-existent file with FileStream
will result in a FileNotFoundException
.
We then use the FileStream
constructor with appropriate parameters to open the file in read-only mode. The using
statement ensures that the file stream is properly disposed of after its use, which is crucial for resource management and preventing file locking issues.
Once the stream is open, we access its Length
property to get the file size in bytes. This method is particularly useful when the file is already being used in a stream for other operations, allowing us to get the size without additional overhead.
Output:
Get File Size With the File.ReadAllBytes
Method in C#
The primary use of File.ReadAllBytes
is to read the entire file content into memory. However, it can also serve to determine the file size by reading the file and then measuring the length of the resulting byte array.
This method is particularly useful in situations where you need both the file’s content and its size or in simpler applications where memory efficiency is not a primary concern.
using System;
using System.IO;
class Program {
static void Main() {
// Specify the file path
string filePath = "example.txt";
// Read all bytes from the file
byte[] fileContent = File.ReadAllBytes(filePath);
// Get the file size in bytes
long fileSizeInBytes = fileContent.Length;
// Print the result
Console.WriteLine($"The size of the file '{filePath}' is: {fileSizeInBytes} bytes");
}
}
In our example, we begin by specifying the file path, ensuring that the specified file exists to avoid a FileNotFoundException
. The method File.ReadAllBytes
is then used to read the entire file into a byte array.
While this method is very straightforward and works well for smaller files, it’s worth noting that it might not be the most memory-efficient approach for very large files, as it requires loading the entire file into memory. However, for moderate file sizes or scenarios where the file’s content is needed along with its size, this method is quite effective.
The resulting byte array’s Length
property gives us a simple way to determine the file size in bytes.
Output:
Conclusion
In this exploration of file size determination methods in C#, we have uncovered the strengths and limitations of three distinct approaches: FileInfo.Length
, FileStream
, and File.ReadAllBytes
.
Each method serves its purpose in different contexts. FileInfo.Length
stands out for its efficiency and ease of use, particularly when only file size information is needed without opening the file.
FileStream
offers a more robust solution, ideal for situations where file size needs to be determined in conjunction with other file operations, especially with larger files. Lastly, File.ReadAllBytes
provides a straightforward, albeit less memory-efficient, method for obtaining file size while simultaneously loading the file’s content into memory.
This comprehensive understanding of each method’s functionality and suitability enables developers to make informed decisions, optimizing file handling in their C# applications. By mastering these techniques, developers can ensure efficient and effective file management, a critical component in the landscape of modern software development.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn