C++에서 파일 크기 가져 오기
-
std::filesystem::file_size
함수를 사용하여 C++에서 파일 크기 가져 오기 -
std::filesystem::file_size
를std::filesystem::path
와 함께 사용하여 C++에서 파일 크기를 가져옵니다 -
std::filesystem::file_size
를error_code
와 함께 사용하여 C++에서 파일 크기 가져 오기 -
stat
함수를 사용하여 C++에서 파일 크기 가져 오기
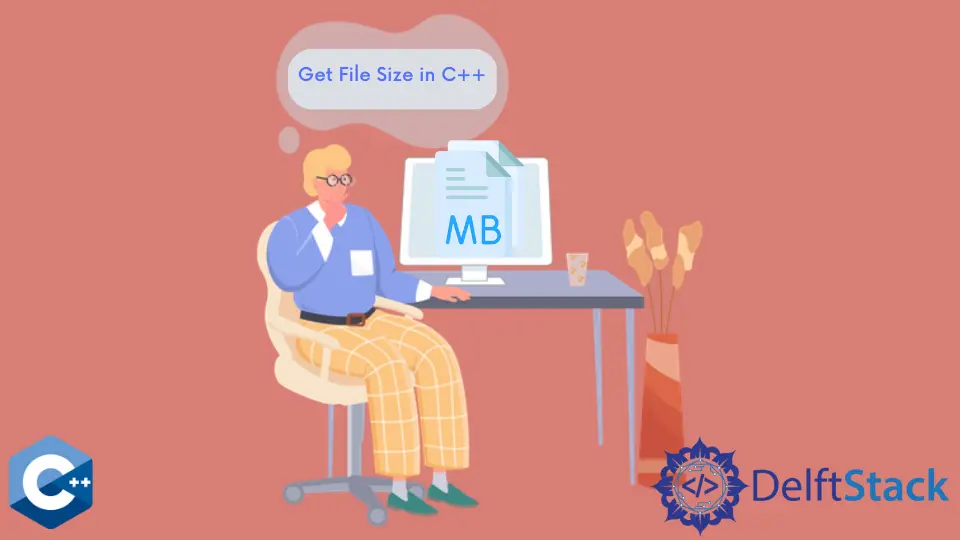
이 기사는 C++에서 파일 크기를 얻는 방법에 대한 여러 가지 방법을 보여줍니다.
std::filesystem::file_size
함수를 사용하여 C++에서 파일 크기 가져 오기
std::filesystem::file_size
는 파일 크기를 바이트 단위로 검색하는 C++ 파일 시스템 라이브러리 함수입니다. std::filesystem::file_size
는std::filesystem::path
유형의 함수 인수로 파일 경로를 사용합니다. 그러나 다음 예제에서는 파일 경로의string
값을 전달한 다음 해당 객체를 구성하고 파일 크기를 검색하는 데 사용됩니다.
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
int main() {
string path = "input.txt";
cout << "size of file '" << path << "' = " << std::filesystem::file_size(path)
<< endl;
return EXIT_SUCCESS;
}
출력:
size of file 'inbox.txt' = 10440
std::filesystem::file_size
를std::filesystem::path
와 함께 사용하여 C++에서 파일 크기를 가져옵니다
std::filesystem::file_size
함수의 또 다른 사용법은 파일 경로 값을path
유형 변수로 삽입하는 것입니다. 처음에는 유형을 사용하기 전에using std::filesystem::path
문을 포함해야합니다. 다음으로 다음 예제 코드와 같이path
객체를 선언하고 문자열 리터럴로 초기화 할 수 있습니다. 생성 된 변수는 파일 크기를 가져 오기 위해file_size
함수에 전달됩니다.
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::error_code;
using std::ifstream;
using std::string;
using std::filesystem::path;
int main() {
class path fd_path = "input.txt";
cout << "size of file '" << fd_path
<< "' = " << std::filesystem::file_size(fd_path) << endl;
return EXIT_SUCCESS;
}
출력:
size of file 'inbox.txt' = 10440
std::filesystem::file_size
를error_code
와 함께 사용하여 C++에서 파일 크기 가져 오기
std::filesystem::file_size
는 기본 운영 체제 서비스를 사용하여 파일 크기를 검색하므로 실패 할 가능성이 높고 예외가 발생할 수 있습니다. 각file_size
함수 호출을 더 잘 처리하려면error_code
객체를 두 번째 인수로 사용하고 실패시 오류 메시지를 저장하는 것이 좋습니다. 결과적으로error_code
객체를 평가하고if...else
문을 사용하여 오류 처리 코드를 구현할 수 있습니다.
#include <filesystem>
#include <fstream>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::error_code;
using std::ifstream;
using std::string;
using std::filesystem::path;
int main() {
string path = "input.txt";
error_code ec{};
auto size = std::filesystem::file_size(path, ec);
if (ec == error_code{})
cout << "size of file '" << path << "' = " << size << endl;
else
cout << "Error accessing file '" << path << "' message: " << ec.message()
<< endl;
return EXIT_SUCCESS;
}
출력:
size of file 'inbox.txt' = 10440
stat
함수를 사용하여 C++에서 파일 크기 가져 오기
stat
는 여러 운영 체제에서 사용할 수있는 POSIX 호환 함수입니다. 종종 std::filesystem::file_size
메서드가 내부적으로 사용하는 함수입니다. stat
는 문자열을 첫 번째 인자로 사용하여struct stat
유형 객체의 파일 주소 경로를 두 번째 인자로 나타냅니다.
struct stat
는 성공적인stat
함수 호출 후 여러 파일 매개 변수를 저장하는 사전 정의 된 특수 객체입니다. 파일 크기를 바이트 수로 저장하는struct stat
객체의st_size
데이터 멤버에만 액세스하면됩니다.
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
int main() {
string path = "input.txt";
struct stat sb {};
if (!stat(path.c_str(), &sb)) {
cout << "size of file '" << path << "' = " << sb.st_size << endl;
} else {
perror("stat");
}
return EXIT_SUCCESS;
}
출력:
size of file 'inbox.txt' = 10440
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook