Array of Strings in C#
-
an Array of String in
C#
-
Declaration of String Array in
C#
-
Initialization and Implementation of String Array in
C#
- Take Values From the User Input and Store It in an Array of String
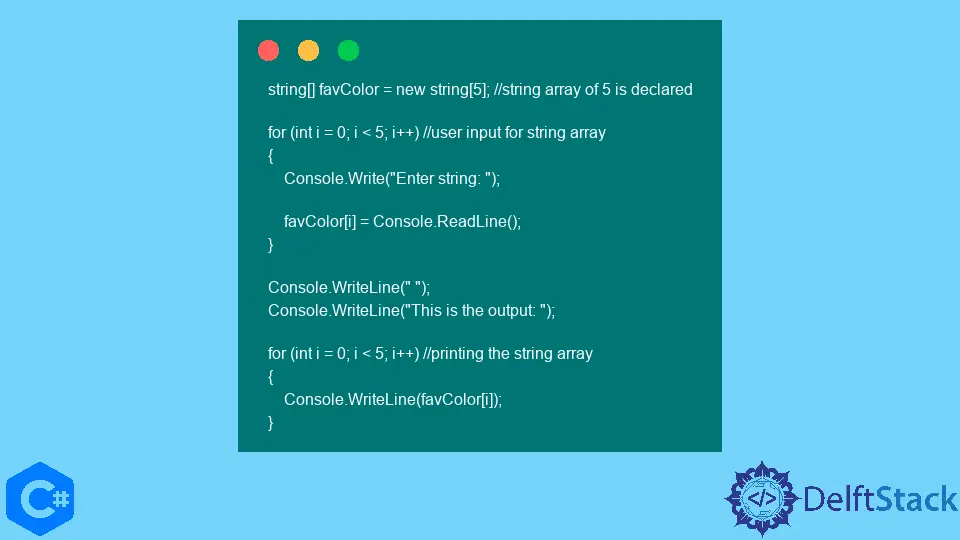
This guide will explain the array of strings in C#.
How to declare an array of strings? How do you initialize it and implement it?
How can we store multiple values inside the array of strings from the user input? All these will be answered in this article.
an Array of String in C#
An array is a way to store multiple elements at a time. In an array, we specify the type before declaring.
So when we store string values in an array, it is called an array of strings.
Declaration of String Array in C#
We must add a string
type at the beginning to declare the array as a string. We must also write the square brackets []
and the string
keyword.
An example of how to declare a string array is below.
string[] favColor = {}; // declare only
A string array of favColor
has been declared. As of now, our array is null as there is no initialization.
There is no value inside this array. Let’s see how we can fill it up.
Initialization and Implementation of String Array in C#
To initialize, we will add the elements in an array during compile time. We can initialize an array by adding elements one by one with the help of an index, or we can initialize it all together.
If an array is declared before, we have to use the new
keyword to initialize the array of a data type. We can also access a value through an index.
Note that, since we are using string values, we have to use double quotation ""
.
string[] favColor = {};
favColor = new string[3] { "Blue", "Green", "White" }; // initialize only
favColor[0] = "red"; // it will override blue
Console.WriteLine("my favorite color " + favColor[0]);
Output:
my favorite color red
We can also initialize and declare an array at the same time. It’s common knowledge that we use a loop to print the whole array, just like in the code below.
string[] favColor = { "blue", "green", "white" };
for (int i = 0; i < favColor.Length; i++) // print favColor using for loop
{
Console.WriteLine(favColor[i]);
}
Output:
blue
green
white
Take Values From the User Input and Store It in an Array of String
After declaration, we store the values in the array through initialization. Now, we can do this in two ways.
One is at compile time. This method was already discussed, so now we will look into the other way, which is at runtime.
The compiler collects the data from the user. So, instead of adding data during compile time, we will add it during runtime.
As we know, data is added in an array to avoid declaring each value as an individual. Hence in runtime, we add our values using a for
loop so that we don’t have to add them one by one.
This example will further explain how to store value from user input.
string[] favColor = new string[5]; // string array of 5 is declared
for (int i = 0; i < 5; i++) // user input for string array
{
Console.Write("Enter string: ");
favColor[i] = Console.ReadLine();
}
Console.WriteLine(" ");
Console.WriteLine("This is the output: ");
for (int i = 0; i < 5; i++) // printing the string array
{
Console.WriteLine(favColor[i]);
}
Output:
Enter string: red
Enter string: blue
Enter string: green
Enter string: red
Enter string: orange
This is the output:
red
blue
green
red
orange
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn