在 C++ 中解析逗號分隔的字串序列
Jinku Hu
2023年10月12日
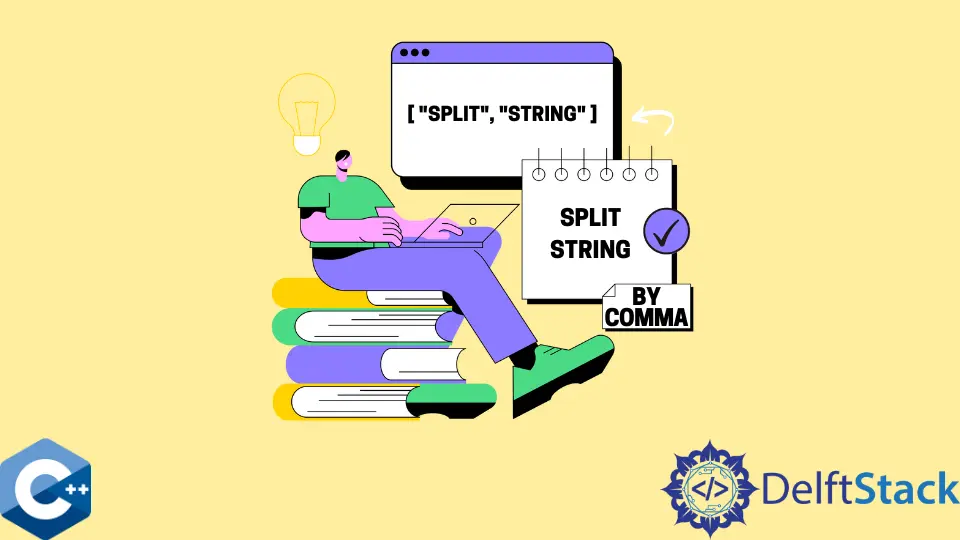
本文將演示有關如何在 C++ 中解析逗號分隔的字串序列的多種方法。
使用 std::string::find
和 std::string::erase
函式來解析 C++ 中逗號分隔的字串序列
std::find
方法是 std::string
類的內建函式,可用於查詢給定子字串的位置。在這種情況下,它採用一個引數來表示要找到的子字串。任何多字元字串變數都可以初始化為作為引數傳遞。儘管在下面的示例中,我們宣告瞭一個包含字串的逗號。由於 find
方法會返回子字串和 string::npos
的位置(當未找到子字串時),因此我們可以將比較表示式放入 while
迴圈中,該迴圈將執行直到表示式計算為真為止。
我們還將每次迭代後解析的字串儲存在 vector
容器中。然後,呼叫 erase
方法以刪除在第一個逗號分隔符之前和包括的字元,以便在下一次迭代中繼續處理相同的 string
物件。最後,我們將儲存在向量中的元素輸出到 cout
流。
#include <algorithm>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "1,2,3,4,5,6,7,8,9,10";
string delimiter = ",";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delimiter)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delimiter.length());
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
輸出:
1
2
3
4
5
6
7
8
9
使用 std::getline
函式來解析逗號分隔的字串序列
先前的解決方案未提取逗號分隔序列中的最後一個數字。因此,最好是通過 std::getline
函式使用以下方法,而不是在前面的程式碼示例中新增條件檢查。getline
從給定的輸入流中讀取字元,並將其儲存到 string
物件中。該函式還使用可選的第三個引數來指定分隔輸入字串的定界符。在每次迭代時,我們還將提取的字串儲存在 std::vector
容器中,以備後用。
#include <algorithm>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "1,2,3,4,5,6,7,8,9,10";
char delimiter = ',';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, delimiter)) {
words.push_back(word);
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
輸出:
1
2
3
4
5
6
7
8
9
10
作者: Jinku Hu