C++의 배열에서 요소 제거
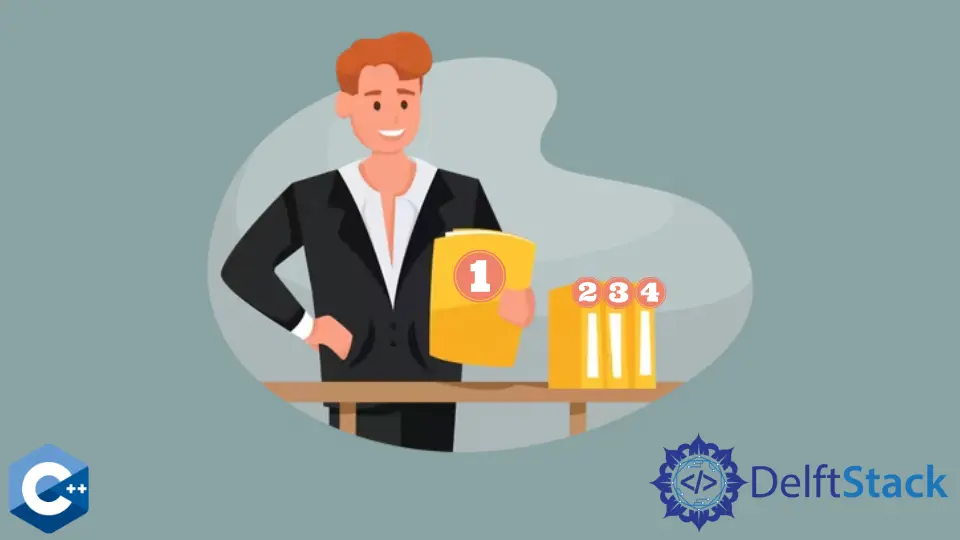
이 기사에서는 C++의 배열에서 요소를 제거하는 방법에 대한 몇 가지 방법을 설명합니다.
std::to_array
및std::remove
함수를 사용하여 C++의 배열에서 요소 제거
배열은 C++에서 고정 길이 또는 동적으로 정의 할 수 있으며 둘 다 요소 제거를 위해 다른 방법이 필요합니다. 이 예에서는 기본 제공 C 스타일 고정 배열을 고려합니다. 이러한 배열은 일반적으로 효율성을 위해 수치 프로그램에 의해 조작되기 때문입니다.
int
배열을 선언하고이 배열에서 두 번 발생하는2
요소 값을 제거합니다. std::remove
는 알고리즘 라이브러리의 일부이며 지정된 범위에서 지정된 요소의 모든 인스턴스를 제거합니다.
처음에는std::remove
메소드와 함께 안전하게 사용하기 위해std::to_array
함수를 사용하여arr
객체를std::array
컨테이너로 변환합니다. 후자의 알고리즘은 범위의 새로운 끝에 대한 반복자를 반환합니다. 즉, 결과array
객체에는 여전히10
요소가 포함되어 있으며 새 위치에 복사해야합니다. 원래 객체가 C 스타일 배열이기 때문에 8 개 요소int
배열에 새 동적 메모리를 할당하고std::memmove
함수를 사용하여array
객체에서 내용을 복사합니다. 하지만std::distance
함수를 사용하여8
값을 계산했습니다.
#include <array>
#include <cstring>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::remove;
int main() {
int arr[10] = {1, 1, 1, 2, 2, 6, 7, 8, 9, 10};
int elem_to_remove = 2;
cout << "| ";
for (const auto &item : arr) {
cout << item << " | ";
}
cout << endl;
auto tmp = std::to_array(arr);
auto len =
std::distance(tmp.begin(), (tmp.begin(), tmp.end(), elem_to_remove));
auto new_arr = new int[len];
std::memmove(new_arr, tmp.data(), len * sizeof(int));
cout << "| ";
for (int i = 0; i < len; ++i) {
cout << new_arr[i] << " | ";
}
cout << endl;
delete[] new_arr;
return EXIT_SUCCESS;
}
출력:
| 1 | 1 | 1 | 2 | 2 | 6 | 7 | 8 | 9 | 10 |
| 1 | 1 | 1 | 6 | 7 | 8 | 9 | 10 |
std::erase
및std::remove
함수를 사용하여 C++의 배열에서 요소 제거
이 문제에 대한 또 다른 시나리오는 주어진 배열이std::vector
유형일 때 발생합니다. 이번에는 동적 배열 기능이 있으며 요소 조작에 내장 함수를 사용하는 것이 더 유연합니다.
다음 예제 코드에서는 erase-remove 관용구를 활용하고 범위에서 주어진 요소의 모든 발생을 제거합니다. std::erase
는 제거 범위를 표시하기 위해 두 개의 반복자를 사용합니다. 따라서 시작점을 지정하려면std::remove
알고리즘의 반환 값이 필요합니다. std::remove
메소드 만 호출하면arr2
객체는-{1, 1, 1, 6, 7, 8, 9, 10, 9, 10}
과 같은 요소를 갖게됩니다.
#include <iostream>
#include <iterator>
#include <vector>
using std::cout;
using std::endl;
using std::remove;
using std::vector;
int main() {
vector<int> arr2 = {1, 1, 1, 2, 2, 6, 7, 8, 9, 10};
int elem_to_remove = 2;
cout << "| ";
for (const auto &item : arr2) {
cout << item << " | ";
}
cout << endl;
arr2.erase(std::remove(arr2.begin(), arr2.end(), elem_to_remove), arr2.end());
cout << "| ";
for (const auto &item : arr2) {
cout << item << " | ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
| 1 | 1 | 1 | 2 | 2 | 6 | 7 | 8 | 9 | 10 |
| 1 | 1 | 1 | 6 | 7 | 8 | 9 | 10 |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook