How to Find the Greatest Common Divisor Using C#
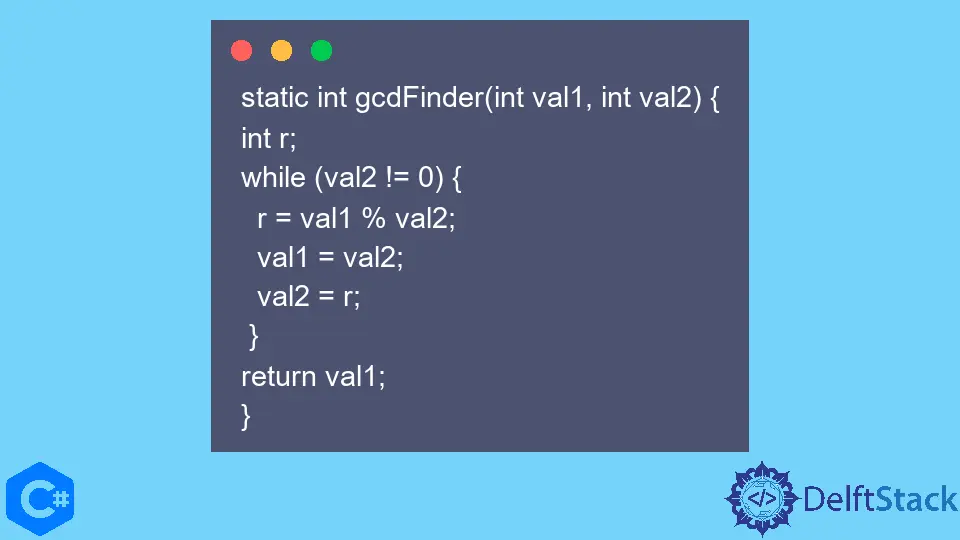
The topic of today’s tutorial will be how to use C# to find the greatest common divisor or GCD. Let’s first have a look at what the GCD is.
Find the Greatest Common Divisor Using C#
The greatest common divisor of two or more non-zero integers is the most significant positive integer that divides the numbers without producing a remainder. It is also known as the greatest common factor (GCF) or highest common factor (HCF).
Let’s use an example to find the greatest common divisor in C#.
-
Firstly, we’ll import the following libraries:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
-
Then, we’ll create a
gcdFinder()
function, which can get two parameters simultaneously. -
In this function, we’ll create a remainder variable
r
, value one calledval1
, and value two namedval2
. This function will find the most divisible integer, as shown below:static int gcdFinder(int val1, int val2) { int r; while (val2 != 0) { r = val1 % val2; val1 = val2; val2 = r; } return val1; }
-
In the
Main()
function, we’ll initialize two int variabless
andh
. -
Then, we’ll get input from the user and parse it to int:
int s, h; Console.Write("Please Enter 1st Number: "); s = int.Parse(Console.ReadLine()); Console.Write("Please Enter 2nd Number: "); h = int.Parse(Console.ReadLine());
-
Lastly, we’ll print the results by passing parameters to the
gcdFinder()
function:Console.WriteLine("\n GCD of {0} , {1} is {2}", s, h, gcdFinder(s, h)); Console.ReadLine();
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class ExamplebyShani {
static int gcdFinder(int val1, int val2) {
int r;
while (val2 != 0) {
r = val1 % val2;
val1 = val2;
val2 = r;
}
return val1;
}
static int Main(string[] args) {
int s, h;
Console.Write("Please Enter 1st Number: ");
s = int.Parse(Console.ReadLine());
Console.Write("Please Enter 2nd Number: ");
h = int.Parse(Console.ReadLine());
Console.WriteLine("\n GCD of {0}, {1} is {2}", s, h, gcdFinder(s, h));
Console.ReadLine();
return 0;
}
}
Output:
Please Enter 1st Number: 2
Please Enter 2nd Number: 3
GCD of 2, 3 is 1
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn