Difference Between a Jagged Array and a Multi-Dimensional Array in C#
- What is a Jagged Array?
- What is a Multi-Dimensional Array?
- Key Differences Between Jagged Arrays and Multi-Dimensional Arrays
- Conclusion
- FAQ
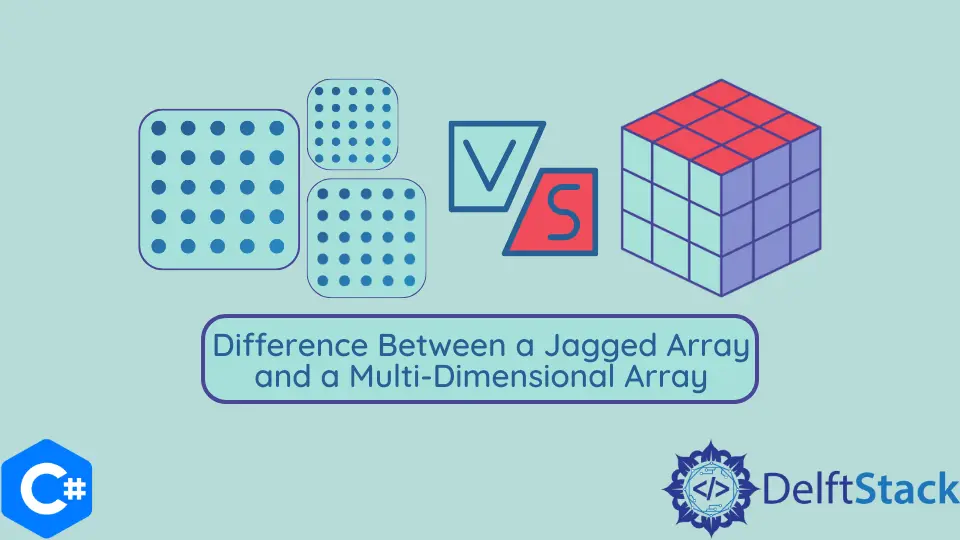
When delving into C# programming, understanding the nuances between jagged arrays and multi-dimensional arrays is crucial. These two types of arrays serve different purposes and have unique characteristics that can significantly impact how data is managed and accessed in your applications. A jagged array allows for arrays of varying sizes at each index, offering flexibility in data storage. In contrast, a multi-dimensional array requires uniform dimensions across all its indices, making it ideal for structured data.
In this article, we will explore these differences in detail, providing insights and examples to help you grasp the concepts effectively.
What is a Jagged Array?
A jagged array, often referred to as an “array of arrays,” is a collection of arrays where each element can be of different sizes. This flexibility allows for the storage of data that doesn’t fit into a traditional rectangular format. A jagged array is defined using a single set of square brackets, and each element can be initialized independently.
Here’s a simple example of how to declare and initialize a jagged array in C#:
int[][] jaggedArray = new int[3][];
jaggedArray[0] = new int[2] { 1, 2 };
jaggedArray[1] = new int[3] { 3, 4, 5 };
jaggedArray[2] = new int[1] { 6 };
In this example, jaggedArray
contains three arrays of varying lengths. The first array has two elements, the second has three, and the third has just one. This structure is particularly useful when dealing with datasets that do not conform to a fixed size, allowing for efficient memory usage.
Output:
jaggedArray[0]: 1, 2
jaggedArray[1]: 3, 4, 5
jaggedArray[2]: 6
The flexibility of jagged arrays makes them suitable for scenarios such as storing data from a database where each record might have a different number of fields or when dealing with lists of varying lengths, such as students in different classes.
What is a Multi-Dimensional Array?
Multi-dimensional arrays, on the other hand, are structured arrays where each dimension has a fixed size. These arrays are often visualized as tables or matrices, making them ideal for representing data that requires a consistent layout, such as images or grids.
To declare a multi-dimensional array in C#, you can use the following syntax:
int[,] multiDimensionalArray = new int[3, 3]
{
{ 1, 2, 3 },
{ 4, 5, 6 },
{ 7, 8, 9 }
};
In this example, multiDimensionalArray
is a 3x3 matrix, where each row and column contains an integer. Each element can be accessed using two indices, which represent the row and column positions.
Output:
multiDimensionalArray[0,0]: 1
multiDimensionalArray[1,1]: 5
multiDimensionalArray[2,2]: 9
Multi-dimensional arrays are particularly useful for mathematical computations, game development, and scenarios where data is naturally organized in a grid-like fashion. Their fixed structure ensures that all rows and columns maintain uniformity, which can simplify algorithms that rely on predictable data arrangements.
Key Differences Between Jagged Arrays and Multi-Dimensional Arrays
Understanding the key differences between jagged arrays and multi-dimensional arrays is essential for choosing the right structure for your data. Here’s a breakdown of the main distinctions:
- Structure: Jagged arrays consist of arrays of varying lengths, while multi-dimensional arrays have a uniform structure with fixed sizes for each dimension.
- Memory Usage: Jagged arrays can be more memory-efficient when dealing with datasets of varying sizes, as they allocate memory only for the elements they contain. In contrast, multi-dimensional arrays reserve memory for all elements, regardless of whether they are used.
- Accessing Elements: Elements in jagged arrays are accessed using a single index for each array, while multi-dimensional arrays require multiple indices to access elements based on their row and column positions.
- Flexibility vs. Predictability: Jagged arrays offer greater flexibility, which can be advantageous in certain scenarios. Multi-dimensional arrays provide predictability and consistency, making them easier to work with when data is structured.
Conclusion
In summary, the choice between using a jagged array or a multi-dimensional array in C# depends on your specific needs. Jagged arrays offer flexibility and are ideal for datasets with varying sizes, while multi-dimensional arrays provide a structured approach for uniform data. By understanding these differences, you can make informed decisions that enhance the efficiency and clarity of your code. Whether you’re developing applications, working with databases, or processing complex data, knowing when to use each type of array is a valuable skill in your programming toolkit.
FAQ
-
what is a jagged array in C#?
A jagged array is an array of arrays in C#, where each element can have different lengths, allowing for flexible data storage. -
what is a multi-dimensional array in C#?
A multi-dimensional array is a structured array in C# where each dimension has a fixed size, making it suitable for representing data in a grid-like format. -
when should I use a jagged array instead of a multi-dimensional array?
Use a jagged array when dealing with datasets of varying sizes, and opt for a multi-dimensional array when you need a consistent structure. -
can jagged arrays contain different data types?
No, jagged arrays in C# can only contain elements of the same data type, but each sub-array can be of different lengths. -
how do you access elements in a multi-dimensional array?
Elements in a multi-dimensional array are accessed using two indices, representing the row and column positions.
#. Understand how jagged arrays allow for varying sizes at each index while multi-dimensional arrays maintain uniform dimensions. This comprehensive guide provides clear examples and explanations to help you grasp these concepts effectively. Perfect for C# developers looking to enhance their programming skills.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn