How to Clear Array Element Values in C++
-
Use the Built-In
fill()
Method to Clear Array Elements in C++ -
Use the
std::fill()
Algorithm to Clear Array Elements in C++ -
Use the
fill_n()
Algorithm to Clear Array Elements - Use a Loop Only to Clear Array Elements
- Conclusion
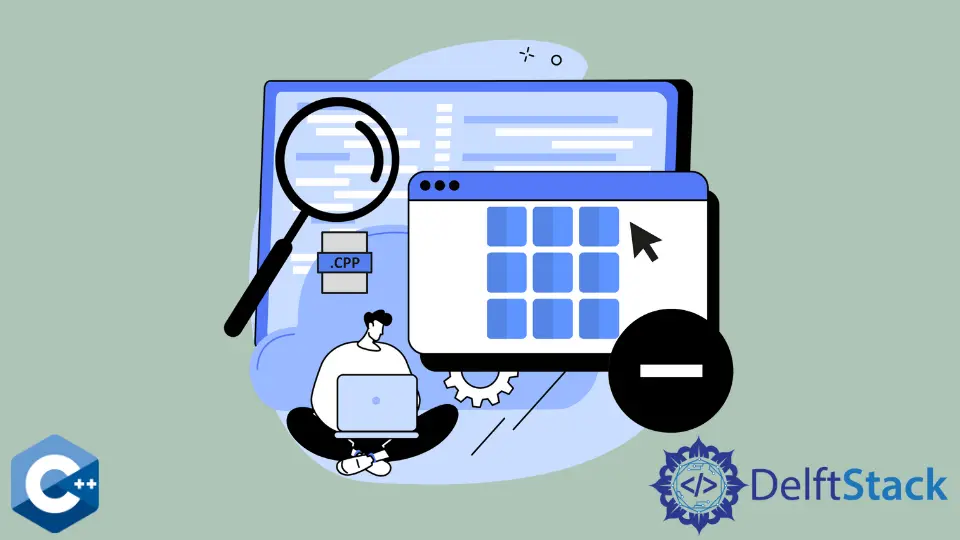
This article presents various methods to clear array element values in C++. Whether you are working with the standard std::array
, utilizing algorithms from the Standard Template Library (STL), or simply using loops, you’ll find different techniques to achieve this task efficiently.
Clearing array elements is a common operation in C++ when you want to reset or initialize the elements to a specific value. In this article, we will explore multiple approaches to accomplish this, allowing you to choose the one that best suits your needs.
Use the Built-In fill()
Method to Clear Array Elements in C++
The std::array
container provides multiple built-in methods that operate on its elements, one of which is the fill()
method. It assigns the given value to each element of the array object.
Note that you can construct an array with any element type and still use the fill()
function to pass the value to assign every member of the container.
Basic Syntax:
nums.fill(value);
Parameters:
nums
: This is the name of thestd::array
you want to modify.value
: This is the value that will replace all the elements in the array.
Example Code:
#include <algorithm>
#include <array>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::fill;
using std::fill_n;
int main() {
array<int, 10> nums{1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
cout << endl;
nums.fill(0);
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
return EXIT_SUCCESS;
}
Output:
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
In the given code, we start by including the necessary libraries and defining the standard aliases to make our code concise. We have an array of integers named nums
, initialized with values from 1
to 10
.
First, we print the original contents of the nums
array in a formatted way, surrounded by vertical bars. The for
loop iterates through each element of the array, and we use cout
to display each element’s value, creating a visual representation of the array.
After printing the original contents, we use the nums.fill(0)
statement. This built-in fill()
method is specific to the std::array
container and assigns the value 0
to every element in the array.
Following this, we print the modified contents of the nums
array in the same visually formatted way. All elements of the array have been set to 0
, which is evident in the output.
Use the std::fill()
Algorithm to Clear Array Elements in C++
Alternatively, we can utilize the std::fill
algorithm defined in the STL <algorithm>
library. This method is called on range range-based object and assigns a given value to its elements.
The range of elements is passed as the first two arguments, and the third one specifies a value to assign. Starting from the C++17 version, the user can also specify the execution policy as an optional argument (see the full details here).
Basic Syntax:
std::fill(nums.begin(), nums.end(), value);
Parameters:
nums.begin()
: This represents the iterator pointing to the first element in the range you want to modify.nums.end()
: This represents the iterator pointing one past the last element in the range you want to modify.value
: This is the value that will replace the elements within the specified range.
Example Code:
#include <algorithm>
#include <array>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::fill;
using std::fill_n;
int main() {
array<int, 10> nums{1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
cout << endl;
std::fill(nums.begin(), nums.end(), 0);
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
return EXIT_SUCCESS;
}
Output:
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
In this C++ code, we begin by including the necessary libraries and creating aliases for standard elements to simplify our code. We declare an array named nums
of size 10
and initialize it with integers from 1
to 10
.
We then proceed to print the original contents of the nums
array. Using a for
loop, we iterate through each element of the array, displaying each value surrounded by vertical bars for a visual representation of the array.
Following this, we employ the std::fill()
algorithm from the <algorithm>
library. This method is applied to the nums
array using the nums.begin()
and nums.end()
iterators and sets every element in the array to the value 0
.
Subsequently, we print the modified contents of the nums
array in the same visually formatted way. All elements in the array have been successfully set to 0
, as clearly seen in the output.
Use the fill_n()
Algorithm to Clear Array Elements
Another useful method from the <algorithm>
header is the fill_n
algorithm, which is similar to the above method except that it takes several elements that need to be modified with the given value. In this case, we specified the return value of the size()
method to emulate the identical behavior as in the above code example.
Note that the execution policy tag applies in this method as well.
Basic Syntax:
std::fill_n(nums.begin(), count, value);
Parameters:
nums.begin()
: This represents the iterator pointing to the first element in the range where you want to start filling.count
: This specifies the number of elements to be filled with the specified value.value
: This is the value that will replace the elements within the specified range.
Example Code:
#include <algorithm>
#include <array>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::fill;
using std::fill_n;
int main() {
array<int, 10> nums{1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
cout << endl;
std::fill_n(nums.begin(), nums.size(), 0);
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
return EXIT_SUCCESS;
}
Output:
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
In this code, we begin by including the necessary libraries and creating aliases for standard elements to simplify our code. We declare an array named nums
with a size of 10
and initialize it with integers ranging from 1
to 10
.
Next, we print the original contents of the nums
array. Using a for
loop, we iterate through each element of the array and display each value, enclosing it with vertical bars for a clear visual representation of the array.
Following this, we use the std::fill_n()
algorithm from the <algorithm>
library. This method is applied to the nums
array, starting from nums.begin()
and specifying the number of elements to modify, which is the size of the array, and it sets each element to the value 0
.
Afterward, we print the modified contents of the nums
array in the same visually formatted way. All elements in the array have been successfully set to 0
, as clearly seen in the output.
Use a Loop Only to Clear Array Elements
We can use a loop to clear the elements of the std::array
nums
by iterating through it and setting each element to a specific value (in this case, 0
). We can use loops like for
or while
to iterate through the array and set each element to a specific value.
In this example, we will use the for
loop.
#include <array>
#include <iostream>
using std::array;
using std::cout;
using std::endl;
int main() {
array<int, 10> nums{1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
cout << endl;
for (int &item : nums) {
item = 0;
}
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
return EXIT_SUCCESS;
}
Output:
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
In this code, we declare an array called nums
with a size of 10
and initialize it with integers from 1
to 10
.
Next, we print the original contents of the nums
array. We used a for
loop to iterate through each element in the array and display each value within vertical bars, providing a visual representation of the array.
Afterward, we employ a simple for
loop to clear the elements in the nums
array. We iterate through the array, setting each element to the value 0
.
Finally, we print the modified contents of the nums
array in the same visually formatted manner. All elements in the array have been successfully changed to 0
, as evident in the output.
Conclusion
Clearing array elements is a fundamental operation in C++ programming. In this article, we explored multiple methods to achieve this task, including the use of the built-in fill()
method provided by std::array
, the std::fill()
algorithm from the STL, and the fill_n()
algorithm and we also demonstrated how to clear array elements using a loop.
Each method has its advantages and use cases, so you can choose the one that best fits your specific requirements. By understanding these techniques, you can efficiently manage and manipulate array data in your C++ programs, making your code more robust and maintainable.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook