How to Convert Object to Int in C#
-
Convert Object to Int With the
(int)
TypeCasting inC#
-
Convert Object to Int With the
int.Parse()
Function inC#
-
Convert Object to Int With the
Convert.ToInt32()
Function inC#
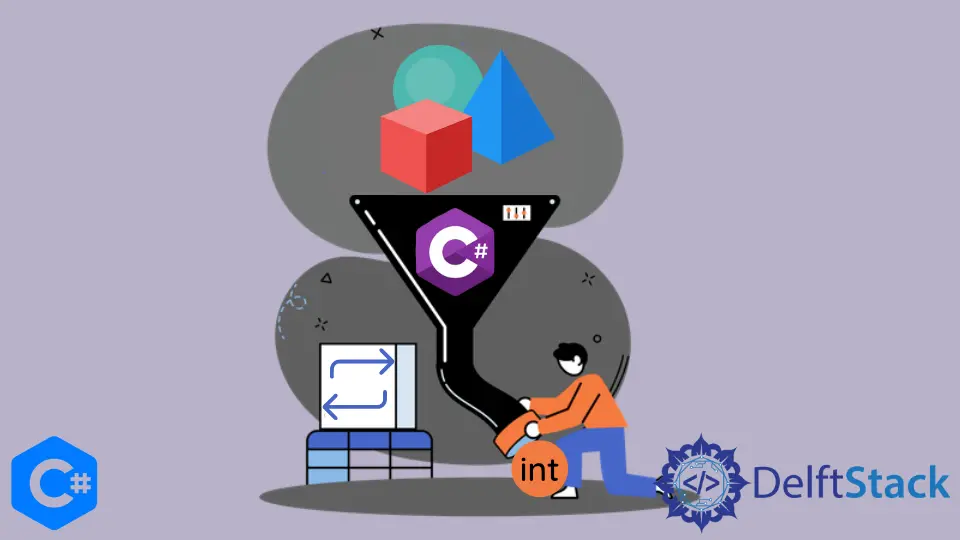
This tutorial will discuss the methods for converting a data type to integer data type in C#.
Convert Object to Int With the (int)
TypeCasting in C#
Type casting is a technique to cast the data from one data type to another similar data type in C#. The (int)
expression is used to typecast a data type to integer data type in C#. The (int)
expression can only be used with numeric data types like float, double, and decimal. We cannot use the (int)
expression with any non-numeric data types. The following code example shows us how we can convert from a numeric data type to an integer data type with the (int)
expression in C#.
using System;
namespace convert_to_int {
class Program {
static void Main(string[] args) {
float f = 1.01f;
int i = (int)f;
Console.WriteLine(i);
}
}
}
Output:
1
In the above code, we initialized the float variable f
and converted it to integer i
with the (int)
typecasting expression in C#.
Convert Object to Int With the int.Parse()
Function in C#
The int.Parse()
function converts a string to the integer data type in C#. It takes a string variable containing integer equivalent data as an argument and returns an integer value. The int.Parse()
function gives an exception if the string variable’s value is not equivalent to the integer data type. The following code example shows us how to convert the string data type to the integer data type with the int.Parse()
function in C#.
using System;
namespace convert_to_int {
class Program {
static void Main(string[] args) {
string s = "1";
int i = int.Parse(s);
Console.WriteLine(i);
}
}
}
Output:
1
In the above code, we initialized the string variable s
with integer equivalent value 1
and converted it to the integer variable i
with the int.Parse(s)
function in C#.
Convert Object to Int With the Convert.ToInt32()
Function in C#
The Convert
class provides functionality for conversion between different basic data types in C#. The Convert.ToInt32()
function converts any data type to an integer data type. The Convert.ToInt32()
function takes a data type as an argument and returns the 32-bit integer equivalent value. The following code example shows us how we can convert any data type to an integer data type with the Convert.ToInt32()
function in C#.
using System;
namespace convert_to_int {
class Program {
static void Main(string[] args) {
string s = "1";
int i = Convert.ToInt32(s);
Console.WriteLine(i);
}
}
}
Output:
1
In the above code, we initialized the string variable s
with integer equivalent value 1
and converted it to the integer variable i
with the Convert.ToInt32(s)
function in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn