How to Print an Array in C++
- Method 1: Using a Simple Loop
- Method 2: Using Range-Based For Loop
-
Method 3: Use the
copy
Function - Method 4: Using the Standard Template Library (STL)
-
Method 5: Use
for_each
Algorithm to Print Out an Array - Conclusion
- FAQ
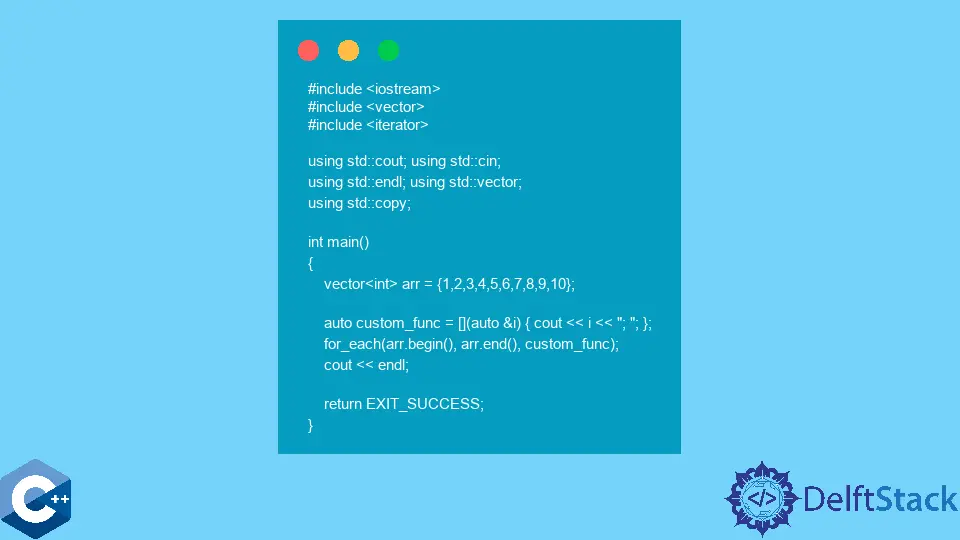
Printing an array in C++ might seem trivial, but it’s a fundamental skill that every programmer should master. Whether you’re debugging your code or just want to visualize the contents of an array, knowing how to effectively display array elements can be incredibly useful.
In this article, we will explore various methods to print an array in C++. We’ll cover the traditional way using loops, as well as more modern approaches such as using the Standard Template Library (STL). By the end of this guide, you’ll have a solid understanding of how to print arrays in C++ with clarity and efficiency.
Method 1: Using a Simple Loop
One of the most straightforward ways to print an array in C++ is by using a simple for loop. This method allows you to iterate through each element of the array and print it individually. Here’s how you can do it:
#include <iostream>
using namespace std;
int main() {
int arr[] = {10, 20, 30, 40, 50};
int length = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < length; i++) {
cout << arr[i] << " ";
}
return 0;
}
Output:
10 20 30 40 50
In this code, we first define an array arr
containing five integers. The length of the array is calculated using sizeof(arr) / sizeof(arr[0])
, which gives us the number of elements in the array. The for loop then iterates from 0 to the length of the array, printing each element followed by a space. This method is efficient and easy to understand, making it a great choice for beginners.
Method 2: Using Range-Based For Loop
C++11 introduced a more elegant way to iterate through arrays: the range-based for loop. This method simplifies the syntax and improves code readability. Here’s how you can use it to print an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {10, 20, 30, 40, 50};
for (int element : arr) {
cout << element << " ";
}
return 0;
}
Output:
10 20 30 40 50
In this example, the range-based for loop iterates directly over the elements of the array arr
. The variable element
takes the value of each item in the array during each iteration. This method reduces the risk of errors associated with indexing and makes the code cleaner and easier to read. It’s a preferred method for many developers when dealing with arrays.
Method 3: Use the copy
Function
copy()
function is implemented in the STL <algorithm>
library and offers a powerful tool for range-based operations. copy
takes start and endpoint iterators of the range as the first two parameters. In this case, we pass an output stream iterator as the third argument to output array elements to the console.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
Output:
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
As an alternative, we can easily reimplement the above example to output the array elements in reverse order. We modify the first two arguments of the copy()
function and substitute them with rbegin
/rend
function calls to achieve this.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
copy(arr.rbegin(), arr.rend(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
Output:
10; 9; 8; 7; 6; 5; 4; 3; 2; 1;
Method 4: Using the Standard Template Library (STL)
If you want to take advantage of C++’s Standard Template Library, you can use std::vector
instead of a traditional array. This approach not only allows dynamic sizing but also provides built-in methods for printing. Here’s how to print a vector:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> vec = {10, 20, 30, 40, 50};
for (const auto& element : vec) {
cout << element << " ";
}
return 0;
}
Output:
10 20 30 40 50
In this snippet, we use a std::vector
to hold our integers. The range-based for loop works similarly to the previous example, iterating through each element in the vector. The use of const auto&
ensures that we are not making unnecessary copies of the elements, which can be crucial for performance in larger datasets. This method is highly recommended for modern C++ programming, especially when dealing with collections of data.
Method 5: Use for_each
Algorithm to Print Out an Array
for_each
is another powerful algorithm from the STL library. It can apply the given function object to every element of the range. In this case, we define a lambda
expression as a custom_func
variable and pass it to the for_each
method to operate on the given array elements.
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto custom_func = [](auto &i) { cout << i << "; "; };
for_each(arr.begin(), arr.end(), custom_func);
cout << endl;
return EXIT_SUCCESS;
}
Output:
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
This code demonstrates the use of std::for_each
, an STL algorithm, to iterate over and process elements in a vector. It creates a vector of integers and defines a lambda function custom_func
that prints each element followed by a semicolon. The for_each
function applies this custom function to every element in the vector, resulting in all elements being printed. This approach showcases a concise way to perform operations on container elements without explicit loops, leveraging modern C++ features for cleaner and potentially more efficient code.
Conclusion
Printing an array in C++ can be accomplished through various methods, each with its own advantages. Whether you opt for a traditional loop, a range-based for loop, or utilize the STL with vectors, understanding these techniques will enhance your programming skills. As you continue your journey in C++, remember that mastering these basic operations lays the groundwork for more complex data manipulations.
FAQ
-
What is the difference between an array and a vector in C++?
An array has a fixed size and cannot be resized after its declaration, while a vector is dynamic and can resize itself automatically. -
Can I print multi-dimensional arrays in C++?
Yes, you can print multi-dimensional arrays using nested loops to access each element. -
Is using std::vector better than using arrays?
In many cases, yes. Vectors provide more flexibility and safety features compared to traditional arrays. -
How do I print an array of strings in C++?
You can use a loop similar to the integer examples, but ensure the array is of typestring
instead ofint
. -
What is the best practice for printing large arrays?
For large arrays, consider printing only a subset of elements or using formatting options to enhance readability.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook