在 C# 中检查列表是否为空
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp List
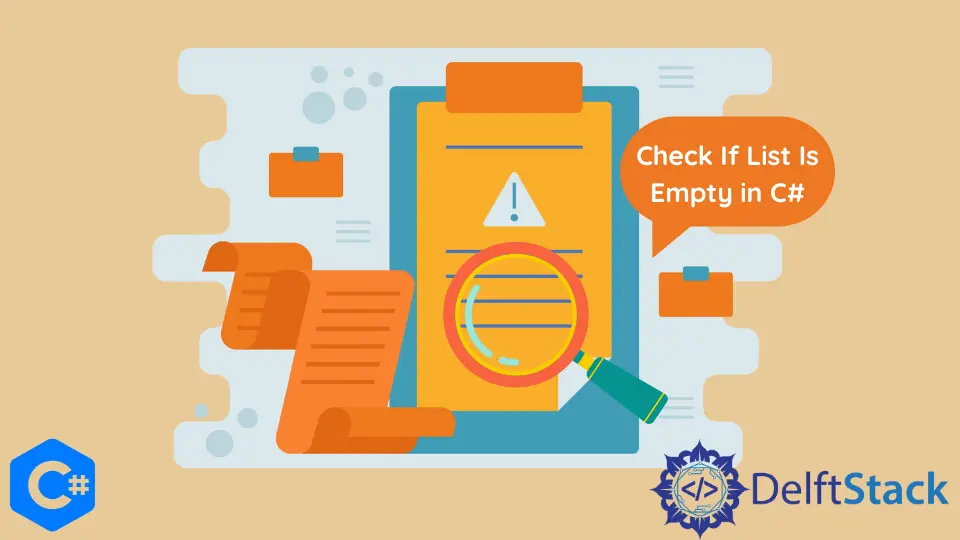
本教程将介绍在 C# 中检查列表是否为空的方法。
使用 C# 中的 List.Count
属性检查列表是否为空
List.Count
属性获得 C# 列表中的元素。如果列表为空,则 List.Count
为 0
。以下代码示例向我们展示了如何使用 C# 中的 List.Count
属性检查列表是否为空。
using System;
using System.Collections.Generic;
using System.Linq;
namespace check_empty_list {
class Program {
static void Main(string[] args) {
List<string> emptyList = new List<string>();
if (emptyList.Count == 0) {
Console.WriteLine("List is Empty");
} else {
Console.WriteLine("Not Empty");
}
}
}
}
输出:
List is Empty
在上面的代码中,我们初始化了一个空字符串 emptyList
列表,并使用 C# 中的 List.Count
属性检查该列表是否为空。
使用 C# 中的 List.Any()
函数检查列表是否为空
List.Any()
函数也可以用于检查该列表在 C# 中是否为空。List.Any()
函数的返回类型为布尔值。如果列表中有一个元素,则 List.Any()
函数将返回 true
;否则返回 false
。请参见下面的示例代码。
using System;
using System.Collections.Generic;
using System.Linq;
namespace check_empty_list {
class Program {
static void Main(string[] args) {
List<string> emptyList = new List<string>();
if (emptyList.Any()) {
Console.WriteLine("Not Empty");
} else {
Console.WriteLine("List is Empty");
}
}
}
}
输出:
List is Empty
在上面的代码中,我们初始化了一个空字符串 emptyList
列表,并使用 C# 中的 List.Any()
函数检查该列表是否为空。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn