How to Parse a Comma Separated String Sequence in C++
-
Use
std::string::find
andstd::string::erase
Functions to Parse Comma Separated String Sequence in C++ -
Use
std::getline
Function to Parse Comma Separated String Sequence
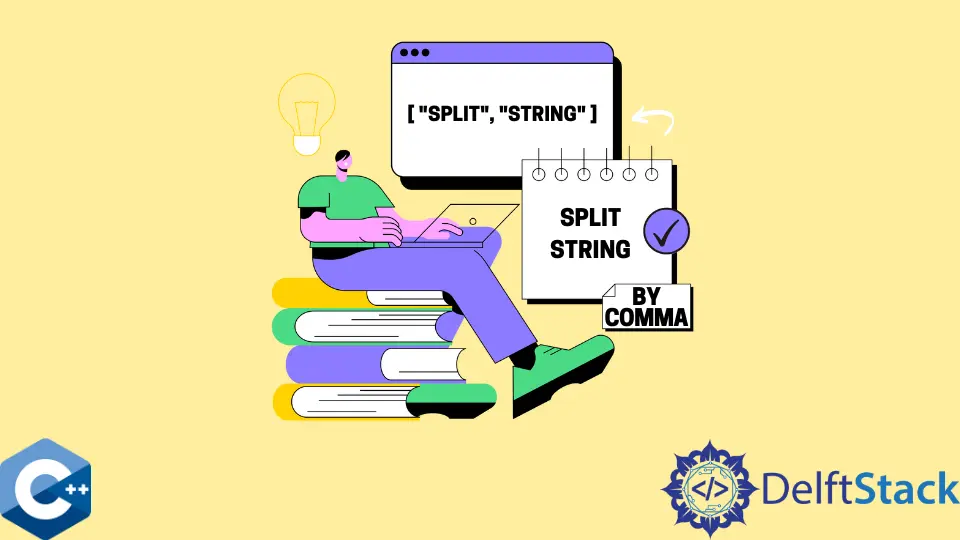
This article will demonstrate multiple methods about how to parse a comma-separated string sequence in C++.
Use std::string::find
and std::string::erase
Functions to Parse Comma Separated String Sequence in C++
The std::find
method is a built-in function of the std::string
class, and it can be utilized to find the position of the given substring. It takes a single argument in this case which denotes the substring to be found. Any multi-character string variable can be initialized to be passed as an argument. Although, in the following example, we declare a single comma containing string
. Since the find
method returns the position of the substring and string::npos
when the substring is not found, we can put the comparison expression in a while
loop that’s gonna execute until the expression evaluates true.
We also store the parsed string on each iteration in the vector
container. Then, the erase
method is called to delete the characters before and including the first found comma delimiter in order to continue processing the same string
object in the next iteration. Finally, we output the stored elements in the vector
to the cout
stream.
#include <algorithm>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "1,2,3,4,5,6,7,8,9,10";
string delimiter = ",";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delimiter)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delimiter.length());
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
Output:
1
2
3
4
5
6
7
8
9
Use std::getline
Function to Parse Comma Separated String Sequence
The previous solution misses extracting the last number inf the comma-separated sequence. So it might be better to use the following method using the std::getline
function rather than add a conditional check in the previous code example. getline
reads characters from the given input stream and stores them into a string
object. The function also takes an optional third argument to specify the delimiter by which to split input string. We also store the extracted strings in the std::vector
container on each iteration for later usage.
#include <algorithm>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "1,2,3,4,5,6,7,8,9,10";
char delimiter = ',';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, delimiter)) {
words.push_back(word);
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
Output:
1
2
3
4
5
6
7
8
9
10
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++