C#의 목록에서 중복 제거
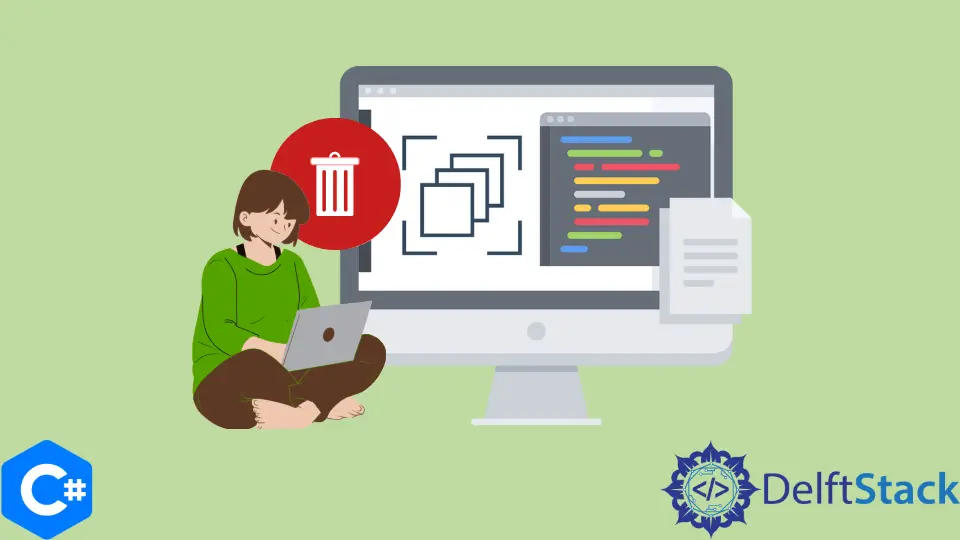
이 자습서에서는 C#의 목록에서 중복 요소를 제거하는 방법을 소개합니다.
C#에서HashSet
클래스를 사용하여 목록에서 중복 제거
HashSet
클래스는 C#에서 집합을 만드는 데 사용됩니다. 집합은 잘 알려진 개별 개체의 순서가 지정되지 않은 컬렉션으로 집합의 요소가 순서가 지정되지 않고 반복되지 않음을 의미합니다. 목록을HashSet
에 저장 한 다음 LINQ의ToList()
함수를 사용하여 해당HashSet
을 다시 목록으로 변환하여 목록에서 반복되는 요소를 제거 할 수 있습니다. 다음 코드 예제는 C#에서HashSet
클래스를 사용하여 목록에서 중복 요소를 제거하는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace remove_duplicates_from_list {
class Program {
static void displayList(List<int> list) {
foreach (var item in list) {
Console.WriteLine(item);
}
}
static void Main(string[] args) {
List<int> listWithDuplicates = new List<int> { 1, 2, 1, 2, 3, 4, 5 };
HashSet<int> hashWithoutDuplicates = new HashSet<int>(listWithDuplicates);
List<int> listWithoutDuplicates = hashWithoutDuplicates.ToList();
displayList(listWithoutDuplicates);
}
}
}
출력:
1
2
3
4
5
위 코드에서 반복되는 값이listWithDuplicates
인 정수 목록을 선언하고 초기화했습니다. 그런 다음HashSet
클래스의 생성자에 목록을 전달하여 목록을hasWithoutDuplicates
세트로 변환했습니다. 그런 다음 LINQ ToList()
메서드를 사용하여 집합을 다시 정수 목록listWithoutDuplicates
로 변환했습니다. 마지막으로listWithoutDuplicates
목록에 요소를 표시했습니다.
C#에서 LINQ 메서드를 사용하여 목록에서 중복 제거
LINQ는 C#의 데이터 구조에 쿼리 기능을 통합합니다. LINQ의 Distinct()
함수는 C#의 데이터 구조에서 고유 한 값을 선택하는 데 사용됩니다. LINQ의ToList()
함수는 요소 컬렉션을 C#의 목록으로 변환합니다. Distinct()
함수를 사용하여 목록에서 반복되지 않는 고유 한 값을 선택한 다음 LINQ의ToList()
함수를 사용하여 선택한 값을 다시 목록으로 변환 할 수 있습니다. 다음 코드 예제는 C#의 LINQ 메서드를 사용하여 목록에서 중복 값을 제거하는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace remove_duplicates_from_list {
class Program {
static void displayList(List<int> list) {
foreach (var item in list) {
Console.WriteLine(item);
}
}
static void Main(string[] args) {
List<int> listWithDuplicates = new List<int> { 1, 2, 1, 2, 3, 4, 5 };
List<int> listWithoutDuplicates = listWithDuplicates.Distinct().ToList();
displayList(listWithoutDuplicates);
}
}
}
출력:
1
2
3
4
5
위 코드에서 반복되는 값이listWithDuplicates
인 정수 목록을 선언하고 초기화했습니다. 그런 다음 LINQ의Distinct()
함수를 사용하여이 목록에서 고유 한 값을 선택했습니다. 그런 다음 LINQ ToList()
함수를 사용하여 선택한 값을listWithoutDuplicates
정수 목록으로 변환했습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn