How to Get File Size in C++
-
How to Get File Size in C++ Using the
std::filesystem::file_size
Function -
How to Get File Size in C++ Using the
stat
Function -
How to Get File Size in C++ Using
Boost.Filesystem
- How to Get File Size in C++ Using C Standard Library Functions
- Conclusion
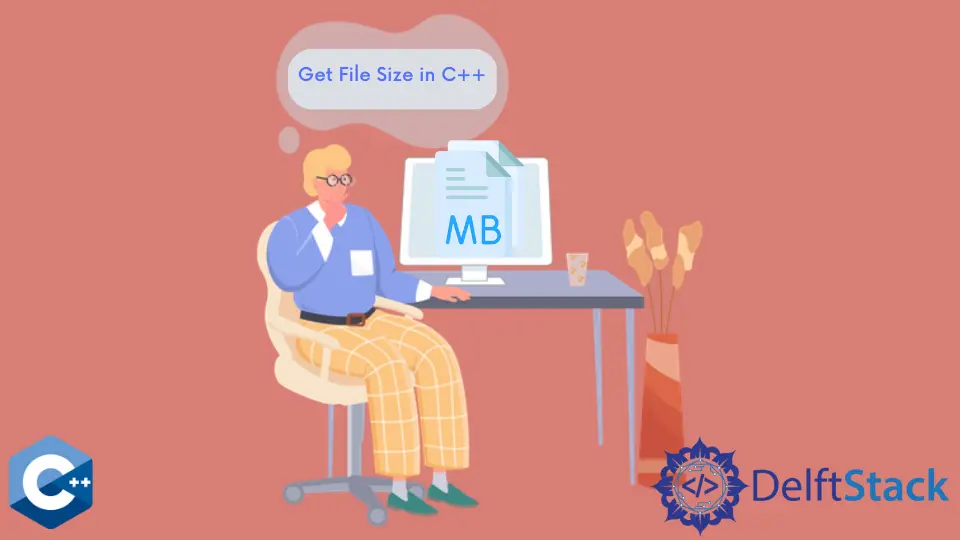
In C++ programming, efficiently retrieving the size of a file is a commonly encountered task. As the language evolves, various methods and libraries have emerged to streamline this process.
In this article, we will explore different approaches to getting file size in C++. We’ll cover modern techniques using the std::filesystem::file_size
function, delve into traditional methods using C standard library functions, explore POSIX-compliant stat
functions, and address scenarios where pre-C++17 codebases can leverage Boost.Filesystem
.
Each method has its own merits and use cases, providing us with a toolkit to choose the most suitable approach based on our project requirements and the C++ version in use.
How to Get File Size in C++ Using the std::filesystem::file_size
Function
The std::filesystem::file_size
function in C++ provides a convenient way to retrieve the size of a file in bytes. This function is part of the C++17 standard and later versions, and it is located in the std::filesystem
library.
By using this function, you can avoid platform-specific code and achieve a cleaner and more portable solution for obtaining file sizes.
The syntax of the std::filesystem::file_size
function is straightforward. It takes a single argument - the file path - and returns the size of the file in bytes as a std::uintmax_t
value.
Here’s the basic syntax:
#include <filesystem>
std::uintmax_t std::filesystem::file_size(const std::filesystem::path& p);
Here, p
represents the path to the file for which you want to determine the size. The function returns the size of the file in bytes as a std::uintmax_t
type.
Code Example:
#include <filesystem>
#include <iostream>
int main() {
// Specify the path to the file
std::filesystem::path filePath = "example.txt";
try {
// Use std::filesystem::file_size to get the file size
std::uintmax_t fileSize = std::filesystem::file_size(filePath);
// Output the result
std::cout << "File size: " << fileSize << " bytes" << std::endl;
} catch (const std::filesystem::filesystem_error& e) {
// Handle exceptions, if any
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
In this example, we begin by including the necessary headers for file system operations and standard input/output. We then declare a std::filesystem::path
object named filePath
and initialize it with the path to the target file example.txt
in this case).
Inside the try
block, we call std::filesystem::file_size(filePath)
to retrieve the size of the file specified by filePath
.
The result is stored in the variable fileSize
, which is of type std::uintmax_t
. We use std::cout
to display the file size in bytes.
To handle potential exceptions, we use a try...catch
block. If an exception occurs (for instance, if the file does not exist), the catch
block captures the exception and prints an error message using std::cerr
.
Code Output:
Assuming the file example.txt
exists, the output of the program would be:
If an error occurs, the program will output an error message detailing the nature of the issue.
How to Get File Size in C++ Using the stat
Function
The stat
function is another convenient and platform-independent way to determine the size of a file in C++. It is a part of the POSIX standard and is widely available across different operating systems.
The stat
function is part of the <sys/stat.h>
header and provides information about a file, including its size.
The syntax of the stat
function is as follows:
int stat(const char *path, struct stat *buf);
Parameters:
path
: The path to the file whose information is to be retrieved.buf
: A pointer to a structure of typestruct stat
that will store the file information.
As we can see, the stat
function takes the path to the file and a pointer to a struct stat
as a parameter. After the function is called, the struct stat
will be populated with various details about the file, such as its size, timestamps, and permissions.
For getting the file size specifically, you can access the st_size
member of the struct stat
. This member represents the size of the file in bytes.
Code Example:
#include <sys/stat.h>
#include <iostream>
int main() {
const char *filename = "example.txt"; // Replace with your file path
struct stat fileStat;
if (stat(filename, &fileStat) == 0) {
// File size in bytes
std::cout << "File Size: " << fileStat.st_size << " bytes" << std::endl;
} else {
std::cerr << "Error getting file information." << std::endl;
}
return 0;
}
In this example, we start by including the necessary headers: <iostream>
for input and output operations and <sys/stat.h>
for the stat
function.
We then declare the main
function, where we define the filename of the file for which we want to get the size (example.txt
in this case). You should replace this with the actual path to your file.
Next, we declare a struct stat
variable named fileStat
. This structure will hold the information about the file.
Inside the main
function, we use the stat
function to retrieve information about the file specified by filename
.
If the function call is successful (returns 0), we print the file size in bytes using fileStat.st_size
. If there is an error, we print an error message to the standard error stream.
Code Output:
When you run the program, it will output the size of the specified file:
Replace example.txt
with the path to the file you want to examine, and the program will display the size of that file in bytes.
How to Get File Size in C++ Using Boost.Filesystem
In cases where your C++ codebase predates C++17, the std::filesystem
library might not be available. To address this, Boost.Filesystem
serves as a valuable alternative, offering filesystem operations in a pre-C++17 environment.
Boost.Filesystem
provides the file_size
function, which operates similarly to std::filesystem::file_size
. The syntax involves including the necessary headers from the Boost.Filesystem
library and calling boost::filesystem::file_size
with a boost::filesystem::path
argument.
The relevant code snippets are as follows:
#include <boost/filesystem.hpp>
#include <iostream>
uintmax_t boost::filesystem::file_size(const boost::filesystem::path& p);
Code Example:
#include <boost/filesystem.hpp>
#include <iostream>
int main() {
boost::filesystem::path filePath = "example.txt";
try {
uintmax_t fileSize = boost::filesystem::file_size(filePath);
std::cout << "The size of the file is: " << fileSize << " bytes"
<< std::endl;
} catch (const boost::filesystem::filesystem_error& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
In this example, we begin by including the necessary headers, specifically <iostream>
and <boost/filesystem.hpp>
. We declare a boost::filesystem::path
object named filePath
and initialize it with the path to the target file (example.txt
in this case).
Inside the try
block, we call boost::filesystem::file_size(filePath)
to retrieve the size of the file specified by filePath
.
The result is stored in the variable fileSize
, which is of type uintmax_t
. We use std::cout
to display the file size in bytes.
To handle potential exceptions, we use a try...catch
block. If an exception occurs (for instance, if the file does not exist), the catch
block captures the exception and prints an error message using std::cerr
.
Code Output:
Assuming the file example.txt
exists, the output of the program would be:
Replace example.txt
with the path to the file you want to examine, and the program will display the size of that file in bytes. If an error occurs, the program will output an error message detailing the nature of the issue.
Boost.Filesystem
provides a convenient and portable way to handle file system operations in C++ for projects that don’t have access to C++17 features.
How to Get File Size in C++ Using C Standard Library Functions
In scenarios where external libraries are not an option, we can leverage standard C library functions to obtain file size information. The combination of fopen
, fseek
, and ftell
allows for a straightforward approach to determining the size of a file.
The general approach involves opening the file using fopen
, moving the file pointer to the end with fseek
, determining the position with ftell
(which gives the file size), and finally closing the file with fclose
. Here’s a brief overview of the syntax:
#include <cstdio>
FILE* fopen(const char* filename, const char* mode);
int fseek(FILE* stream, long int offset, int origin);
long int ftell(FILE* stream);
int fclose(FILE* stream);
Code Example:
#include <cstdio>
#include <iostream>
int main() {
const char* filePath = "example.txt";
FILE* file = std::fopen(filePath, "rb");
if (file != nullptr) {
std::fseek(file, 0, SEEK_END);
long fileSize = std::ftell(file);
std::fclose(file);
if (fileSize != -1) {
std::cout << "File size of example.txt is: " << fileSize << " bytes"
<< std::endl;
} else {
std::cerr << "Error getting file size." << std::endl;
}
} else {
std::cerr << "Error opening file." << std::endl;
}
return 0;
}
In this example, we begin by including the necessary headers, including <cstdio>
for C standard I/O operations.
We declare the file path as a character array (const char* filePath
) and open the file using std::fopen(filePath, "rb")
. The second argument, rb
, specifies that we are opening the file in binary read mode.
Next, we use std::fseek
to move the file pointer to the end of the file (SEEK_END
). The file size is then obtained using std::ftell
.
If successful, the file is closed using std::fclose
. We check for errors at each step and output the file size if everything is in order.
Code Output:
Assuming the file example.txt
exists, the program output would be:
You can also replace example.txt
with the path to the file you want to examine, and the program will display the size of that file in bytes. If an error occurs, such as the file not existing or being inaccessible, the program will output an appropriate error message.
This approach provides a basic yet effective method for obtaining file size information using standard C library functions.
Conclusion
Knowing how to obtain file size in C++ is a fundamental skill for developers working with file manipulation and storage. Whether you’re coding in a modern C++17 environment, dealing with pre-C++17 codebases, or navigating different operating systems, there’s a method tailored to your needs.
The std::filesystem::file_size
function offers a concise and elegant solution for contemporary projects, while C standard library functions provide a robust, traditional approach. For those traversing POSIX-compliant systems, the stat
functions become invaluable.
Additionally, when working in pre-C++17 settings, Boost.Filesystem
is a reliable approach. By understanding and utilizing these diverse methods, you can confidently tackle file size retrieval in any coding scenario, enhancing the versatility and robustness of your applications.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook