C++에서 배열의 합계 계산
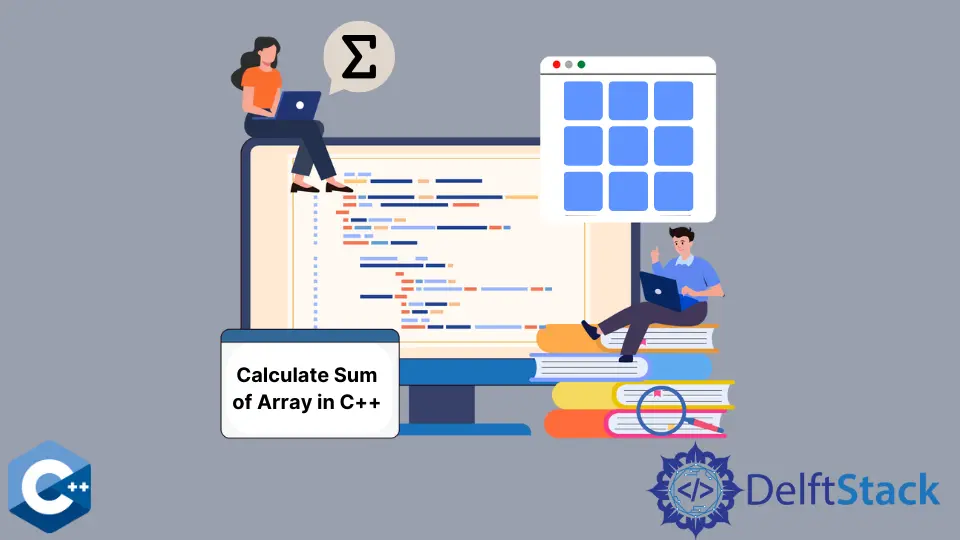
이 기사에서는 C++에서 배열 요소의 합계를 계산하는 방법에 대한 몇 가지 방법을 설명합니다.
std::accumulate
함수를 사용하여 C++에서 배열 요소의 합계를 계산합니다
std::accumulate
는 헤더 파일<numeric>
아래 STL 라이브러리에 포함 된 숫자 함수의 일부입니다. std::accumulate
는 주어진 범위의 요소에 대해 작동하는 일반 함수로, 해당 반복자가 나타내는 처음 두 개의 인수로 지정됩니다. 세 번째 인수는 개체의 리터럴 값이 될 수있는 합계의 초기 값을 전달하는 데 사용됩니다. 이 경우 std::vector
컨테이너에 저장된 정수의 동적 배열을 보여줍니다. std::accumulate
는 선택적으로 이진 함수 객체 유형의 네 번째 인수를 사용하여 더하기 연산을 대체 할 수 있습니다. 다음 예제 코드에서std::accumulate
에 대한 두 번째 호출은 곱셈이 이진 연산으로 사용된다는 유일한 차이점을 제외하고 이러한 시나리오를 보여줍니다.
#include <iostream>
#include <numeric>
#include <vector>
using std::accumulate;
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr1 = {1, 12, 13, 10, 11};
printVector(arr1);
int arr1_sum = accumulate(arr1.begin(), arr1.end(), 0);
cout << "sum of arr1 = " << arr1_sum << endl;
int arr1_product =
accumulate(arr1.begin(), arr1.end(), 1, std::multiplies<>());
cout << "product of arr1 = " << arr1_product << endl;
return EXIT_SUCCESS;
}
출력:
1, 12, 13, 10, 11,
sum of arr1 = 47
product of arr1 = 17160
std::partial_sum
함수를 사용하여 C++에서 부분 배열의 부분 합계 계산
동일한 헤더 파일에서 제공하는 또 다른 유용한 숫자 함수는std::partial_sum
으로, 주어진 범위의 하위 범위를 합산합니다. 이 함수는 세 번째 인수가 계산 된 숫자가 저장 될 범위의 시작 반복자 여야한다는 점을 제외하고 이전 것과 유사한 인터페이스를 제공합니다. 이 경우 합산 된 결과로 기존 벡터 요소를 덮어 씁니다. 사용자 지정 바이너리 연산은std::partial_sum
으로 지정하여 기본 옵션 인 추가를 변경할 수도 있습니다.
#include <iostream>
#include <numeric>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr1 = {1, 12, 13, 10, 11};
printVector(arr1);
std::partial_sum(arr1.begin(), arr1.end(), arr1.begin());
printVector(arr1);
std::partial_sum(arr1.begin(), arr1.end(), arr1.begin(), std::multiplies());
printVector(arr1);
return EXIT_SUCCESS;
}
출력:
1, 13, 26, 36, 47,
1, 13, 338, 12168, 571896,
대안으로, 이러한 함수는 주어진 범위의 요소들 사이에서 비트 연산을 수행하는 데 사용될 수도 있습니다. 예를 들어, 다음 코드 스 니펫은vector
요소를 XOR하고 결과 값을 동일한 범위에 저장합니다.
#include <iostream>
#include <numeric>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr1 = {1, 12, 13, 10, 11};
printVector(arr1);
std::partial_sum(arr1.begin(), arr1.end(), arr1.begin(), std::bit_xor());
printVector(arr1);
return EXIT_SUCCESS;
}
출력:
1, 13, 0, 10, 1,
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook