How to Convert Double to Decimal in C#
-
Use the
Convert.ToDecimal(Double)
Method to Convert Double to Decimal in C# -
Use the Casting
(decimal)
Method to Convert Double to Decimal in C# -
Use the
Constructor
Method to Convert Double to Decimal in C# - Conclusion
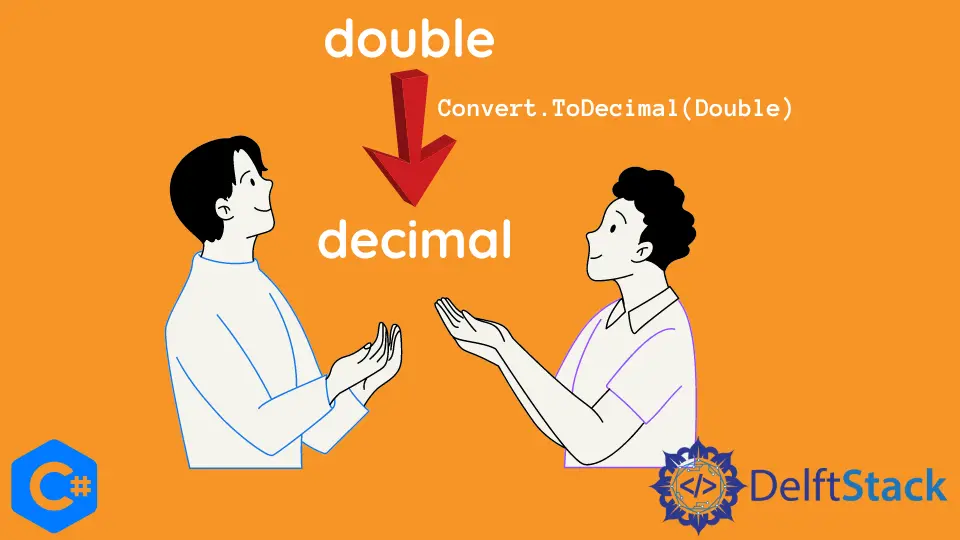
Accurate numeric representation is pivotal in programming, particularly in scenarios involving financial or scientific calculations. When precision matters, the choice between the double
and decimal
data types becomes crucial.
This article explores three methods to convert a double
to a decimal
in C#: using the Convert.ToDecimal
method, casting, and the decimal
constructor. Each method addresses precision needs in different scenarios, offering developers versatile tools to handle numeric conversions.
Use the Convert.ToDecimal(Double)
Method to Convert Double to Decimal in C#
We will use the Convert.ToDecimal(Double)
method found in the System.Convert
namespace, which is responsible for converting from double to decimal. This method accepts a value of type double as its argument and returns a value of type decimal.
This function can only return decimal numbers, and those values can have no more than 15 significant digits. If the value parameter contains more than 15 significant digits, it is rounded to the closest decimal before being used.
Consider a scenario where a monetary value is stored as a double
, and the objective is to convert it to a decimal
for precise calculations.
using System;
class Program {
static void Main() {
// Original monetary amount stored as a double
double originalAmount = 1234.56;
// Using Convert.ToDecimal for the conversion
decimal convertedAmount = Convert.ToDecimal(originalAmount);
// Displaying the results with data types
Console.WriteLine("Original Amount (double): " + originalAmount);
Console.WriteLine("Converted Amount (decimal): " + convertedAmount.GetType() + ": " +
convertedAmount);
}
}
We start by declaring a double
variable named originalAmount
with an initial value of 1234.56
.
The Convert.ToDecimal
method is then applied to originalAmount
, and the result is stored in the variable convertedAmount
. This single line encapsulates the entire conversion process.
To provide clarity, we use Console.WriteLine statements to display both the original amount and the converted amount. Additionally, we include the GetType
method to showcase the change in data type from double
to decimal
.
Output:
Original Amount (double): 1234.56
Converted Amount (decimal): System.Decimal: 1234.56
Use the Casting (decimal)
Method to Convert Double to Decimal in C#
When dealing with numeric values in C#, developers often encounter situations where precision is a top priority. The process of casting a double
to a decimal
is a precise conversion method that provides explicit control over the type transition.
Casting, denoted by (decimal)
, involves explicitly specifying the desired target type (in this case, decimal
). It signals to the compiler that the developer is intentionally converting a double
to a decimal
.
Let’s consider a scenario where a numeric value is stored as a double
, and the goal is to convert it to a decimal
using casting.
using System;
class Program {
static void Main() {
// Original numeric value stored as a double
double originalValue = 987.654;
// Using casting to convert double to decimal
decimal convertedValue = (decimal)originalValue;
// Displaying the results with data types
Console.WriteLine($"Original Value (double): {originalValue}");
Console.WriteLine($"Converted Value (decimal): {convertedValue.GetType()}: {convertedValue}");
}
}
We start by defining a double
variable named originalValue
with an initial value of 987.654
. The goal is to convert this double
to a decimal
using casting.
The casting operation is performed with the syntax (decimal)originalValue
. This explicit casting instructs the compiler to convert the double
value to a decimal
.
The result is stored in the variable convertedValue
.
To provide clarity, we use the Console.WriteLine
statements to display both the original and converted values. Additionally, we include the GetType
method to showcase the data type of the converted value, affirming the successful transition from double
to decimal
.
Output:
Original Value (double): 987.654
Converted Value (decimal): System.Decimal: 987.654
Use the Constructor
Method to Convert Double to Decimal in C#
Another approach, equally effective, is to utilize the decimal
constructor to achieve the conversion. In this section, we’ll explore the concept of using a constructor to convert a double
to a decimal
in C#, highlighting its importance and practical implementation.
Consider a scenario where a numeric value is stored as a double
, and the goal is to convert it to a decimal
using a constructor.
using System;
class Program {
static void Main() {
// Original numeric value stored as a double
double originalValue = 789.012;
// Using decimal constructor for the conversion
decimal convertedValue = new decimal(originalValue);
// Displaying the results with data types
Console.WriteLine($"Original Value (double): {originalValue}");
Console.WriteLine($"Converted Value (decimal): {convertedValue.GetType()}: {convertedValue}");
}
}
We begin by defining a double
variable named originalValue
with an initial value of 789.012
. Our objective is to convert this double
value to a decimal
using the decimal
constructor.
The key step involves creating a new decimal
object by invoking the decimal
constructor and passing the originalValue
as an argument. This constructor is specifically designed to handle the conversion from a double
to a decimal
.
To provide clarity, we use the Console.WriteLine
statements to display both the original and converted values. Additionally, we include the GetType
method to showcase the data type of the converted value, affirming the successful transition from double
to decimal
.
Output:
Original Value (double): 789.012
Converted Value (decimal): System.Decimal: 789.012
Conclusion
In conclusion, these three methods for converting double
to decimal
in C# provide developers with diverse approaches catering to specific precision requirements. The Convert.ToDecimal
method excels in simplicity, casting offers explicit control, and the decimal
constructor provides an alternative constructor-based approach.
Understanding these methods empowers developers to choose the most suitable technique based on the specific needs of their applications, ensuring precision and accuracy in numeric operations.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn