如何在 C++ 中清除数组元素值
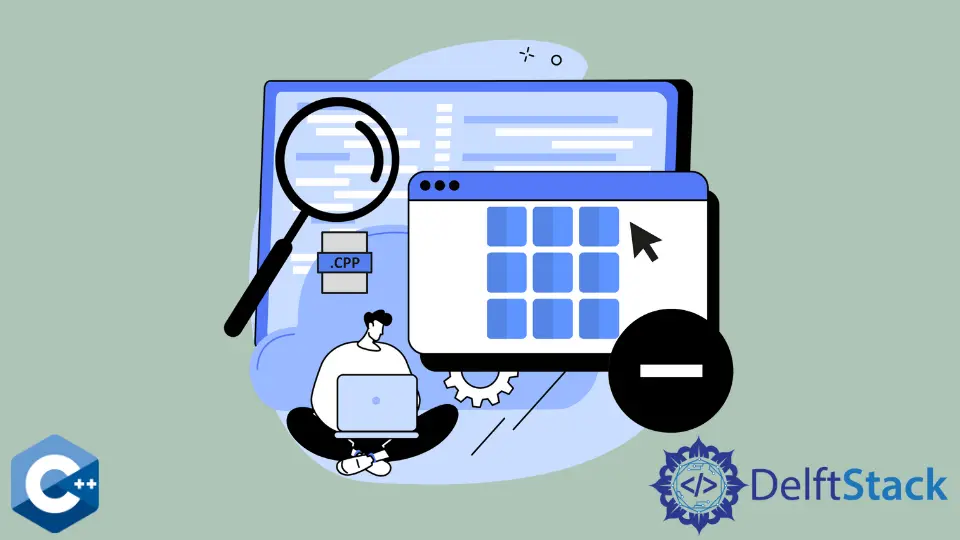
本文将介绍多种关于在 C++ 中如何清除数组元素值的方法。
使用 C++ 中内置的 fill()
方法清除数组元素
std::array
容器提供了多种对其元素进行操作的内置方法,其中之一是 fill()
方法。它将给定的值分配给数组对象的每个元素。请注意,你可以用任何元素类型构造一个数组,并且仍然可以使用 fill()
函数传递值来分配容器的每个成员。
#include <algorithm>
#include <array>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::fill;
using std::fill_n;
int main() {
array<int, 10> nums{1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
cout << endl;
nums.fill(0);
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
return EXIT_SUCCESS;
}
输出:
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
使用 std::fill()
算法在 C++ 中清除数组元素
或者,我们可以利用 STL <algorithm>
库中定义的 std::fill
算法。这个方法在基于范围的对象上被调用,并给它的元素分配一个给定的值。元素的范围作为前两个参数传递的,第三个参数指定一个要分配的值。从 C++ 17 版本开始,用户还可以将执行策略作为一个可选的参数来指定(详见全文这里)。
#include <algorithm>
#include <array>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::fill;
using std::fill_n;
int main() {
array<int, 10> nums{1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
cout << endl;
std::fill(nums.begin(), nums.end(), 0);
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
return EXIT_SUCCESS;
}
输出:
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
使用 fill_n()
算法清除数组元素
<algorithm>
头文件的另一个有用的方法是 fill_n
算法,它与上面的方法类似,只是它需要用给定的值修改几个元素。在这种情况下,我们指定了 size()
方法的返回值,以模拟与上面代码例子中相同的行为。注意,执行策略标签也适用于这个方法。
#include <algorithm>
#include <array>
#include <iostream>
#include <iterator>
using std::array;
using std::cout;
using std::endl;
using std::fill;
using std::fill_n;
int main() {
array<int, 10> nums{1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
cout << endl;
std::fill_n(nums.begin(), nums.size(), 0);
cout << "| ";
for (const auto &item : nums) {
cout << item << " | ";
}
return EXIT_SUCCESS;
}
输出:
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu