如何在 C++ 中将字符串转换为大写
Jinku Hu
2023年12月11日
C++
C++ String
-
使用
std::transform()
和std::toupper()
将字符串转换为大写字母 -
使用
icu::UnicodeString
和toUpper()
将字符串转换为大写 -
使用
icu::UnicodeString
和toUpper()
与特定的地域转换字符串为大写字母
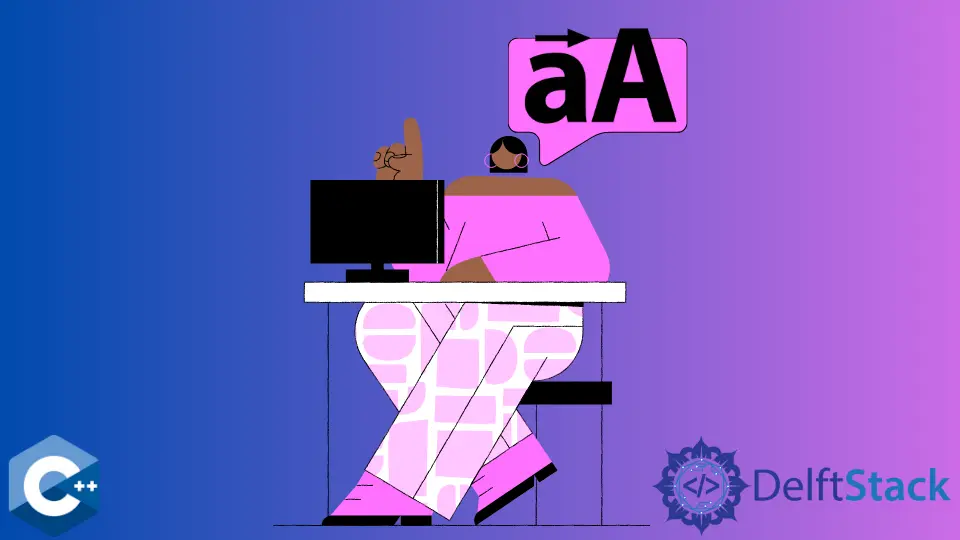
本文将介绍几种将 string
转换为大写字母的 C++ 方法。
使用 std::transform()
和 std::toupper()
将字符串转换为大写字母
std::transform
方法来自 STL <algorithm>
库,它可以将给定的函数应用于一个范围。在本例中,我们利用它对 std::string
字符范围进行操作,并使用 toupper
函数将每个 char
转换为大写字母。请注意,尽管这个方法成功地转换了给定字符串中的单字节字符,但如程序输出所示,具有多字节编码的字符不会被转换为大写。
#include <iostream>
#include <algorithm>
#include <string>
using std::cout; using std::string;
using std::endl; using std::cin;
using std::transform; using std::toupper;
string capitalizeString(string s)
{
transform(s.begin(), s.end(), s.begin(),
[](unsigned char c){ return toupper(c); });
return s;
}
int main() {
string string1("hello there είναι απλά ένα κείμενο χωρίς");
cout << "input string: " << string1 << endl
<< "output string: " << capitalizeString(string1) << endl << endl;
return 0;
}
输出:
input string: hello there είναι απλά ένα κείμενο χωρίς
output string: HELLO THERE είναι απλά ένα κείμενο χωρίς
使用 icu::UnicodeString
和 toUpper()
将字符串转换为大写
上面的代码对于 ASCII 字符串和一些其他字符都能正常工作,但是如果你传递某些 Unicode 字符串序列,toupper
函数就不能将它们大写。所以,可移植的解决方案是使用 ICU
(International Components for Unicode)库中的例程,它足够成熟,提供了稳定性,被广泛使用,并能保证你的代码跨平台。
要使用 ICU
库,你应该在你的源文件中包含以下头文件 <unicode/unistr.h>
,<unicode/ustream.h>
和 <unicode/locid.h>
。这些库很有可能已经安装在你的操作系统上,并且可以使用,代码示例应该可以正常工作。但是如果你得到编译时的错误,请参考 ICU 网站上关于如何下载特定平台的库的说明。
需要注意的是,你应该提供以下编译器标志才能成功地与 ICU 库依赖链接。
g++ sample_code.cpp -licuio -licuuc -o sample_code
#include <iostream>
#include <string>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <unicode/locid.h>
using std::cout; using std::string;
using std::endl; using std::cin;
using std::transform; using std::toupper;
int main() {
string string1("hello there είναι απλά ένα κείμενο χωρίς");
icu::UnicodeString unicodeString(string1.c_str());
cout << "input string: " << string1 << endl
<< "output string: " << unicodeString.toUpper() << endl;
return 0;
}
输出:
input string: hello there είναι απλά ένα κείμενο χωρίς
output string: HELLO THERE ΕΊΝΑΙ ΑΠΛΆ ΈΝΑ ΚΕΊΜΕΝΟ ΧΩΡΊΣ
使用 icu::UnicodeString
和 toUpper()
与特定的地域转换字符串为大写字母
toUpper
函数也可以接受 locale
参数,以特定的 locale
惯例对字符串进行操作。要传递的参数可以单独构造为 icu::Locale
对象,或者你可以直接在字符串文字中指定 locale 给 toUpper
函数,如下面的代码示例所示。
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <iostream>
int main() {
string string2 = "Contrairement à une opinion répandue";
icu::UnicodeString unicodeString2(string2.c_str());
cout << unicodeString2.toUpper("fr-FR") << endl;
return 0;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu