C++ でファイルサイズを取得する
-
C++ で
std::filesystem::file_size
関数を使用してファイルサイズを取得する -
C++ で
std::filesystem::file_size
とstd::filesystem::path
を使用してファイルサイズを取得する -
C++ で
std::filesystem::file_size
とerror_code
を使用してファイルサイズを取得する -
C++ で
stat
関数を使用してファイルサイズを取得する
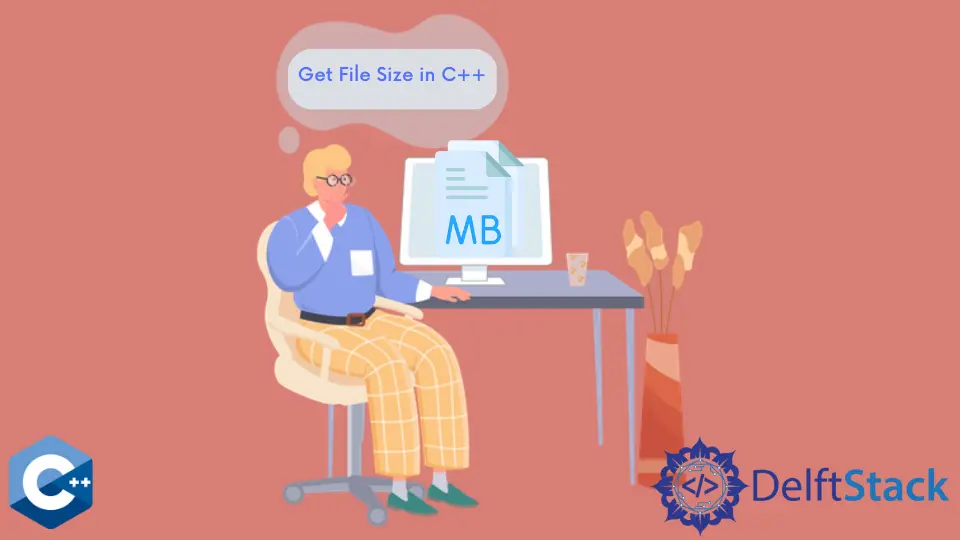
この記事では、C++ でファイルサイズを取得する方法の複数の方法を示します。
C++ で std::filesystem::file_size
関数を使用してファイルサイズを取得する
std::filesystem::file_size
はファイルのサイズをバイト単位で取得する C++ ファイルシステムライブラリ関数です。std::filesystem::file_size
はファイルのパスを関数の引数にとり、型は std::filesystem::path
です。これは std::filesystem::path
型のものであるが、以下の例ではファイルのパスの string
値を渡しています。
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
int main() {
string path = "input.txt";
cout << "size of file '" << path << "' = " << std::filesystem::file_size(path)
<< endl;
return EXIT_SUCCESS;
}
出力:
size of file 'inbox.txt' = 10440
C++ で std::filesystem::file_size
と std::filesystem::path
を使用してファイルサイズを取得する
関数 std::filesystem::file_size
の代替的な使い方は、ファイルパスの値を path
型変数に挿入することです。まず、型を使用する前に using std::filesystem::path
文を含める必要があります。次に、以下の例のように path
オブジェクトを宣言し、文字列リテラルで初期化します。構築した変数を file_size
関数に渡してファイルサイズを取得します。
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::error_code;
using std::ifstream;
using std::string;
using std::filesystem::path;
int main() {
class path fd_path = "input.txt";
cout << "size of file '" << fd_path
<< "' = " << std::filesystem::file_size(fd_path) << endl;
return EXIT_SUCCESS;
}
出力:
size of file 'inbox.txt' = 10440
C++ で std::filesystem::file_size
と error_code
を使用してファイルサイズを取得する
std::filesystem::file_size
はファイルのサイズを取得するために基盤となるオペレーティングシステムのサービスを利用しているので、失敗して例外が発生する可能性が高いです。各 file_size
関数呼び出しをより適切に処理するために、error_code
オブジェクトを第 2 引数に用い、失敗した場合のエラーメッセージを格納しておくことを推奨します。その結果、error_code
オブジェクトを評価し、if...else
文を用いてエラー処理コードを実装することができます。
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::error_code;
using std::ifstream;
using std::string;
using std::filesystem::path;
int main() {
string path = "input.txt";
error_code ec{};
auto size = std::filesystem::file_size(path, ec);
if (ec == error_code{})
cout << "size of file '" << path << "' = " << size << endl;
else
cout << "Error accessing file '" << path << "' message: " << ec.message()
<< endl;
return EXIT_SUCCESS;
}
出力:
size of file 'inbox.txt' = 10440
C++ で stat
関数を使用してファイルサイズを取得する
stat
は複数のオペレーティングシステムで利用できる POSIX 準拠の関数です。多くの場合、std::filesystem::file_size
メソッドの下にある std::filesystem::file_size
メソッドが使用している関数です。stat
は第 1 引数に文字列を取り、第 2 引数に struct stat
型のオブジェクトのファイルアドレスのパスを指定します。
struct stat
は定義済みの特別なオブジェクトであり、stat
関数呼び出しが成功した後に複数のファイルパラメータを格納します。ファイルサイズをバイト数で格納する struct stat
オブジェクトの st_size
データメンバにアクセスするだけでよいことに注意してください。
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
int main() {
string path = "input.txt";
struct stat sb {};
if (!stat(path.c_str(), &sb)) {
cout << "size of file '" << path << "' = " << sb.st_size << endl;
} else {
perror("stat");
}
return EXIT_SUCCESS;
}
出力:
size of file 'inbox.txt' = 10440