How to Convert String to Uppercase in C++
-
Use
std::transform()
andstd::toupper()
to Convert String to Uppercase -
Use
icu::UnicodeString
andtoUpper()
to Convert String to Uppercase -
Use
icu::UnicodeString
andtoUpper()
With Specific Locale to Convert String to Uppercase
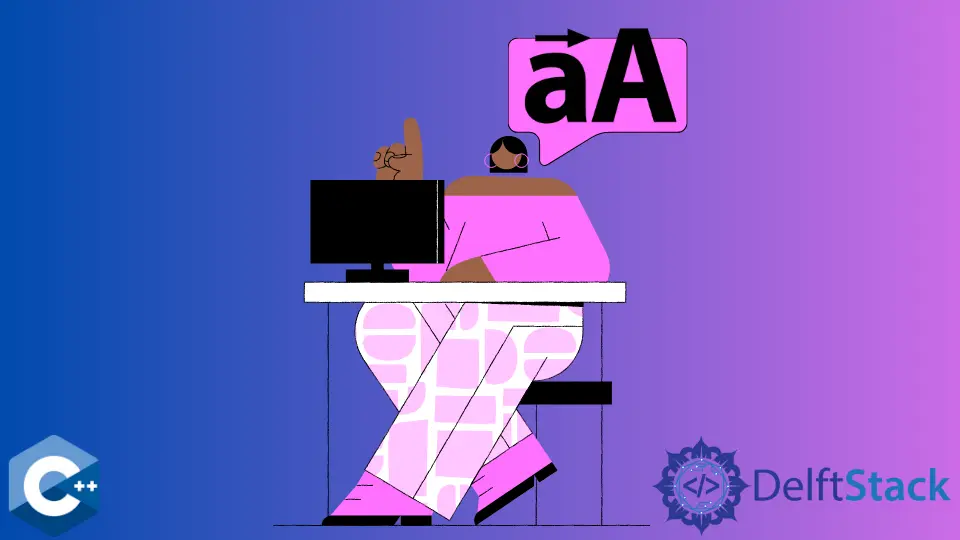
This article will explain several C++ methods of how to convert a string
to uppercase.
Use std::transform()
and std::toupper()
to Convert String to Uppercase
std::transform
method is from the STL <algorithm>
library, and it can apply the given function to a range. In this example, we utilize it to operate on std::string
characters range and convert each char
to uppercase letters using the toupper
function. Notice that, even though this method successfully transforms the single-byte characters from the given string, characters with multi-byte encoding don’t get capitalized, as seen in the program output.
#include <iostream>
#include <algorithm>
#include <string>
using std::cout; using std::string;
using std::endl; using std::cin;
using std::transform; using std::toupper;
string capitalizeString(string s)
{
transform(s.begin(), s.end(), s.begin(),
[](unsigned char c){ return toupper(c); });
return s;
}
int main() {
string string1("hello there είναι απλά ένα κείμενο χωρίς");
cout << "input string: " << string1 << endl
<< "output string: " << capitalizeString(string1) << endl << endl;
return 0;
}
Output:
input string: hello there είναι απλά ένα κείμενο χωρίς
output string: HELLO THERE είναι απλά ένα κείμενο χωρίς
Use icu::UnicodeString
and toUpper()
to Convert String to Uppercase
The above code works fine for the ASCII strings and some other characters, but if you pass e.g. certain Unicode string sequences, the toupper
function won’t capitalize them. So, the portable solution is to use routines from the ICU
(International Components for Unicode) library, which is mature enough to offer stability, is widely accessible, and will keep your code cross-platform.
To utilize the ICU
library you should include the following headers <unicode/unistr.h>
, <unicode/ustream.h>
and <unicode/locid.h>
in your source file. There’s a good chance that these libraries are already installed and available on your operating system, and code examples should work just fine. But if you get compile-time errors, refer to the instructions on the ICU website about how to download libraries for specific platforms.
Note that you should provide the following compiler flags to successfully link with the ICU library dependencies:
g++ sample_code.cpp -licuio -licuuc -o sample_code
#include <iostream>
#include <string>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <unicode/locid.h>
using std::cout; using std::string;
using std::endl; using std::cin;
using std::transform; using std::toupper;
int main() {
string string1("hello there είναι απλά ένα κείμενο χωρίς");
icu::UnicodeString unicodeString(string1.c_str());
cout << "input string: " << string1 << endl
<< "output string: " << unicodeString.toUpper() << endl;
return 0;
}
Output:
input string: hello there είναι απλά ένα κείμενο χωρίς
output string: HELLO THERE ΕΊΝΑΙ ΑΠΛΆ ΈΝΑ ΚΕΊΜΕΝΟ ΧΩΡΊΣ
Use icu::UnicodeString
and toUpper()
With Specific Locale to Convert String to Uppercase
toUpper
function can also accept locale
parameter to operate on the string with the conventions of a specific locale
. The argument to be passed can be constructed separately as icu::Locale
object or you can just specify locale in string literal to toUpper
function, as demonstrated in the next code sample:
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <iostream>
int main() {
string string2 = "Contrairement à une opinion répandue";
icu::UnicodeString unicodeString2(string2.c_str());
cout << unicodeString2.toUpper("fr-FR") << endl;
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++