How to Check if List Is Empty in C#
-
Use the
List.Count
Property to Check Whether a List Is Empty in C# -
Use the
List.Any()
Function to Check Whether a List Is Empty in C# -
Use the
is null
for Reference Types to Check Whether a List Is Empty in C# - Conclusion
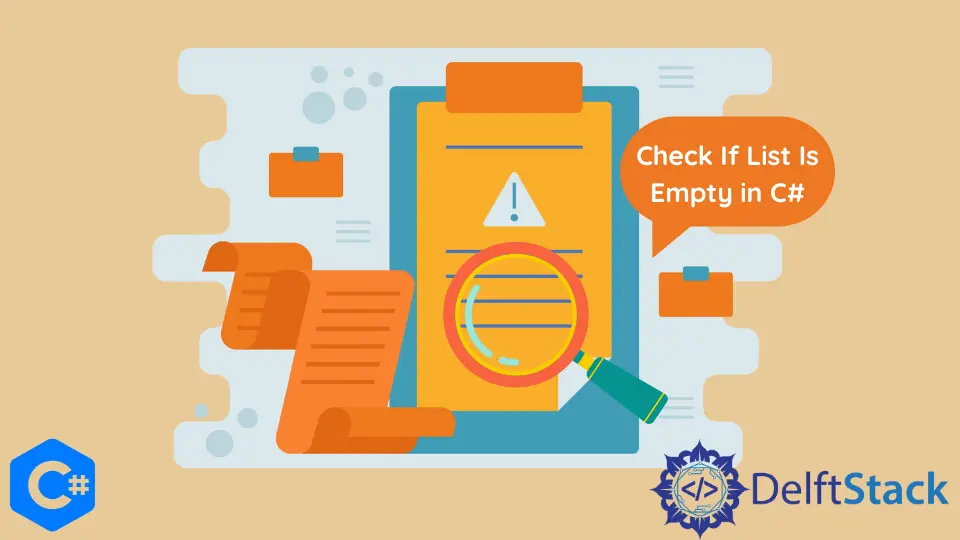
This tutorial will introduce methods to check if a list is empty or not in C#. Three commonly used techniques are introduced, each providing a distinct approach to ascertain the absence of elements in a list.
Use the List.Count
Property to Check Whether a List Is Empty in C#
In C#, the List.Count
property is a way to determine the number of elements in a List<T>
, where T
is the type of elements in the list. When you use List.Count
on an instance of the List<T>
class, it returns an integer representing the number of elements in the list.
To check whether a list is empty, you can compare the result of List.Count
to zero. If the count is zero, it indicates that the list has no elements and is, therefore, considered empty.
Basic Syntax:
int count = yourList.Count;
There isn’t a traditional parameter in this syntax. It’s simply assigning the count of elements in yourList
to the variable count
.
If you have a method or function that takes a list as a parameter, the usage of that parameter would be in the method signature and body, not in this particular line of code.
Code Example:
using System;
using System.Collections.Generic;
using System.Linq;
namespace check_empty_list {
class Program {
static void Main(string[] args) {
List<string> emptyList = new List<string>();
if (emptyList.Count == 0) {
Console.WriteLine("List is Empty");
} else {
Console.WriteLine("Not Empty");
}
}
}
}
Output:
List is Empty
In the code, we declared a list and instantiated it as an empty list. The subsequent conditional statement uses the Count
property of the list to determine its size.
If the count is equal to zero, indicating an absence of elements, the program outputs "List is Empty"
using Console.WriteLine
. In this case, since the list is empty, the output of the program will be "List is Empty"
.
The else
block is not executed in this scenario. This concise program effectively demonstrates how to use the List.Count
property to check for an empty list in C#.
Use the List.Any()
Function to Check Whether a List Is Empty in C#
The List.Any()
function is a convenient method provided by LINQ (Language Integrated Query) that checks whether any elements in a collection satisfy a specified condition. When used without parameters, List.Any()
returns true
if the list contains any elements and false
if the list is empty.
Basic Syntax:
bool anyElements = yourList.Any();
The List.Any()
method typically doesn’t take explicit parameters in its basic form. When used without parameters, it checks whether the list contains any elements.
Code Example:
using System;
using System.Collections.Generic;
using System.Linq;
namespace check_empty_list {
class Program {
static void Main(string[] args) {
List<string> emptyList = new List<string>();
if (emptyList.Any()) {
Console.WriteLine("Not Empty");
} else {
Console.WriteLine("List is Empty");
}
}
}
}
Output:
List is Empty
In the code, the list is declared and instantiated as an empty list. The subsequent conditional statement checks if there are any elements in the list by invoking the Any()
method.
If the list is not empty, the program outputs "Not Empty"
using Console.WriteLine
. However, in this case, since the list is empty, the condition returns false, and the program executes the else
block, resulting in the output "List is Empty"
.
This program effectively demonstrates how to utilize the List.Any()
method to ascertain the emptiness of a list in C#.
Use the is null
for Reference Types to Check Whether a List Is Empty in C#
The is null
check is a simple and direct way to determine whether a reference type, such as a list, is null
. A reference type variable can either point to an object or have a null
value, indicating the absence of an object.
Code Example:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main(string[] args) {
List<string> emptyList = new List<string>();
if (emptyList is null || emptyList.Count == 0) {
Console.WriteLine("List is Empty");
} else {
Console.WriteLine("List Not Empty");
}
}
}
Output:
List is Empty
In this code, we declare and instantiate the list as empty. The subsequent if
statement employs the is null
check alongside the Count
property to determine if the list is either uninitialized or has zero elements.
If either condition is true, indicating an empty list, we output "List is Empty"
using Console.WriteLine
. In this specific case, as the list is indeed empty, the program will print "List is Empty"
.
The else
block is not executed in this scenario. This concise program effectively demonstrates how to use the is null
check in conjunction with the Count
property to check for an empty list in C#.
Conclusion
These methods provide us with options to efficiently check for an empty list in C#. Depending on the context and coding preferences, we can choose between the List.Count
property, the List.Any()
function, or the is null
check.
The provided code examples serve as practical illustrations, enabling developers to integrate these techniques seamlessly into our projects.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn