How to Remove Element From Array in C++
-
Use
std::to_array
andstd::remove
Functions to Remove Element From an Array in C++ -
Use
std::erase
andstd::remove
Functions to Remove Element From an Array in C++
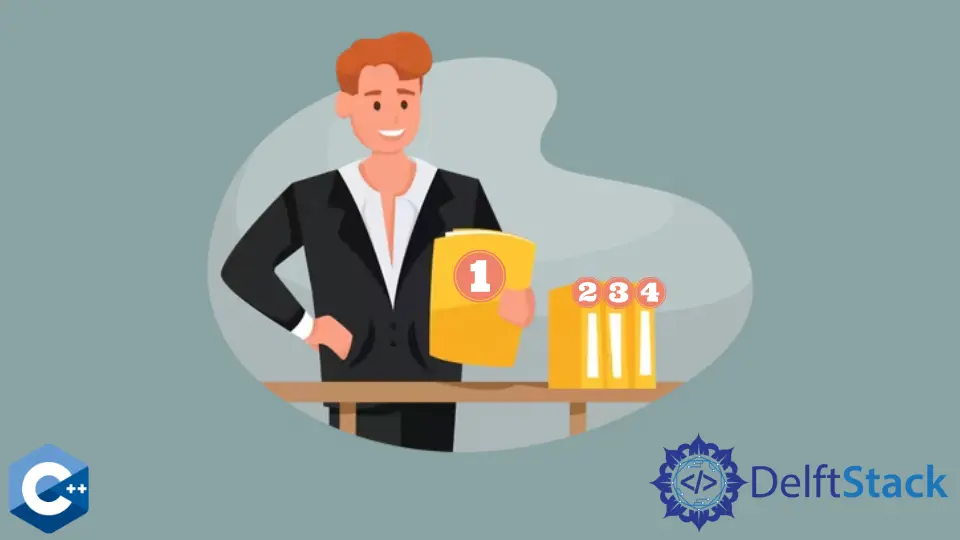
This article will explain several methods of how to remove an element from an array in C++.
Use std::to_array
and std::remove
Functions to Remove Element From an Array in C++
Arrays can be defined as fixed-length or dynamic in C++, and both of them need different methods for element removal. In this example, we consider the built-in C-style fixed arrays, since these are commonly manipulated by the numerical programs for efficiency.
We will declare an array of int
and remove the element value of 2
, which occurs twice in this array. std::remove
is part of the algorithms library and it removes all instances of the given element in the specified range.
Although, at first, we convert the arr
object to the std::array
container using std::to_array
function in order to safely use with std::remove
method. The latter algorithm returns the iterator for the new end of the range, which means that the resulting array
object still contains 10
elements, and we need to copy them to the new location. Since the original object was a C-style array, we allocate new dynamic memory for the eight-element int
array and use the std::memmove
function to copy the contents from the array
object. Mind though, that we calculated the 8
value using the std::distance
function.
#include <array>
#include <cstring>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::remove;
int main() {
int arr[10] = {1, 1, 1, 2, 2, 6, 7, 8, 9, 10};
int elem_to_remove = 2;
cout << "| ";
for (const auto &item : arr) {
cout << item << " | ";
}
cout << endl;
auto tmp = std::to_array(arr);
auto len =
std::distance(tmp.begin(), (tmp.begin(), tmp.end(), elem_to_remove));
auto new_arr = new int[len];
std::memmove(new_arr, tmp.data(), len * sizeof(int));
cout << "| ";
for (int i = 0; i < len; ++i) {
cout << new_arr[i] << " | ";
}
cout << endl;
delete[] new_arr;
return EXIT_SUCCESS;
}
Output:
| 1 | 1 | 1 | 2 | 2 | 6 | 7 | 8 | 9 | 10 |
| 1 | 1 | 1 | 6 | 7 | 8 | 9 | 10 |
Use std::erase
and std::remove
Functions to Remove Element From an Array in C++
Another scenario for this problem occurs when the given array is of type std::vector
. This time, we have the dynamic array features, and it’s more flexible to use a built-in function for element manipulations.
In the following example code, we will utilize the erase-remove idiom and remove all occurrences of the given element in the range. Note that std::erase
takes two iterators to denote the range for removal. Thus, it needs the return value from the std::remove
algorithm to specify the starting point. Mind that, if we call only std::remove
method the arr2
object will have elements as - {1, 1, 1, 6, 7, 8, 9, 10, 9, 10}
.
#include <iostream>
#include <iterator>
#include <vector>
using std::cout;
using std::endl;
using std::remove;
using std::vector;
int main() {
vector<int> arr2 = {1, 1, 1, 2, 2, 6, 7, 8, 9, 10};
int elem_to_remove = 2;
cout << "| ";
for (const auto &item : arr2) {
cout << item << " | ";
}
cout << endl;
arr2.erase(std::remove(arr2.begin(), arr2.end(), elem_to_remove), arr2.end());
cout << "| ";
for (const auto &item : arr2) {
cout << item << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
| 1 | 1 | 1 | 2 | 2 | 6 | 7 | 8 | 9 | 10 |
| 1 | 1 | 1 | 6 | 7 | 8 | 9 | 10 |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook