Standard Deviation in C#
- Standard Deviation With the Self-Defined Method in C#
- Standard Deviation With the Extension Function in C#
- Conclusion
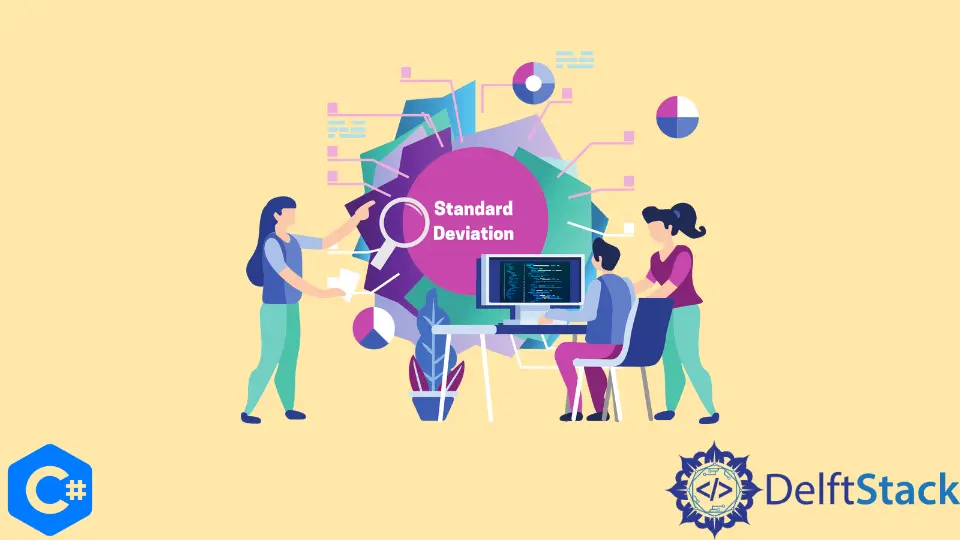
In statistics, the standard deviation stands as a vital measure, portraying the dispersion of data from its mean. Despite C# lacking a native method to compute standard deviation directly, we can still devise a logic to accomplish this essential statistical calculation.
This tutorial will introduce the methods to find the standard deviation of elements of a list in C#.
Standard Deviation With the Self-Defined Method in C#
Standard deviation is a statistical metric used to quantify the amount of variation or dispersion in a set of values. It gauges how spread out the values in a data set are, providing insights into the data’s distribution.
A low standard deviation implies that the values tend to be close to the mean, while a high standard deviation suggests that the values are more scattered.
To calculate the standard deviation for a given set of values, one typically follows these steps:
-
Calculate the mean (average) of the values.
-
Compute the squared difference between each value and the mean.
-
Sum up all the squared differences.
-
Divide the sum by the total number of values.
-
Take the square root of the result to obtain the standard deviation.
Formula:
Although there isn’t a pre-built method to calculate the standard deviation, we can create a custom logic to achieve this goal. The following code demonstrates how we can compute the standard deviation of elements within a list using a self-defined method:
using System;
using System.Collections.Generic;
using System.Linq;
namespace sd_list {
class Program {
static double standardDeviation(IEnumerable<double> sequence) {
double result = 0;
if (sequence.Any()) {
double average = sequence.Average();
double sum = sequence.Sum(d => Math.Pow(d - average, 2));
result = Math.Sqrt((sum) / sequence.Count());
}
return result;
}
static void Main(string[] args) {
List<double> intList = new List<double> { 1, 2, 3, 4, 5 };
double standard_deviation = standardDeviation(intList);
Console.WriteLine("Standard Deviation = {0}", standard_deviation);
}
}
}
Output:
Standard Deviation = 1.4142135623731
As we can see, the Program
class contains two methods: standardDeviation()
and Main()
.
The standardDeviation()
method calculates the standard deviation of the input sequence using the provided formula.
It first checks if the sequence is not empty (using sequence.Any()
). If it is not, the sequence calculates the standard deviation.
On the other hand, if the sequence is empty, it returns 0.
It calculates the mean of the values using sequence.Average()
and the sum of the squared differences between each element and the average using LINQ’s Sum
method.
Finally, it calculates the standard deviation using the formula: sqrt(sum / count)
, where sum
is the sum of squared differences, and count
is the number of elements in the sequence.
The Main()
method is the entry point of the program. It creates a List<double>
named intList
with some sample double values and then calls the standardDeviation()
method to calculate the standard deviation.
Finally, it prints the calculated standard deviation to the console.
Standard Deviation With the Extension Function in C#
Additionally, we can encapsulate the standard deviation calculation logic into an extension function to enhance ease of use and code modularity. The subsequent code exhibits this approach, showcasing how the previous example’s logic can be transformed into an extension method in C#:
using System;
using System.Collections.Generic;
using System.Linq;
namespace sd_list {
public static class ExtensionClass {
public static double standardDeviation(this IEnumerable<double> sequence) {
double average = sequence.Average();
double sum = sequence.Sum(d => Math.Pow(d - average, 2));
return Math.Sqrt((sum) / sequence.Count());
}
}
class Program {
static void Main(string[] args) {
List<double> intList = new List<double> { 1, 2, 3, 4, 5 };
double standard_deviation = intList.standardDeviation();
Console.WriteLine("Standard Deviation = {0}", standard_deviation);
}
}
}
Output:
Standard Deviation = 1.4142135623731
In the example code, ExtensionClass
is a static class that defines an extension method standardDeviation()
for IEnumerable<double>
objects.
The standardDeviation()
method calculates the standard deviation of the input sequence using the formula for standard deviation. It calculates the average using sequence.Average()
and then computes the sum of the squared differences between each element and the average.
Finally, it takes the square root of the sum divided by the count of elements in the sequence.
As we can see, we also have the Program
class containing the Main()
method, which is the entry point of the program. In the Main()
method, a List<double>
named intList
is created with some sample double values.
The standardDeviation()
extension method is invoked on intList
to calculate the standard deviation. The calculated standard deviation is then printed to the console.
Conclusion
Understanding and calculating the standard deviation is fundamental in statistical analysis. In C#, while there is no built-in method for this calculation, we’ve demonstrated how to create a custom logic and an extension method to compute the standard deviation of elements within a list.
The approaches discussed above enable programmers to effectively calculate the standard deviation, a critical statistical metric, in their C# applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn