在 C++ 中解析逗号分隔的字符串序列
Jinku Hu
2023年10月12日
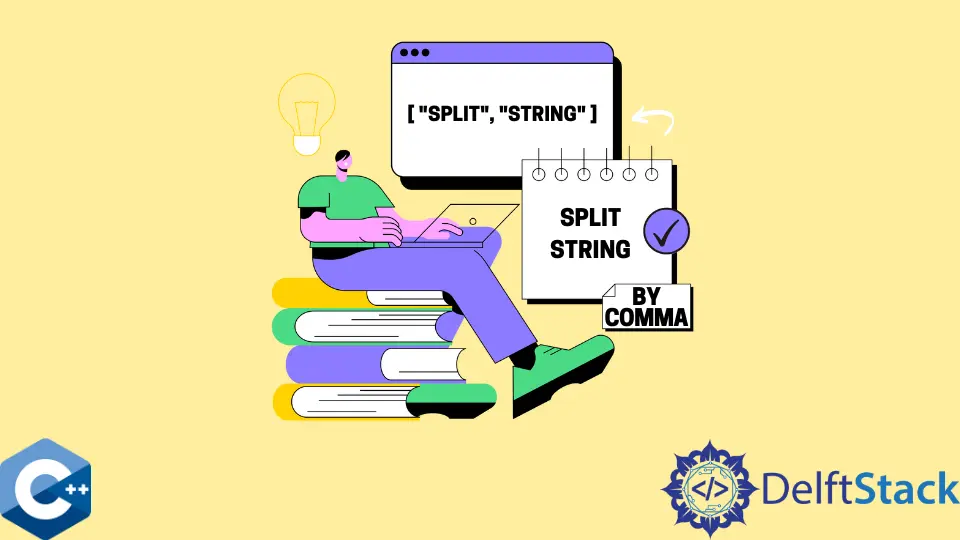
本文将演示有关如何在 C++ 中解析逗号分隔的字符串序列的多种方法。
使用 std::string::find
和 std::string::erase
函数来解析 C++ 中逗号分隔的字符串序列
std::find
方法是 std::string
类的内置函数,可用于查找给定子字符串的位置。在这种情况下,它采用一个参数来表示要找到的子字符串。任何多字符字符串变量都可以初始化为作为参数传递。尽管在下面的示例中,我们声明了一个包含字符串的逗号。由于 find
方法会返回子字符串和 string::npos
的位置(当未找到子字符串时),因此我们可以将比较表达式放入 while
循环中,该循环将执行直到表达式计算为真为止。
我们还将每次迭代后解析的字符串存储在 vector
容器中。然后,调用 erase
方法以删除在第一个逗号分隔符之前和包括的字符,以便在下一次迭代中继续处理相同的 string
对象。最后,我们将存储在向量中的元素输出到 cout
流。
#include <algorithm>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "1,2,3,4,5,6,7,8,9,10";
string delimiter = ",";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delimiter)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delimiter.length());
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
输出:
1
2
3
4
5
6
7
8
9
使用 std::getline
函数来解析逗号分隔的字符串序列
先前的解决方案未提取逗号分隔序列中的最后一个数字。因此,最好是通过 std::getline
函数使用以下方法,而不是在前面的代码示例中添加条件检查。getline
从给定的输入流中读取字符,并将其存储到 string
对象中。该函数还使用可选的第三个参数来指定分隔输入字符串的定界符。在每次迭代时,我们还将提取的字符串存储在 std::vector
容器中,以备后用。
#include <algorithm>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "1,2,3,4,5,6,7,8,9,10";
char delimiter = ',';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, delimiter)) {
words.push_back(word);
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
输出:
1
2
3
4
5
6
7
8
9
10
作者: Jinku Hu