How to Calculate Sum of Array in C++
-
Use the
std::accumulate
Function to Calculate the Sum of Array Elements in C++ -
Use
std::partial_sum
Function to Calculate Partial Sums of Subarrays in C++
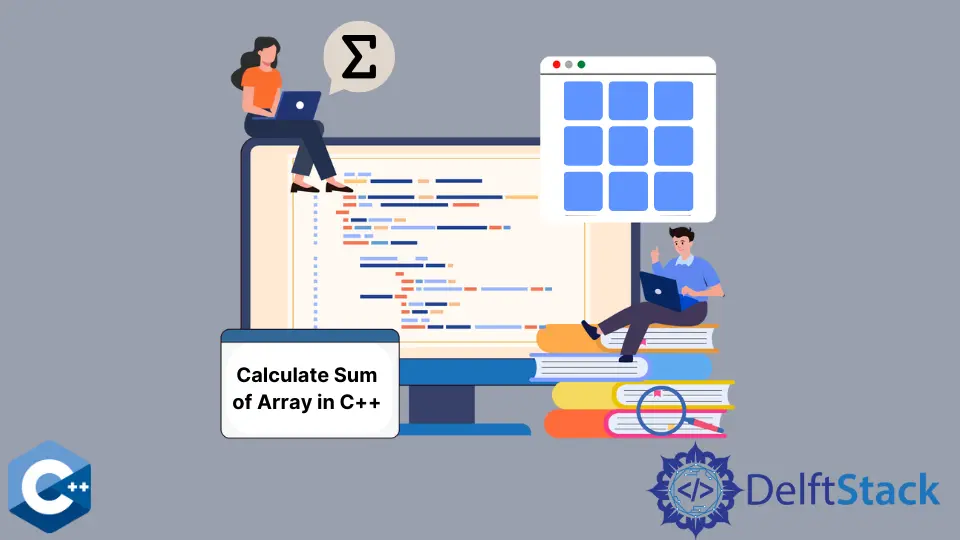
This article will explain several methods of how to calculate a sum of array elements in C++.
Use the std::accumulate
Function to Calculate the Sum of Array Elements in C++
std::accumulate
is part of numeric functions included in the STL library under the header file <numeric>
. std::accumulate
is a generic function that works on the elements in the given range, which is specified by the first two arguments represented by the corresponding iterators. The third argument is used to pass the initial value of the sum, which can be the object’s literal value. In this case, we demonstrate a dynamic array of integers stored in the std::vector
container. Notice that std::accumulate
can optionally take the fourth argument of a binary function object type to substitute the addition operation. The second call to std::accumulate
in the next example code demonstrates such a scenario, with the only difference that the multiplication is used as a binary operation.
#include <iostream>
#include <numeric>
#include <vector>
using std::accumulate;
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr1 = {1, 12, 13, 10, 11};
printVector(arr1);
int arr1_sum = accumulate(arr1.begin(), arr1.end(), 0);
cout << "sum of arr1 = " << arr1_sum << endl;
int arr1_product =
accumulate(arr1.begin(), arr1.end(), 1, std::multiplies<>());
cout << "product of arr1 = " << arr1_product << endl;
return EXIT_SUCCESS;
}
Output:
1, 12, 13, 10, 11,
sum of arr1 = 47
product of arr1 = 17160
Use std::partial_sum
Function to Calculate Partial Sums of Subarrays in C++
Another useful numeric function provided by the same header file is a std::partial_sum
, which sums the sub-ranges in the given range. The function offers a similar interface as the previous one, except that the third argument should be the starting iterator of the range where the calculated numbers will be stored. In this case, we overwrite the existing vector elements with the summed results. Notice that the custom binary operation can also be specified with std::partial_sum
to change the default option - addition.
#include <iostream>
#include <numeric>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr1 = {1, 12, 13, 10, 11};
printVector(arr1);
std::partial_sum(arr1.begin(), arr1.end(), arr1.begin());
printVector(arr1);
std::partial_sum(arr1.begin(), arr1.end(), arr1.begin(), std::multiplies());
printVector(arr1);
return EXIT_SUCCESS;
}
Output:
1, 13, 26, 36, 47,
1, 13, 338, 12168, 571896,
Alternatively, these functions can also be utilized to conduct bitwise operations between the elements in the given range. For example, the following code snippet XORs the vector
elements and stores the result values in the same range.
#include <iostream>
#include <numeric>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr1 = {1, 12, 13, 10, 11};
printVector(arr1);
std::partial_sum(arr1.begin(), arr1.end(), arr1.begin(), std::bit_xor());
printVector(arr1);
return EXIT_SUCCESS;
}
Output:
1, 13, 0, 10, 1,
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook