How to Remove Duplicates From List in C#
-
Remove Duplicates From List With the
HashSet
Class inC#
-
Remove Duplicates From List With the LINQ Method in
C#
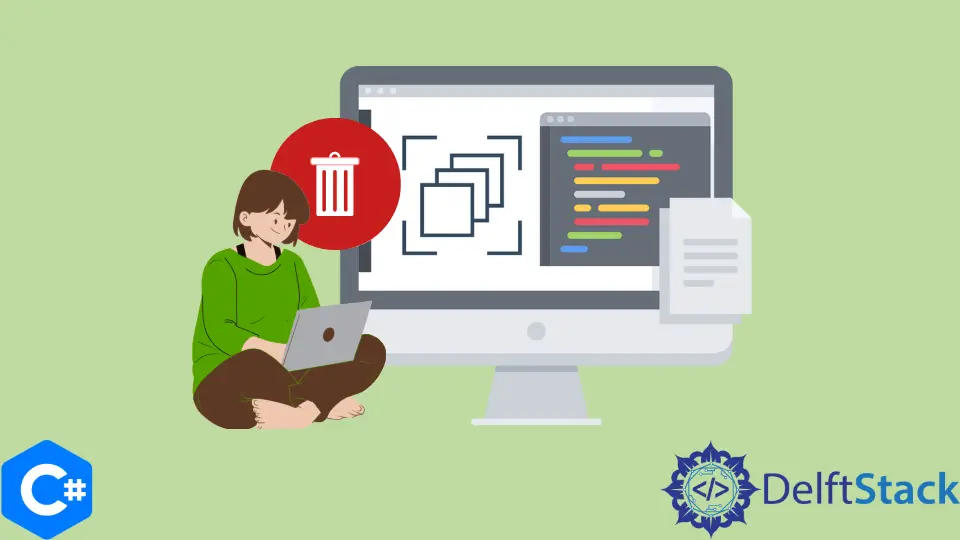
This tutorial will introduce the methods to remove duplicate elements from a list in C#.
Remove Duplicates From List With the HashSet
Class in C#
The HashSet
class is used to create a set in C#. A set is a well-known, unordered collection of distinct objects, which means that a set’s elements are unordered and do not repeat themselves. We can remove repeating elements from a list by storing the list into a HashSet
and then converting that HashSet
back to a list with the ToList()
function of LINQ. The following code example shows us how to remove duplicate elements from a list with the HashSet
class in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace remove_duplicates_from_list {
class Program {
static void displayList(List<int> list) {
foreach (var item in list) {
Console.WriteLine(item);
}
}
static void Main(string[] args) {
List<int> listWithDuplicates = new List<int> { 1, 2, 1, 2, 3, 4, 5 };
HashSet<int> hashWithoutDuplicates = new HashSet<int>(listWithDuplicates);
List<int> listWithoutDuplicates = hashWithoutDuplicates.ToList();
displayList(listWithoutDuplicates);
}
}
}
Output:
1
2
3
4
5
We declared and initialized the list of integers with repeating values listWithDuplicates
in the above code. We then converted the list to the set hasWithoutDuplicates
by passing the list in the constructor of the HashSet
class. We then converted the set back to the list of integers listWithoutDuplicates
with the LINQ ToList()
method. In the end, we displayed the elements inside the listWithoutDuplicates
list.
Remove Duplicates From List With the LINQ Method in C#
The LINQ integrates the query functionality in data structures in C#. The Distinct()
function of the LINQ is used to select unique values from a data structure in C#. The ToList()
function of the LINQ converts a collection of elements to a list in C#. We can use the Distinct()
function to select unique, non-repeating values from the list and then convert the selected values back to a list with the ToList()
function of LINQ. The following code example shows us how to remove duplicate values from a list with the LINQ method in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace remove_duplicates_from_list {
class Program {
static void displayList(List<int> list) {
foreach (var item in list) {
Console.WriteLine(item);
}
}
static void Main(string[] args) {
List<int> listWithDuplicates = new List<int> { 1, 2, 1, 2, 3, 4, 5 };
List<int> listWithoutDuplicates = listWithDuplicates.Distinct().ToList();
displayList(listWithoutDuplicates);
}
}
}
Output:
1
2
3
4
5
We declared and initialized the list of integers with repeating values listWithDuplicates
in the above code. We then selected unique values from this list with the Distinct()
function of LINQ. We then converted the selected values to the list of integers listWithoutDuplicates
with the LINQ ToList()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn