如何在 C++ 中將字串轉換為大寫
Jinku Hu
2023年12月11日
C++
C++ String
-
使用
std::transform()
和std::toupper()
將字串轉換為大寫字母 -
使用
icu::UnicodeString
和toUpper()
將字串轉換為大寫 -
使用
icu::UnicodeString
和toUpper()
與特定的地域轉換字串為大寫字母
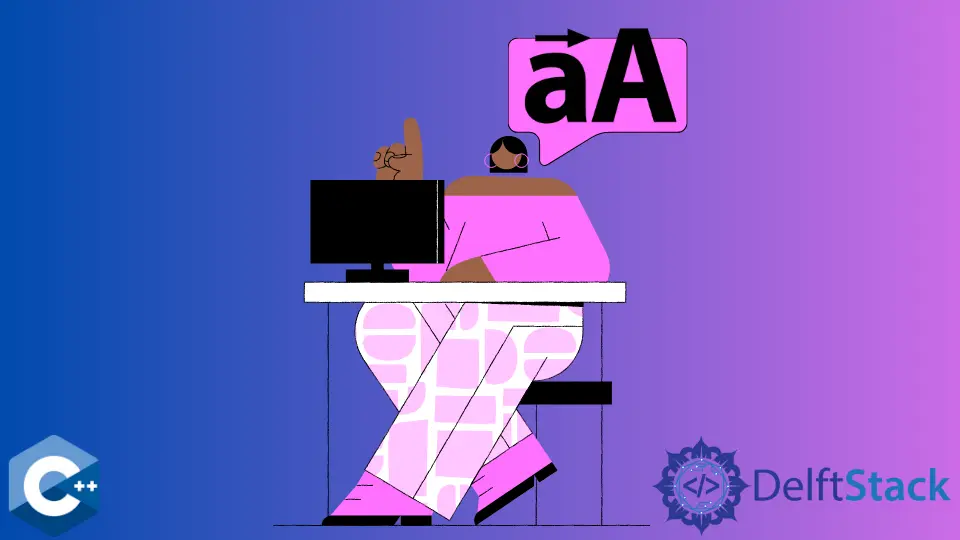
本文將介紹幾種將 string
轉換為大寫字母的 C++ 方法。
使用 std::transform()
和 std::toupper()
將字串轉換為大寫字母
std::transform
方法來自 STL <algorithm>
庫,它可以將給定的函式應用於一個範圍。在本例中,我們利用它對 std::string
字元範圍進行操作,並使用 toupper
函式將每個 char
轉換為大寫字母。請注意,儘管這個方法成功地轉換了給定字串中的單位元組字元,但如程式輸出所示,具有多位元組編碼的字元不會被轉換為大寫。
#include <iostream>
#include <algorithm>
#include <string>
using std::cout; using std::string;
using std::endl; using std::cin;
using std::transform; using std::toupper;
string capitalizeString(string s)
{
transform(s.begin(), s.end(), s.begin(),
[](unsigned char c){ return toupper(c); });
return s;
}
int main() {
string string1("hello there είναι απλά ένα κείμενο χωρίς");
cout << "input string: " << string1 << endl
<< "output string: " << capitalizeString(string1) << endl << endl;
return 0;
}
輸出:
input string: hello there είναι απλά ένα κείμενο χωρίς
output string: HELLO THERE είναι απλά ένα κείμενο χωρίς
使用 icu::UnicodeString
和 toUpper()
將字串轉換為大寫
上面的程式碼對於 ASCII 字串和一些其他字元都能正常工作,但是如果你傳遞某些 Unicode 字串序列,toupper
函式就不能將它們大寫。所以,可移植的解決方案是使用 ICU
(International Components for Unicode)庫中的例程,它足夠成熟,提供了穩定性,被廣泛使用,並能保證你的程式碼跨平臺。
要使用 ICU
庫,你應該在你的原始檔中包含以下標頭檔案 <unicode/unistr.h>
,<unicode/ustream.h>
和 <unicode/locid.h>
。這些庫很有可能已經安裝在你的作業系統上,並且可以使用,程式碼示例應該可以正常工作。但是如果你得到編譯時的錯誤,請參考 ICU 網站上關於如何下載特定平臺的庫的說明。
需要注意的是,你應該提供以下編譯器標誌才能成功地與 ICU 庫依賴連結。
g++ sample_code.cpp -licuio -licuuc -o sample_code
#include <iostream>
#include <string>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <unicode/locid.h>
using std::cout; using std::string;
using std::endl; using std::cin;
using std::transform; using std::toupper;
int main() {
string string1("hello there είναι απλά ένα κείμενο χωρίς");
icu::UnicodeString unicodeString(string1.c_str());
cout << "input string: " << string1 << endl
<< "output string: " << unicodeString.toUpper() << endl;
return 0;
}
輸出:
input string: hello there είναι απλά ένα κείμενο χωρίς
output string: HELLO THERE ΕΊΝΑΙ ΑΠΛΆ ΈΝΑ ΚΕΊΜΕΝΟ ΧΩΡΊΣ
使用 icu::UnicodeString
和 toUpper()
與特定的地域轉換字串為大寫字母
toUpper
函式也可以接受 locale
引數,以特定的 locale
慣例對字串進行操作。要傳遞的引數可以單獨構造為 icu::Locale
物件,或者你可以直接在字串文字中指定 locale 給 toUpper
函式,如下面的程式碼示例所示。
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <iostream>
int main() {
string string2 = "Contrairement à une opinion répandue";
icu::UnicodeString unicodeString2(string2.c_str());
cout << unicodeString2.toUpper("fr-FR") << endl;
return 0;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu