C# を使用して最大公約数を求める
Muhammad Zeeshan
2023年10月12日
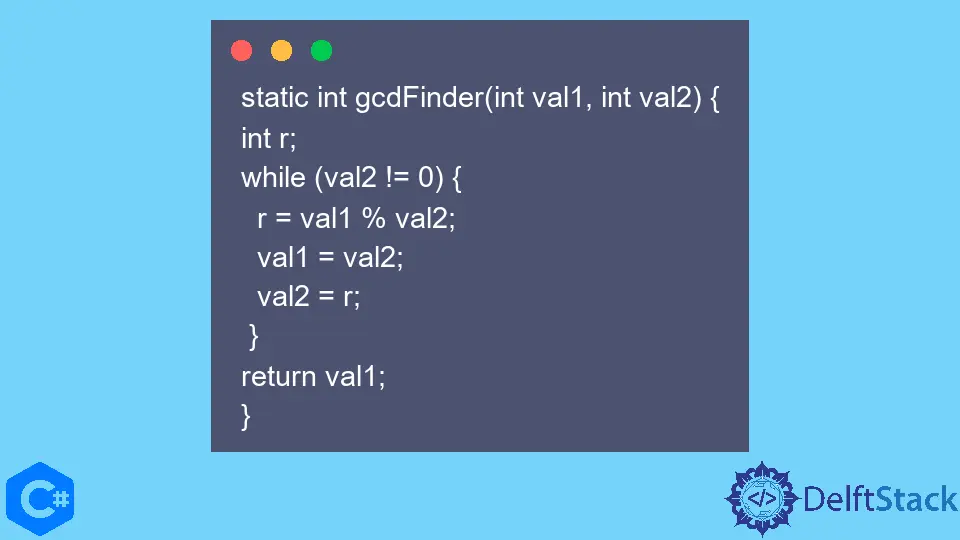
今日のチュートリアルのトピックは、C# を使用して最大公約数または GCD を見つける方法です。 まず、GCD とは何かを見てみましょう。
C#
を使用して最大公約数を見つける
2つ以上のゼロ以外の整数の最大公約数は、剰余を生成せずに数値を除算する最も重要な正の整数です。 これは、最大公約数 (GCF) または最大公約数 (HCF) としても知られています。
例を使用して、C# で最大公約数を見つけてみましょう。
-
まず、次のライブラリをインポートします。
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
-
次に、2つのパラメーターを同時に取得できる
gcdFinder()
関数を作成します。 -
この関数では、剰余変数
r
、値 1 はval1
、値 2 はval2
を作成します。 この関数は、以下に示すように、最も割り切れる整数を見つけます。static int gcdFinder(int val1, int val2) { int r; while (val2 != 0) { r = val1 % val2; val1 = val2; val2 = r; } return val1; }
-
Main()
関数では、2つの int 変数s
とh
を初期化します。 -
次に、ユーザーから入力を取得し、それを int に解析します。
int s, h; Console.Write("Please Enter 1st Number: "); s = int.Parse(Console.ReadLine()); Console.Write("Please Enter 2nd Number: "); h = int.Parse(Console.ReadLine());
-
最後に、パラメーターを
gcdFinder()
関数に渡して結果を出力します。Console.WriteLine("\n GCD of {0} , {1} is {2}", s, h, gcdFinder(s, h)); Console.ReadLine();
完全なソース コード:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class ExamplebyShani {
static int gcdFinder(int val1, int val2) {
int r;
while (val2 != 0) {
r = val1 % val2;
val1 = val2;
val2 = r;
}
return val1;
}
static int Main(string[] args) {
int s, h;
Console.Write("Please Enter 1st Number: ");
s = int.Parse(Console.ReadLine());
Console.Write("Please Enter 2nd Number: ");
h = int.Parse(Console.ReadLine());
Console.WriteLine("\n GCD of {0}, {1} is {2}", s, h, gcdFinder(s, h));
Console.ReadLine();
return 0;
}
}
出力:
Please Enter 1st Number: 2
Please Enter 2nd Number: 3
GCD of 2, 3 is 1
著者: Muhammad Zeeshan
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn