How to Convert Decimal to Double in C#
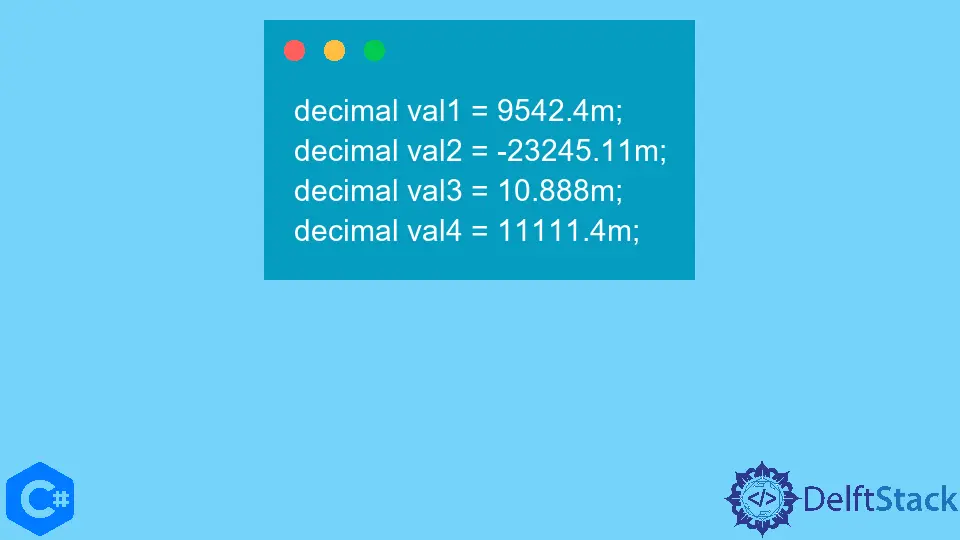
This tutorial will teach us how to convert decimal numbers to double in the C# language.
Use the Decimal.ToDouble()
Method to Convert Decimal to Double in C#
The value of the provided decimal is converted into the equivalent double-precision floating-point number using Decimal.ToDouble()
. Because a double-precision floating-point integer contains fewer significant digits than a decimal, this method has the potential to cause round-off mistakes.
It converts a specified decimal
value to a double-precision, floating-point number using the Decimal.ToDouble()
method.
Syntax:
public static double ToDouble(decimal deci);
deci
is the value indecimal
notation that we want to convert to a double.- This method will return a number with
double
precision and a floating point format that is the same as the expressiondec
.
Let’s look at an example for us to understand.
-
To begin, we have to use the following libraries.
using System; using System.Collections.Generic; using System.IO; using System.Linq;
-
Within the
Main()
class, we will construct fourdecimal
variables and initialize them with some values.decimal val1 = 9542.4m; decimal val2 = -23245.11m; decimal val3 = 10.888m; decimal val4 = 11111.4m;
-
Let’s use the
Decimal.ToDouble()
method on thedecimal
numbers and store the results on variousdouble
variables,val1converted
,val2converted
,val3converted
andval4converted
.double val1converted = Decimal.ToDouble(val1); double val2converted = Decimal.ToDouble(val2); double val3converted = Decimal.ToDouble(val3); double val4converted = Decimal.ToDouble(val4);
-
Lastly, we will output the resulting
double
values to the console.Console.WriteLine(val1converted); Console.WriteLine(val2converted); Console.WriteLine(val3converted); Console.WriteLine(val4converted);
Complete Source Code:
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
class decimal2doublebyZeeshan {
static void Main() {
decimal val1 = 9542.4m;
decimal val2 = -23245.11m;
decimal val3 = 10.888m;
decimal val4 = 11111.4m;
double val1converted = Decimal.ToDouble(val1);
double val2converted = Decimal.ToDouble(val2);
double val3converted = Decimal.ToDouble(val3);
double val4converted = Decimal.ToDouble(val4);
Console.WriteLine(val1converted);
Console.WriteLine(val2converted);
Console.WriteLine(val3converted);
Console.WriteLine(val4converted);
}
}
Output:
9542.4
-23245.11
10.888
11111.4
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn