How to Convert Long to Int in C#
-
Use Type Casting Operator to Convert
long
toint
in C# -
Use the
Convert.ToInt32()
Method to Convertlong
toint
in C# -
Use Checked and Unchecked Keywords to Convert
long
toint
in C# - Conclusion
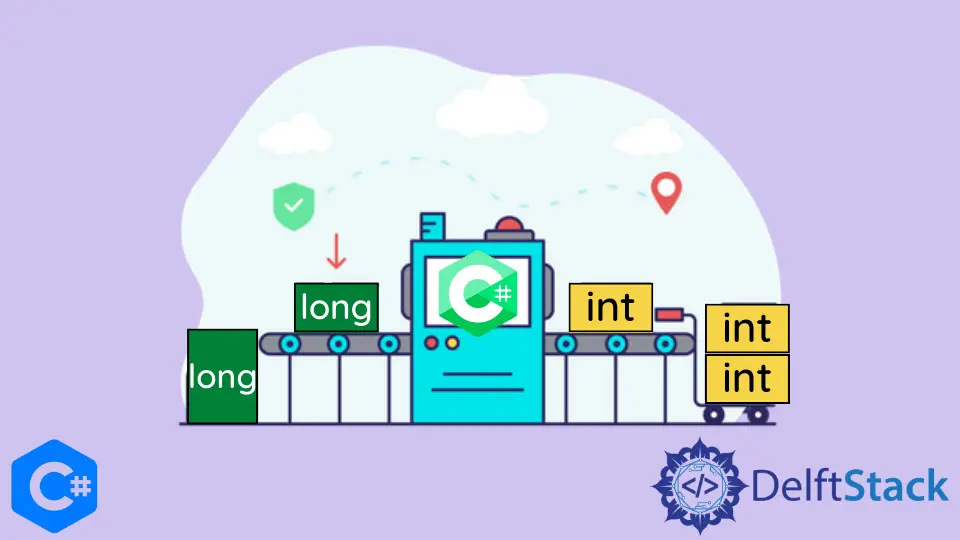
In the realm of numeric data manipulation in C#, the need to convert between different numeric types often arises.
One such conversion, and a topic of great significance, is converting from long
to int
. This operation is crucial when dealing with large numerical values, as the long
type offers a wider range than int
but at the cost of increased memory consumption.
In C#, a long
is a 64-bit integer data type capable of holding larger values than an int
, which is a 32-bit integer. Converting from a long
to an int
can result in a loss of precision if the value of the long
exceeds the range of an int
.
This tutorial will discuss the methods of converting a long
variable to an int
variable in C#, including handling data loss and overflow.
Use Type Casting Operator to Convert long
to int
in C#
In C#, you can use the type casting operator to explicitly convert one data type to another. Since the long
data type takes more bytes than the int
data type, we must use the explicit type casting method to convert the long
data type to the int
data type.
Syntax:
(target_type) expression
Here, target_type
is the data type you want to convert to, and expression
is the value you want to convert. Keep in mind that not all conversions are allowed, and it’s essential to consider the potential loss of data when performing conversions.
Example 1:
To convert a long
to an int
, you can use the type casting operator as follows:
long myLong = 10000000000;
int myInt = (int)myLong;
In this example, we have a long
variable named myLong
with the value 10000000000
. We then use the type casting operator (int)
to explicitly convert it to an int
, which may result in a loss of precision.
Example 2:
using System;
namespace convert_long_to_int {
class Program {
static void Main(string[] args) {
long l = 12345;
int i = (int)l;
Console.WriteLine("long = {0}", l);
Console.WriteLine("Integer = {0}", i);
}
}
}
Output:
long = 12345
Integer = 12345
In the above code, we used the explicit type casting operator (int)
to convert the long
variable l
to the int
variable i
. It will give you an incorrect result if l
is larger than 231 - 1.
Handling Potential Data Loss
It’s crucial to be aware that converting a long
to an int
can result in data loss if the value of the long
exceeds the range of an int
. To mitigate this, you may want to perform range checking before the conversion.
long myLong = 10000000000;
if (myLong >= int.MinValue && myLong <= int.MaxValue) {
int myInt = (int)myLong;
Console.WriteLine("Conversion successful: " + myInt);
} else {
Console.WriteLine("Value is out of range for int.");
}
In this example, we first check if the value of myLong
falls within the range of an int
. If it does, we will proceed with the conversion. Otherwise, we handle the case where the value is too large for an int
.
Output:
Value is out of range for int.
Dealing With Overflow
It’s important to note that converting a long
to an int
can result in overflow if the value of the long
is too large to fit into an int
. In such cases, the result will be truncated, and you may not get the expected outcome.
long myLong = 10000000000;
int myInt = (int)myLong;
Console.WriteLine("Result: " + myInt);
In this example, since 10000000000
is beyond the range of an int
, the result will be 1410065408
due to overflow.
Output:
Result: 1410065408
Use the Convert.ToInt32()
Method to Convert long
to int
in C#
The Convert
class converts between different base data types in C#. Since integer and long
are base data types, we can convert from long
to integer data type with the Convert.ToInt32()
method in C#.
The Convert.ToInt32()
method is used to convert any base data type to a 32-bit integer data type.
Syntax:
int Convert.ToInt32(long value)
Here, value
is the long
you want to convert to an int
.
The following code examples show how to convert a long
variable to an integer variable with the Convert.ToInt32()
method in C#.
Example 1:
To convert a long
to an int
using Convert.ToInt32()
, you simply pass the long
value as an argument to the method. The method will then perform the conversion and return the result as an int
.
long myLong = 10000000000;
int myInt = Convert.ToInt32(myLong);
In this example, we have a long
variable named myLong
with the value 10000000000
. We use the Convert.ToInt32()
method to convert it to an int
, which may result in a loss of precision.
Example 2:
using System;
namespace convert_long_to_int {
class Program {
static void Main(string[] args) {
long l = 12345;
int i = Convert.ToInt32(l);
Console.WriteLine("long = {0}", l);
Console.WriteLine("Integer = {0}", i);
}
}
}
Output:
long = 12345
Integer = 12345
In the above code, we converted the long
variable l
to the integer variable i
with the Convert.ToInt32()
function in C#. This method gives an exception if the value of the long
variable is too big for the integer variable to handle.
Handling Data Loss and Overflow
It’s crucial to be aware that converting a long
to an int
can result in data loss if the value of the long exceeds the range of an int
. To mitigate this, you may want to perform range checking before the conversion.
long myLong = 10000000000;
if (myLong >= int.MinValue && myLong <= int.MaxValue) {
int myInt = Convert.ToInt32(myLong);
Console.WriteLine("Conversion successful: " + myInt);
} else {
Console.WriteLine("Value is out of range for int.");
}
In this example, we first check if the value of myLong
falls within the range of an int
. If it does, we will proceed with the conversion. Otherwise, we handle the case where the value is too large for an int
.
Output:
Value is out of range for int.
Additionally, it’s important to note that converting a long
to an int
can result in overflow if the value of the long
is too large to fit into an int
. In such cases, the result will be truncated, and you may not get the expected outcome.
long myLong = 10000000000;
int myInt = Convert.ToInt32(myLong);
Console.WriteLine("Result: " + myInt);
The code above involves the conversion of a long
variable (myLong
) with the value 10000000000
to an int
using the Convert.ToInt32()
method. Given that long
can hold larger values than int
, this operation might result in data loss or truncation.
The resulting int
value is then printed to the console. However, it’s important to note that if myLong
exceeds the maximum value that an int
can hold, an overflow will occur, potentially leading to unexpected results.
Output:
System.OverflowException: 'Value was either too large or too small for an Int32.'
Use Checked and Unchecked Keywords to Convert long
to int
in C#
In C#, arithmetic operations are checked for overflow by default. This means that if an operation results in a value outside the range of the target data type, an exception will be thrown.
The checked
keyword is used to explicitly enable overflow checking for arithmetic operations, while the unchecked
keyword is used to disable it.
Converting long
to int
Using the checked
Keyword
To convert a long
to an int
using the checked
keyword, you enclose the code block in a checked
context. Within this context, arithmetic operations are checked for overflow, and if an overflow occurs, an exception is thrown.
checked {
long myLong = 10000000000;
int myInt = (int)myLong; // Potential overflow
Console.WriteLine("Result: " + myInt);
}
In this example, the code block is enclosed in a checked
context. The long
variable myLong
is assigned a value of 10000000000
.
We then attempt to convert it to an int
, which may result in a potential overflow. If an overflow occurs, an exception will be thrown.
Output:
System.OverflowException: 'Arithmetic operation resulted in an overflow.'
Converting long
to int
Using the unchecked
Keyword
Conversely, if you want to explicitly disable overflow checking, you can use the unchecked
keyword. This allows potentially unsafe operations to be performed without throwing exceptions.
unchecked {
long myLong = 10000000000;
int myInt = (int)myLong; // Potential overflow
Console.WriteLine("Result: " + myInt);
}
In this example, the code block is enclosed in an unchecked
context. The long
variable myLong
is assigned a value of 10000000000
.
We then attempt to convert it to an int
, which may result in a potential overflow. With unchecked
, no exception will be thrown in the event of an overflow.
Output:
Result: 1410065408
Handling Overflow Situations
When using the checked
keyword, you can catch the OverflowException
to handle potential overflow situations.
try {
checked {
long myLong = 10000000000;
int myInt = (int)myLong; // Potential overflow
Console.WriteLine("Result: " + myInt);
}
} catch (OverflowException ex) {
Console.WriteLine("OverflowException caught: " + ex.Message);
}
In this example, we enclose the code block in a try
block and catch the OverflowException
that may occur. If an overflow occurs during the conversion, the exception will be caught, and you can handle it accordingly.
Output:
OverflowException caught: Arithmetic operation resulted in an overflow.
Conclusion
Converting between different numeric types is a common task in C# programming, and handling conversions from long
to int
requires careful consideration of potential data loss and overflow scenarios.
Using the type casting operator allows for direct conversion from long
to int
, but it’s important to be aware of the potential loss of precision, especially if the value of the long
exceeds the range of an int
. Range checking before the conversion is recommended to avoid unintended consequences.
The Convert.ToInt32()
method provides a convenient way to convert a long
to an int
in C#. It handles the conversion and also throws an exception if the value is too large for an int
to handle.
Additionally, the checked
and unchecked
keywords offer explicit control over overflow checking during arithmetic operations. Using checked
ensures that potential overflows are caught and handled, while unchecked
allows potentially unsafe operations without throwing exceptions.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn