How to Get Double Value by Dividing Two Integers in C#
-
Division With Integer Data Type in
C#
-
Use the
Double
Keyword to Get a Double Value by Dividing Two Integers inC#
-
Use
decimal.ToDouble
to Get a Double Value by Dividing Two Integers inC#
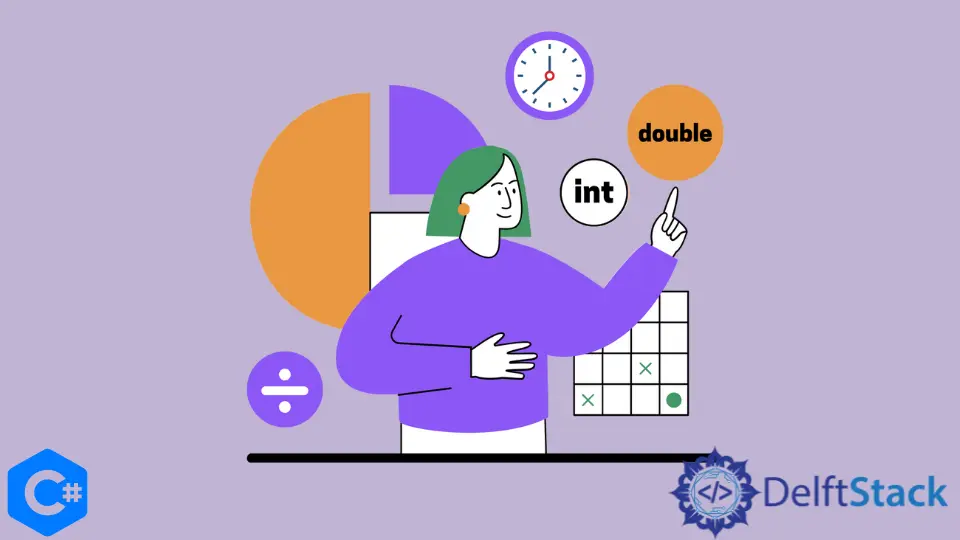
This article will discuss dividing two integers and getting results as a double data type.
Division With Integer Data Type in C#
Applying the divide operation on the integer data type only gives us the result of complete division and ignores all values after the decimal point.
The below image shows the division of values of integer data type. For example, we divide two numbers, 30 and 7, with integer data types.
When we try to divide both values with integer data types, the divide operation gets the first values before decimal places. Like in our case, when we divided 30 by 7, the operation gives us only 4 as the quotient of 30/7
because it ignores all remaining values after decimal places.
Use the Double
Keyword to Get a Double Value by Dividing Two Integers in C#
To overcome this problem and include the values of the result after the decimal point, we need to convert the data type from integers to double. With integers types, it’s directly impossible to get the complete answer with decimal places.
So when we convert our integer value into double using only (double)
, it will include all values after the decimal point, which will give us the complete result of division.
Use decimal.ToDouble
to Get a Double Value by Dividing Two Integers in C#
When we converted n1
and n2
to double using the double
keyword at its start and applied the divide operation between operands, n1
and n2
, we got a complete answer of division 30 by 7 as 4.28571428571429.
In the code below, we used decimal.ToDouble
to convert an integer value to double before applying the divide operation. When we use all these values as double data types, it will be helpful to get good results.
Below is the complete code portion used in this article.
using System;
public class Division {
public static void Main(string[] args) {
int n1, n2;
n1 = 30;
n2 = 7;
int result = n1 / n2;
Console.WriteLine("result of normal integer division:" + result + "\n");
double res = decimal.ToDouble(n1) / decimal.ToDouble(n2);
Console.WriteLine("result by converting to double through decimal.ToDouble() is " + res + "\n");
res = (double)n1 / (double)n2;
Console.WriteLine("result by converting to double through (double) is " + res + "\n");
Console.Read();
}
}
Whenever we need to apply division, our preference should be to check the data type of operands. If data types are integers, then it may cause a calculation error.
We can say it is a logical error, and we will not get an accurate answer, so it’s risky with scientific calculations that may cause any severe problem. It is already cleared to avoid issues related to sensitive calculations; we need to make sure about its data type.
It should be double to be with accurate results. The main data type will remain integer; we use the double data type to get accurate results.