The new Keyword and No Match for Operator Error in C++
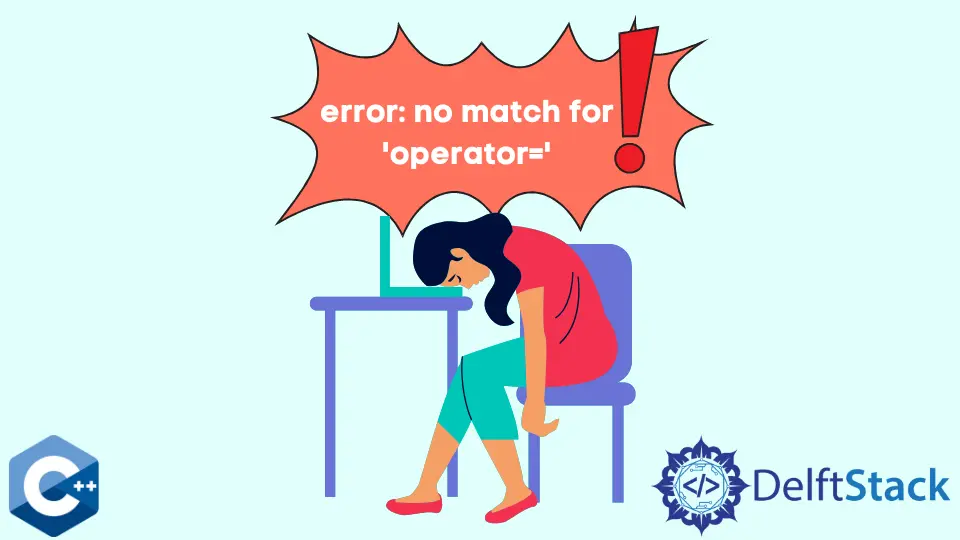
This article will discuss using the new
keyword in C++. Novice programmers usually make many mistakes while using the new
keyword.
We will also discuss some of the most common errors they make and their potential fixes.
the new
Keyword in C++
The new
keyword is used as an operator that places a request to the operating system to allocate some space on Heap memory. If that required amount of memory is available on the heap, it is allocated, and the address of that allocated, and initialized memory is returned in the pointer variable.
The syntax for using the new
keyword is:
datatype pointer_variable_name = new datatype;
This data type can also be some implicit data type or any class name. When we make a pointer variable of a class with the new
keyword, the class constructor is called, and memory is allocated on the heap.
If we do not use a new
keyword and the variable is initialized on the stack, that variable is automatically destroyed when it goes out of scope. But this is not the case with heap variables.
Heap variables are not destroyed automatically; rather, they need to be destroyed manually using the delete
operator; otherwise, they become dangling in the memory, which causes the issues of memory leakage.
Let’s look at the example below.
#include <iostream>
using namespace std;
int main() {
int *intPtr = new int;
*intPtr = 10;
cout << "Value of Integer pointer is: " << *intPtr << endl;
delete intPtr;
return 0;
}
Output:
Value of Integer pointer is: 10
Common Errors With the new
Keyword in C++
In certain situations, programmers do not initialize it with correct data and use the unallocated data, resulting in some unknown or unwanted data that can disturb the program execution. For example, there are 2 situations:
int *ptr = new int; // situation 1
int *ptr2 = new int[5]; // situation 2
In the first situation, memory is allocated to the single variable, and in the second situation, memory is allocated to 5
integer variables, i.e., an array of 5 indices is created on the heap.
Suppose we access ptr[3]
by declaring the pointer using the first situation. In that case, we are accessing an unallocated space which can result in some unknown error because we don’t know what was placed previously on that memory location.
Therefore we must be very careful while allocating and using the memory allocated on the heap.
Consider a situation where we have a pointer for a class, and we need to create an array of class objects.
class Students {
int roll_num;
string name;
};
int main() {
Students *student_list = new Students[10];
for (int k = 0; k < 10; k++) {
student_list[k] = new Students(); // line 10
}
}
In the above code snippet, at line 10, we are initializing the object at each index of the array. But this line will produce an error like this:
/tmp/qqnmmMnApj.cpp: In function 'int main()':
/tmp/qqnmmMnApj.cpp:14:40: error: no match for 'operator=' (operand types are 'Students' and 'Students*')
14 | student_list[k] = new Students();
| ^
This is because every index of the array student_list
is an object of the Students
class, not the pointer of the Students
class. Therefore, we do not need a new
keyword to initialize it.
It should be initialized like this:
student_list[k] = Students();
Another common error is accessing a memory location already deleted or freed up. There are certain situations where some function deallocates the allocated memory from the heap using the delete
operator, and at any other point in the program, you try to access that location.
This can cause an error and is very difficult to trace down, especially when working on larger projects. Consider the example below.
int main() {
int *p = new int;
*p = 5;
cout << "before deletion: " << *p << endl;
delete p;
cout << "After deletion: " << *p << endl;
}
Output:
before deletion: 5
After deletion: 0
We can see in the output that after deletion, the value is erased from the memory; therefore, it returns zero. In larger projects, this can cause unwanted errors at runtime because there can be a case where freed-up memory location is acquired by some other program and contains other data that can disturb your program logic.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn