C++에서 배열을 인쇄하는 방법
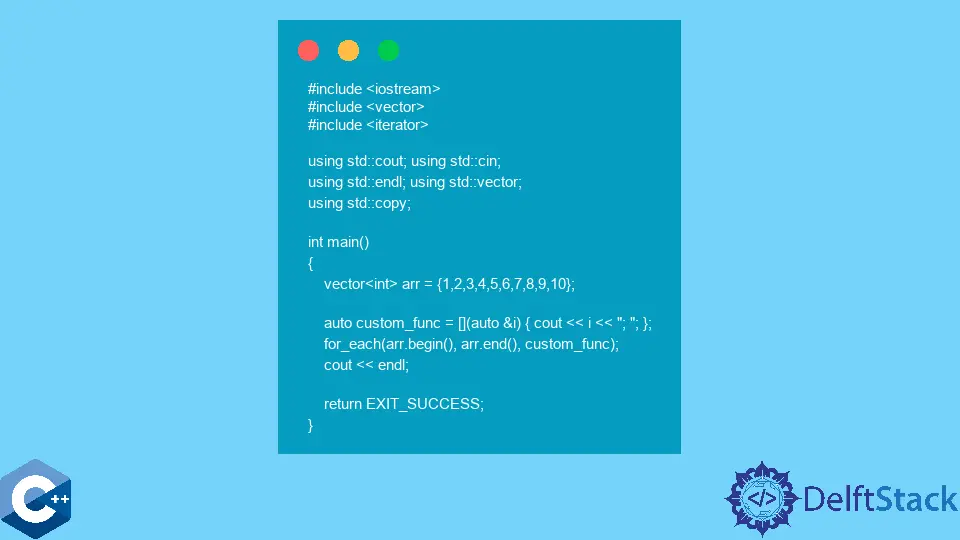
이 기사에서는 배열의 요소를 콘솔에 출력하는 C++ 메서드를 소개합니다.
범위 기반 루프를 사용하여 배열 인쇄
이 메서드는 최신 C++ 11 범위 기반 스타일에서만 사용되는 일반적인 for
루프입니다. 범위 기반 반복은 const 참조 (auto const& i
), 값 (auto i
) 또는 전달 참조 (auto i
)와 같은 사용자 지정 지정자를 사용하여 요소에 액세스하는 옵션을 제공합니다. 기존의 for
루프에 비해이 방법의 이점은 사용 용이성과 가독성입니다.
#include <iostream>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (auto const& value : arr) cout << value << "; ";
cout << endl;
return EXIT_SUCCESS;
}
출력:
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
copy
기능을 사용하여 배열 인쇄
copy()
함수는 STL <algorithm>
라이브러리에서 구현되며 범위 기반 작업을위한 강력한 도구를 제공합니다. copy
는 범위의 시작 및 끝점 반복자를 처음 두 매개 변수로 사용합니다. 이 경우 출력 스트림 반복기를 세 번째 인수로 전달하여 array
요소를 콘솔에 출력합니다.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
출력:
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
대안으로 위의 예를 쉽게 다시 구현하여 배열 요소를 역순으로 출력 할 수 있습니다. copy()
함수의 처음 두 인수를 수정하고이를rbegin
/rend
함수 호출로 대체하여이를 달성합니다.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
copy(arr.rbegin(), arr.rend(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
출력:
10; 9; 8; 7; 6; 5; 4; 3; 2; 1;
for_each
알고리즘을 사용하여 배열 인쇄
for_each
는 STL 라이브러리의 또 다른 강력한 알고리즘입니다. 주어진 함수 객체를 범위의 모든 요소에 적용 할 수 있습니다. 이 경우lambda
표현식을custom_func
변수로 정의하고 주어진 배열 요소에서 작동하도록for_each
메서드에 전달합니다.
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto custom_func = [](auto &i) { cout << i << "; "; };
for_each(arr.begin(), arr.end(), custom_func);
cout << endl;
return EXIT_SUCCESS;
}
출력:
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook