How to Convert Image to Byte Array in C#
- Overview of Byte Array
-
Use
Memory Stream
to Convert Image to Byte Array inC#
-
Use
ImageConverter
to Convert Image to Byte Array inC#
-
Convert Byte Array to Image in
C#
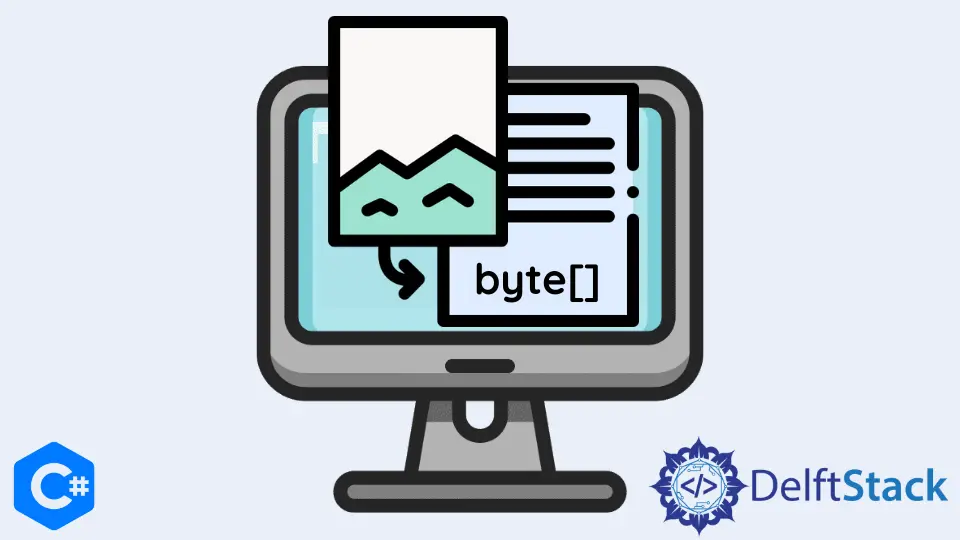
This tutorial will teach us how to convert an image into a byte array using the Memory Stream
and the Image Converter
classes. In addition, we will better understand the process of converting a C# array of bytes into an image.
Overview of Byte Array
A collection of binary data is often stored in a format known as a byte array, which is an array of bytes. For instance, the information regarding each pixel of a picture is stored inside the byte array that constitutes the image.
In the byte array, we can save the binary representation of the contents of any file. In the same way, we initialize the regular array with the bytes; we can also initialize the byte array with the bytes.
Use Memory Stream
to Convert Image to Byte Array in C#
The .NET Framework provides support for a wide variety of image formats. The Picture object has a save method that allows developers to save an image to a file in any of these formats.
Code:
public byte[] imgToByteArray(Image img) {
using (MemoryStream mStream = new MemoryStream()) {
img.Save(mStream, img.RawFormat);
return mStream.ToArray();
}
}
In the above example, we write a function called imgToByteArray
that accepts an img
input of type Image
as its argument. Inside this method’s using
line, we create an instance of the MemoryStream()
class.
Then we apply the Save()
function to the MemoryStream
object during this instance while an image format is specified. Now that the item is already stored in memory, the ToArray()
method available on the MemoryStream
object may quickly and efficiently transform it into a byte array.
Use ImageConverter
to Convert Image to Byte Array in C#
Code:
public static byte[] imgToByteConverter(Image inImg) {
ImageConverter imgCon = new ImageConverter();
return (byte[])imgCon.ConvertTo(inImg, typeof(byte[]));
}
We created a function called imgToByteConverter()
. It takes an input named inImg
and has the type Image
as its parameter.
Inside this method, we create an instance of the ImageConverter()
class. After converting the image that was sent to it using the .ConvertTo()
method, this function will return the bytes that were converted to their original format.
Convert Byte Array to Image in C#
Converting an image into a byte array is one that we are familiar with. Now that the byte array has been loaded, we will look at the function that will transform it into an image so that we may utilize the image in our code moving forward.
Code:
public Image byteArrayToImage(byte[] byteArrayIn) {
using (MemoryStream mStream = new MemoryStream(byteArrayIn)) {
return Image.FromStream(mStream);
}
}
A function called byteArrayToImage()
is created. It takes an input named byteArrayIn
and has the type byte[]
as its parameter.
This method contains a using
statement that initializes an instance of the MemoryStream
class by providing it with the bytes stored in the byteArrayIn
variable. The bytes are then transformed into an image with the assistance of the .FromStream()
method, which calls for an argument referred to as mStream
and returns it.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn